You know how a pen works, right? A nice ballpoint pen that you use every day.
Now explain how it works. 5 minutes. Write down how a pen works in as much detail as you can muster.
No worries if you can't. Neither could participants in a 2002 study into The Illusion of Explanatory Depth.
We think we understand problems until we try to solve them. Then all sorts of nasty little details crop up. Tiny gotchas you never could've guessed.
Say you're writing a loop that calculates times in 7 day intervals. You might write something like this:
function getTimes(startAt, N) {
let times = [];
for (let i = 0; i < N; i++) {
times.push(addDays(startAt, 7 * i));
}
return times;
}
Add multiples of 7 days to the start time until there's N
timestamps in your array. Not the prettiest code in the world, but it works and it's easy to read.
You find more depth
The code has a problem. Works great for UTC because there's no daylight savings. +7 days is +7 days no matter when.
Translate those UTC timestamps into human time and they shift around by 1 hour depending on season. Depending on where in the world you are.
For users in USA, except Arizona, the shift happens on March 13th and November 6th. In Europe it happens on March 27th and October 30th.
To support human time, your code needs to become timezone aware. Like this:
function getTimes(startAt, N, timezone) {
let times = [];
const zonedStartAt = utcToZonedTime(startAt, timezone);
for (let i = 0; i < N; i++) {
times.push(zonedTimeToUtc(addDays(zonedStartAt, 7 * i), timezone));
}
return times;
}
The code converts startAt
from a UTC timestamp to the right time in our target timezone. Then it adds multiples of +7 days in a loop while converting from zoned time to UTC time.
utcToZonedTime
and zonedTimeToUtc
use the IANA Timezone Database to lookup the correct time conversions for any date. When the database changes in the future, we'll need to upgrade the date-fns-tz
library.
The rabbit hole goes deep, always
But that still doesn't work for all dates.
The transition from Julian to Gregorian calendar in 1582 removed 15 days of that year. Few date-time libraries and execution environments support that change correctly.
You can't run this code on a satellite either. For that you'd need to take relativistic effects into account. Especially, if you need millisecond precision.
Let's not even get into leap seconds and how UTC is measured as the average between multiple atomic clocks spread around the world.
Rabbit holes are common in programming because we translate fuzzy understanding into exacting specifications.
Like what is the best way to store a person's name? Or a billing address? Or map data?
Popular misconceptions are common enough that Falsehoods programmers believe about X emerged as a genre of internet writing. The real world is messy.
Even the invented world is messy
Even something as well-defined as an email address can be full of hidden complexity. Check out this state machine that validates an email address
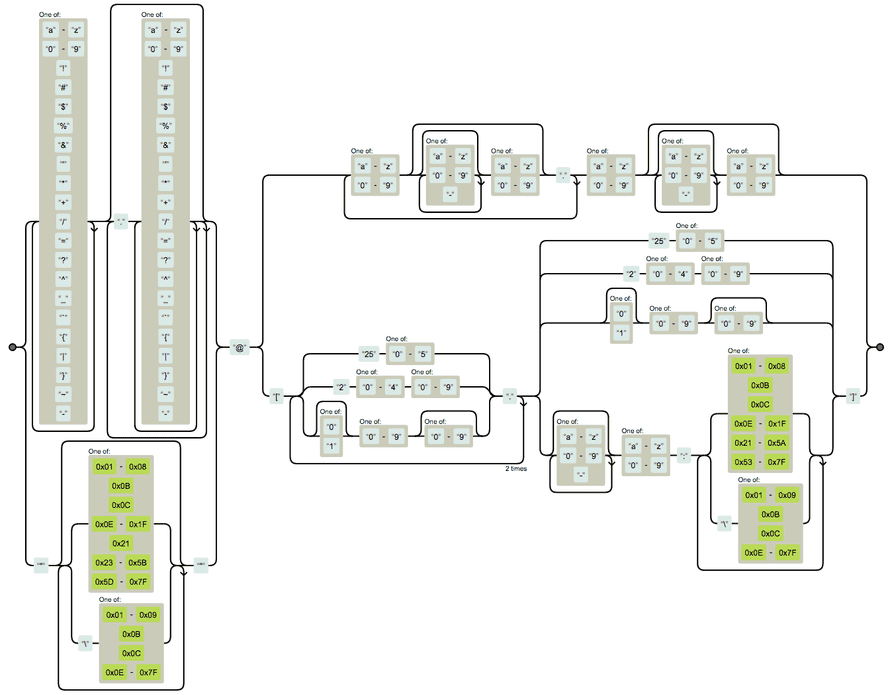
Yeah, I never would've guessed it either.
Next time you look at convoluted code and think "wow terrible, needs a rewrite", take a moment to consider you're missing some nuance.
Or you've learned the nuance and see that the code looks wrong. Sometimes you code around a problem in circles. The insight of understanding simplifies it all.
Cheers,
~Swizec
Continue reading about Coding forces you to understand the problem
Semantically similar articles hand-picked by GPT-4
- Saving time in UTC doesn't work and offsets aren't enough
- A day is not 60*60*24 seconds long
- Words that scare developers
- Loops are the hardest
- It takes about two months to write a technical book
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️