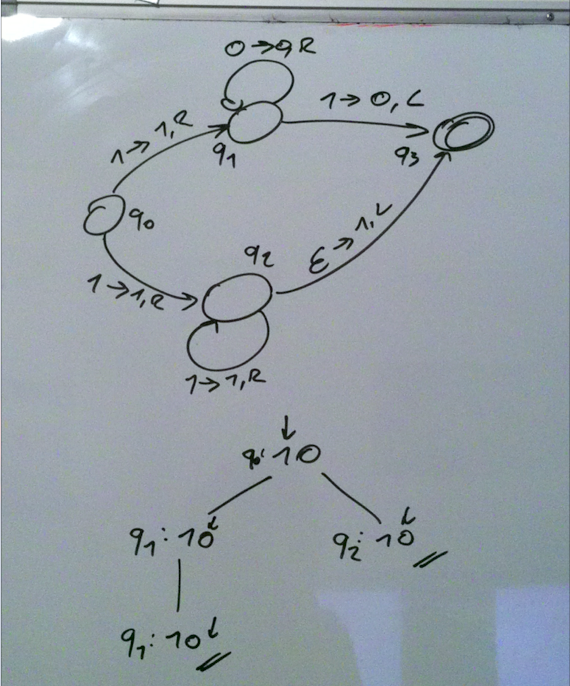
Felt like doing some coding last night and since my friends were just trolling me about codegolfing a turing machine simulator(they need it for a term paper), I decided to do just that.
At first I wanted to make it super simple, 10 lines of code max ... and I failed.
What better way to embrace failure than to implement a simulator of nondeterministic turing machines in as little lines of code as possible?
Adding "eps" to the alphabet of course - basically a means for the machine to know when it's gone off either end of the tape and that it can also write nothing to the tape. Both very useful features!
And here's the code in all its glory. You can also view it on github if that's your thing.
// transition format: {from: 'q1', to: 'q2', via: 'A', put: 'B', move: 1/-1}
// spits out whether end state was reached
// basic way to run: node turing.js machine.json 101 q4
// node turing.js
var _ = require("underscore"),
states = {};
var delta = function (states, step, end) {
if (_.keys(step).indexOf(end) >= 0) return true;
var _step = {},
foo = _.keys(step).map(function (k) {
var i = step[k][0],
t = step[k][1],
cur = i < 0 || i >= t.length ? states[k]["esp"] : states[k][t[i]];
if (cur)
cur.map(function (cur) {
if (cur.put != "eps") t.splice(i, 1, cur.put);
_step[cur.to] = [i + cur.move, t];
});
});
return _.size(_step) ? delta(states, _step, end) : false;
};
JSON.parse(require("fs").readFileSync(process.argv[2], "utf-8")).map(function (
i
) {
states[i.from] = states[i.from] || {};
states[i.from][i.via] = states[i.from][i.via] || [];
states[i.from][i.via].push(i);
});
console.log(
delta(states, { q0: [0, process.argv[3].split("")] }, process.argv[4])
);
Basically what you're doing is performing a breadth-first-search on a graph, looking for the end state and a pinch of complications with observing contents the tape as well. You should make sure not to accidentally implement a depth-first search since some branches might never terminate.
The above implementation could probably be codegolfed some more, but I don't like codegolfing by just compating lines beyond what is reasonable. I much prefer doing it with languages features and perhaps algorithmic improvements.
One of the biggest hindrances to making this much shorter is that a lot of operations on javascript arrays affect state, but don't return the new object as well. Lost at least three lines because of this!
An approach worth trying would be to perform a reduce on the tree of states and if the start and end state ever overlap, you know to return a truthy value, otherwise you go with something falsy. But I wasn't sure how to go about that.
Continue reading about Nondeterministic turing machine simulator in 23 lines of JavaScript
Semantically similar articles hand-picked by GPT-4
- Fun javascript feature
- A turing machine in 133 bytes of javascript
- Strangest line of python you have ever seen
- When your brain is breaking, try Stately.ai
- A new VSCode extension makes state machines shine on a team
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️