[name|Friend] unclear state dependencies are the biggest source of code that's difficult to detangle.
So much so that Shared State Bad is a programming dogma described in books like Pragmatic Programmer and many others. But shared state is fine, even necessary, when done right. Let me explain.
One of my earliest big projects was a text-based GUI for DOS. I was 12 and called it an operating system because I didn't know any better. That project taught me an important lesson about shared state.
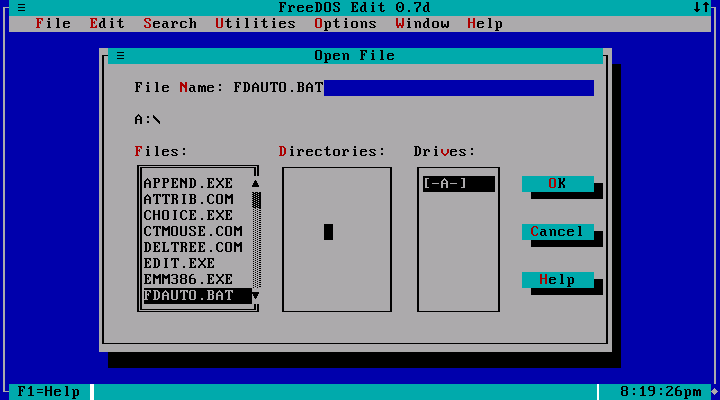
I wrote the project in Turbo Pascal before learning about functions, procedures, arrays, and objects. The whole codebase lived in 1 file, used nearly 200 global variables, and to give you a sense of scale: I ran into trouble because GOTO couldn't jump more than 4096 lines of code. Solved that with double jumps 🤘
All talent no skill.
Note: The GOTO statement lets you jump to pre-defined locations in the code. Once popular, the practice went away with the Structured Programming movement of the 60's and 70's and was replaced in most contexts with functions by the early 90's. You might still see GOTO in performance-critical low-level applications.
The word "mess" doesn't even begin to describe my big project. I used global variables named a
, b
, .. aa
, ab
sort of like a programmer in the 1960's might use absolute memory addressing. I even re-used variables between features, which meant that a variable's exact meaning depended on how you got there.
This caused many hard to fix bugs. Click through the UI in a new sequence of steps and everything gets jumbled up.
How shared state causes problems
The intermingled state made it so my code's exact behavior depended on how users (really just me) went through features. Here's a simplified example:
var counter: integer
counter := 0
add:
counter := counter + 1
writeln(counter)
sub:
counter := counter - 1
writeln(counter)
goto add
fun_feature1:
counter := counter + 10
goto sub
fun_feature2:
counter := 2
goto fun_feature1
add
: theadd
block adds +1 and prints the valuesub
: thesub
block subs -1, prints the value, and jumps toadd
fun_feature1
: thefun_feature1
block adds +10 and jumps tosub
fun_feature2
: thefun_feature2
block prints a message and jumps tofun_feature1
Note: DOS was limited to 25 lines. Turbo Pascal used 4 lines for the UI. Meaning my code spanned at least 195 screens. With all 200+ variables defined in a big block at the top of the file. 12 year old Swiz must've been a genius.
Unclear shared state happens quickly
You don't need GOTO programming to run into unclear shared state. Here's a typical situation that happens in event-based code:
let menuIsOpen = false;
function toggleMenu() {
menuIsOpen = !menuIsOpen;
window.localStorage.setItem("savedMenuState", menuIsOpen);
updateMenu();
}
function updateMenu() {
if (menuIsOpen) {
// display menu
} else {
// hide menu
}
}
document.onload = () => {
menuIsOpen = window.localStorage.getItem("savedMenuState");
updateMenu();
};
Prefer pure functions
The issue is that neither toggleMenu
nor updateMenu
are pure functions. You can't tell what they'll do unless you know the value of menuIsOpen
.
Note: A pure function is one that relies only on its arguments and has no side-effects. You know it's pure when calling the same function multiple times with unchanged arguments produces the same result.
When shared state works great
Shared state isn't all doom and gloom. It causes problems when you have asynchronous or threaded code and unclear access patterns.
But your database is a repository of shared state and that works great. The cache in your networking layer is a type of shared state. Works fine. State management libraries popular in modern app development are all about sharing state and they can be fantastic.
What gives?
Explicitly declared state dependencies with strict guidelines around access patterns make all the difference. If a compiler or linter can enforce those patterns, even better.
function SomeBeautifulData({ id }) {
const { data } = useQuery(
['beautiful-data', id],
...
)
return data.map( ... )
}
Thanks to explicit state dependencies, you can let framework machinery handle the details 😍
Cheers,
~Swizec
PS: databases achieve this with ACID – atomicity, consistency, isolation, durability. By ensuring every write completes before any read and that writes can't conflict, a database can ensure you always read the right data.
Continue reading about Avoid spooky action at a distance
Semantically similar articles hand-picked by GPT-4
- Do you really need immutable data?
- Wormhole state management
- How to think of your business logic as data
- When your brain is breaking, try Stately.ai
- A new VSCode extension makes state machines shine on a team
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️