What makes code hard to read and think about? A combinatorial explosion of states and execution paths.
This happens when you put everything inside your component. Data loading, transformations, error handling, loading states, and that's before you even think about user interactions.
Need to coordinate 2 or more data loaders? Fuggedaboutit.
if ((loading1 || loading2) && !error1 || ...)
The number of bugs I've caused this way is too damn high 🫠
Code like this leads to high cyclomatic complexity, which is less problematic than architectural complexity, but does make smoke come out your ears. The code is brittle and likely to break any time you sneeze wrong.
Suspense to the rescue
Ever since using TanStack Router, where these patterns are built-in at the router level, I'm increasingly a fan of solving these problems with React Suspense.
https://x.com/Swizec/status/1792051188432839105
The code shows a piece of UI that renders when showBirthdays = true
. Like when you click a button to start loading.
Suspense
handles the loading state. ErrorBoundary
handles the error state.
You can try it out here and see full code on GitHub.
Main component – happy path
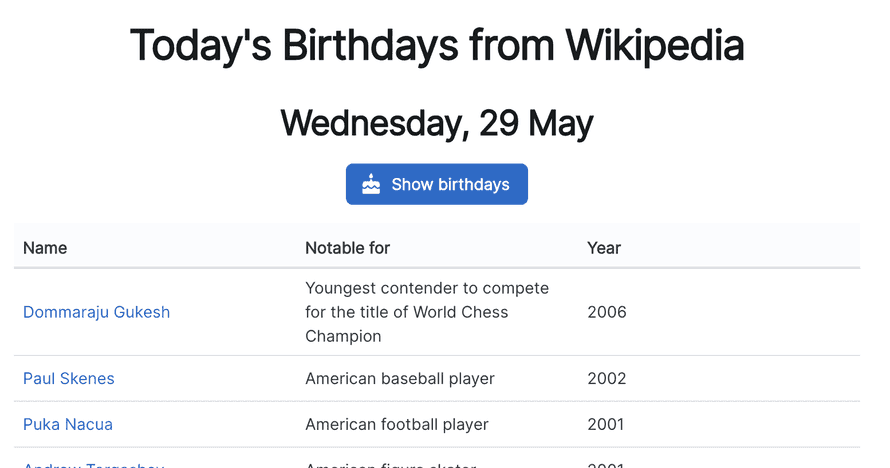
That leaves your component to implement the happy path.
const Birthdays: FC<{ day: Date }> = ({ day }) => {
const births = useBirths(day)
return <Table data-testid="birthday-list">// ...</Table>
}
No loading states. No error handling. Just a data loader and rendering. You could add more data loaders and assume everything's perfect in the rendering portion of your component.
Data loader – leverages suspense
The data loader leverages suspense to suspend rendering while data loads. I'm using useSuspenseQuery
from React Query, but similar tools exist in other popular libraries.
function useBirths(day: Date) {
const query = useSuspenseQuery<BirthEntry[]>({
queryKey: ["wikipedia-birthdays", day],
queryFn: async () => {
const response = await fetch(
`https://en.wikipedia.org/api/rest_v1/feed/onthisday/births/${
day.getMonth() + 1
}/${day.getDate()}`
)
if (!response.ok) {
throw new Error("Error fetching births")
}
const { births } = (await response.json()) as {
births: BirthEntry[]
}
births.sort((a, b) => b.year - a.year)
return births
},
})
return query.data
}
Notice that I can just throw an Error when it happens. This is important. You want any error during processing or rendering to throw so that the ErrorBoundary
can catch it and show feedback to the user.
Another important detail is that I'm sorting and parsing data directly in the loader. That improves your render performance because you're caching the data you use, not just the data you fetch.
https://x.com/Swizec/status/1794136049276669977
Error component – for the errors
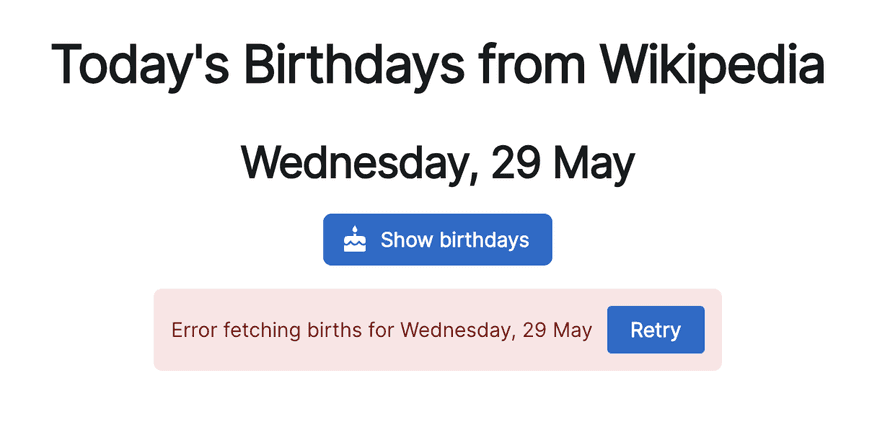
The ErrorBoundary
renders an error component when something's wrong. This component lets users recover from an error by resetting the boundary and refetching the data.
const LoadError: FC<{ day: Date }> = ({ day }) => {
const queryClient = useQueryClient()
const { resetBoundary } = useErrorBoundary()
function refetch() {
resetBoundary()
queryClient.refetchQueries({
queryKey: ["wikipedia-birthdays", day],
})
}
return (
<Alert color="danger">
Error fetching births for {getPrettyDate(day)}{" "}
<Button onClick={refetch}>Retry</Button>
</Alert>
)
}
Powered by the popular react-error-boundary component from B Vaughn, a former member of the React team.
Loading component – for loading states
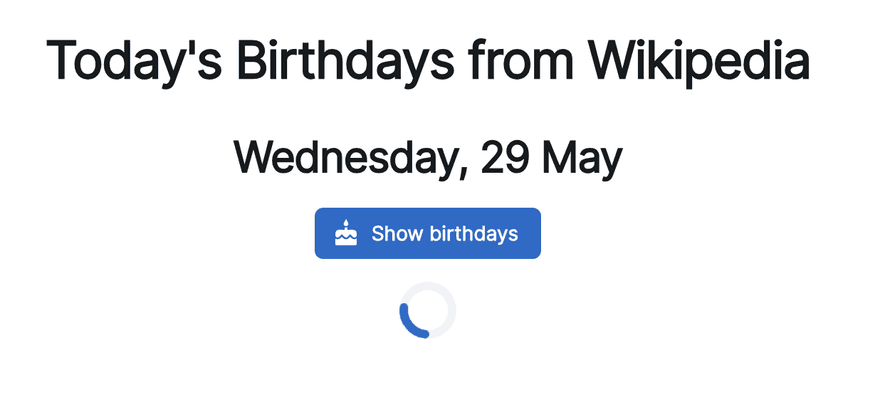
The loading component in my case is just a <CircularProgress>
spinner. You can make it as fancy as you want.
Why this helps
No more god components that do everything! No more god hooks that try to coordinate a bazillion different states.
Keep your happy path, loading state, and errors separate. Think about one at a time ✌️
~Swizec
Followup answer to Wtf why does this work? 👉 Why useSuspenseQuery works?
Continue reading about Cleaner components with useSuspenseQuery
Semantically similar articles hand-picked by GPT-4
- Why useSuspenseQuery works
- React 18 and the future of async data
- Towards a Gatsby+Suspense proof-of-concept
- Async React with NextJS 13
- A new VSCode extension makes state machines shine on a team
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️