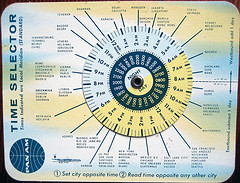
A couple of days ago I started refactoring some code to handle timezones better. The problem manifests as a single time appearing at different times in different parts of the application.
Granted, a lot of it is more of a UX problem along the lines of my rant on why computers don't handle timezones intuitively. But there's a deeper issue with the fact that the codebase is handling timezones rather naively.
Until a week ago I would have done it exactly the same way.
Naive approach
Usually when people first implement timing in an application they (or rather we) don't really care about timezones. You just take a timestamp and store it in a database. This suffices for most use cases ... sure the times are in whatever timezone your server is set to but as long as you're using the same server for everything and you're only using times for timestamps when objects were inserted into the database and for logging ...
It's just not a problem.
Then you are faced with a distributed environment and suddenly all those timestamps start acting funny, or maybe you have users in different timezones and you want to display times to them.
Still an easy problem to solve; just handle all times as UTC internally and translate to the proper timezone when producing outputs. Easy as pie right?
And then you notice strange things happen around daylight savings. Suddenly 3am happens twice on the 31st of October in Slovenia ... but the same thing only happens a week later in the US. And some states in the US don't even observe DST and now what do you do?
pyTZ
Those ambiguous periods are the main thing pytz takes care of.
To be perfectly honest I never even considered this problem before reading the documentation and having my mind blown. There's just so much intricacies over keeping up to date on what each timezone is doing and what particular timezone a user is in right now ... it's insane!
Even though pytz claims they don't take care of ambiguous periods like Poland rewinding their clocks half an hour in 1915 to start using CET, they're still pretty vital for everything else.
Of course you'll still want to handle all times internally as UTC otherwise doing time arithmetic can get quite hairy, although pytz can supposedly handle that too ... but at least now it should be possible to correctly get the UTC time out of user input and then correctly display the result.
An example:
from datetime import datetime
from pytz import *
eastern = timezone('US/Eastern')
loc_dt = eastern.localize(datetime(2011, 11, 2, 7, 27, 0))
print loc_dt
# 2011-11-02 07:27:00-04:00
ljubljana = timezone("Europe/Ljubljana")
print loc_dt.astimezone(ljubljana)
# 2011-11-02 12:27:00+01:00
Conclusion
Turns out there's much more to timezones than one might think. Just goes to show why all those other coders are spending so much time on trivial stuff ... nothing is really trivial. Nothing.
And let's not even get into leap seconds.
Continue reading about Handling timezones in python
Semantically similar articles hand-picked by GPT-4
- Why don't computers understand timezones?
- Saving time in UTC doesn't work and offsets aren't enough
- A day is not 60*60*24 seconds long
- Words that scare developers
- I kicked myself in the balls
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️