You probably remember spirographs as kid's toys from your youth. I had a simple set that was just a collection of plastic sprockets with holes for pencils.
Endless amounts of fun when I was two or three years old. I think ... I don't really remember much from that time, but I remember having those thingies and loving playing with them. One of my earliest memories even!
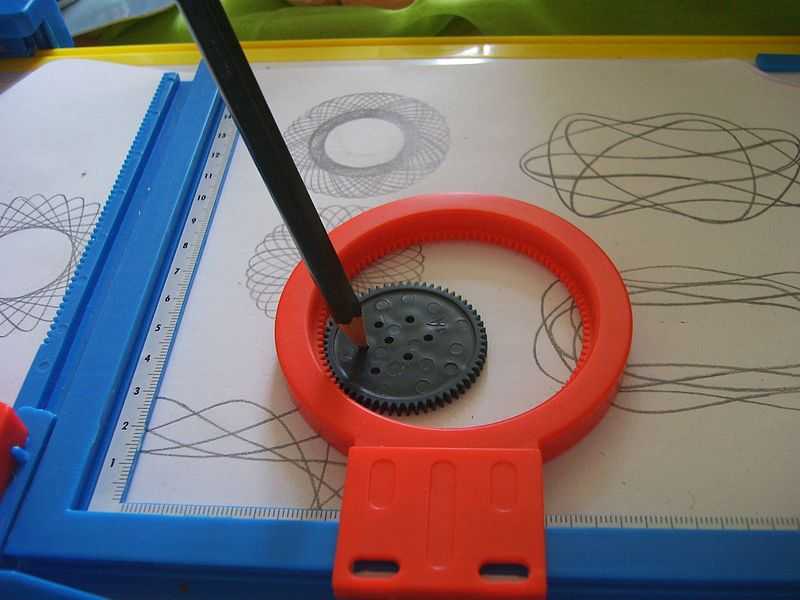
Last night I was making an animation example for the d3.js book I'm writing and ended up with the idea of progressively drawing out cool looking parametric equations. Little did I know, those are actually spirographs! Learned something while I was learning something.
<tweet gone missing>
My failure in common knowledge aside, it's possible to animate the drawing of parametric equations with just a few lines of d3.js code. What I'm about to show you has a pretty big problem, but produces cool looking results "in the lab". Try to guess what the problem is.
Animating spirographs
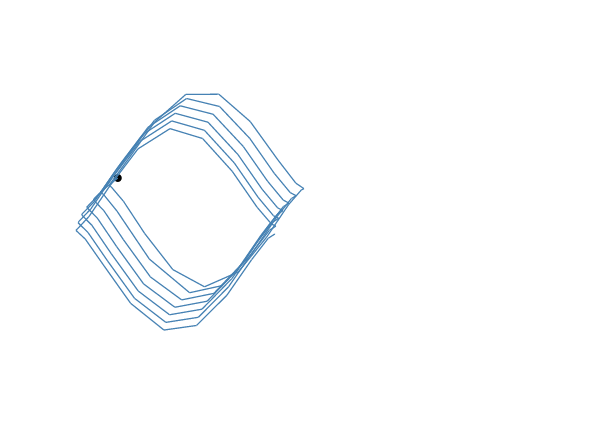
We'll make an animation timer and for each tick we'll draw a new step of the spirograph. After the spirograph is finished, we'll stop the timer. To make the animation more interesting to watch, we'll also fake a brush flying around (just a black dot).
We start with some basic html:
<title></title>
<link href="../bootstrap/css/bootstrap.min.css" rel="stylesheet" />
<div id="graph"></div>
<script src="http://d3js.org/d3.v3.min.js"></script>
<script src="timers.js"></script>
Then we hop into the javascript to flesh out the actual code.
var width = 600,
height = 600,
svg = d3
.select("#graph")
.append("svg")
.attr({ width: width, height: height });
I found the parametric function in the wikipedia's article on parametric equations. We'll be giving it a simple parameter calculated from the animation timer and it will return a two dimensional position.
var position = function (t) {
var a = 80,
b = 1,
c = 1,
d = 80;
return {
x: Math.cos(a * t) - Math.pow(Math.cos(b * t), 3),
y: Math.sin(c * t) - Math.pow(Math.sin(d * t), 3),
};
};
Tweaking the a, b, c, and d parameters will change the final shape.
Next we're going to define some scales to help us translate between maths space and our drawing space.
var t_scale = d3.scale
.linear()
.domain([500, 30000])
.range([0, 2 * Math.PI]),
x = d3.scale
.linear()
.domain([-2, 2])
.range([100, width - 100]),
y = d3.scale
.linear()
.domain([-2, 2])
.range([height - 100, 100]);
var brush = svg.append("circle").attr({ r: 4 }),
previous = position(0);
The t_scale is going to translate time into a parameter, x and y calculate proper positions on the final image using the coordinates returned by the position function.
We also put a simple circle on the image - this will represent the brush - and we need to take note of the previous position so we can draw lines between our current and previous state.
var step = function (time) {
if (time > t_scale.domain()[1]) {
return true;
}
var t = t_scale(time),
pos = position(t);
brush.attr({ cx: x(pos.x), cy: y(pos.y) });
svg.append("line").attr({
x1: x(previous.x),
y1: y(previous.y),
x2: x(pos.x),
y2: y(pos.y),
stroke: "steelblue",
"stroke-width": 1.3,
});
previous = pos;
};
This is our step function. It draws every consecutive step of the animation by moving the brush and putting a line between the current and previous position. The animation will stop when this function returns true so we make sure the time parameter doesn't go beyond t_scales's domain.
Finally, we simply start the timer.
var timer = d3.timer(step, 500);
The timer will start running after 500 milliseconds and repeatedly call the step _function until it returns _true.
You can check the animation out via the magic of github pages. The final spirograph looks like this:
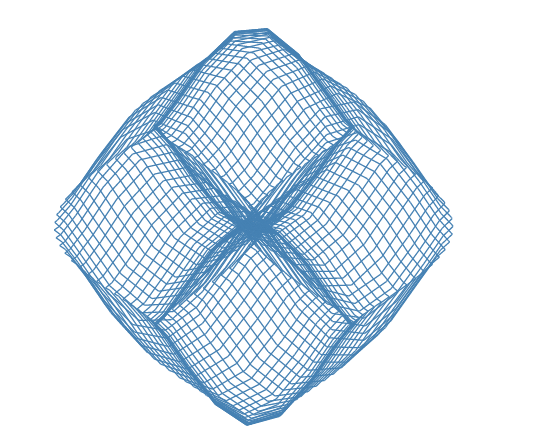
Figured out the problem yet?
The problem with this approach is that I'm using the animation timer itself as a parameter to the function, which means point density depends on how long you're willing to let the animation run. It will always draw the complete function because of how d3 scales work, but it might look very approximate. Think squares for circles approximate.
Another problem is that using a slower computer, or doing anything that lags the CPU even a little bit while the animation is running will ruin the final picture.
For instance, this is what happens when I switch desktops around while the browser is drawing.
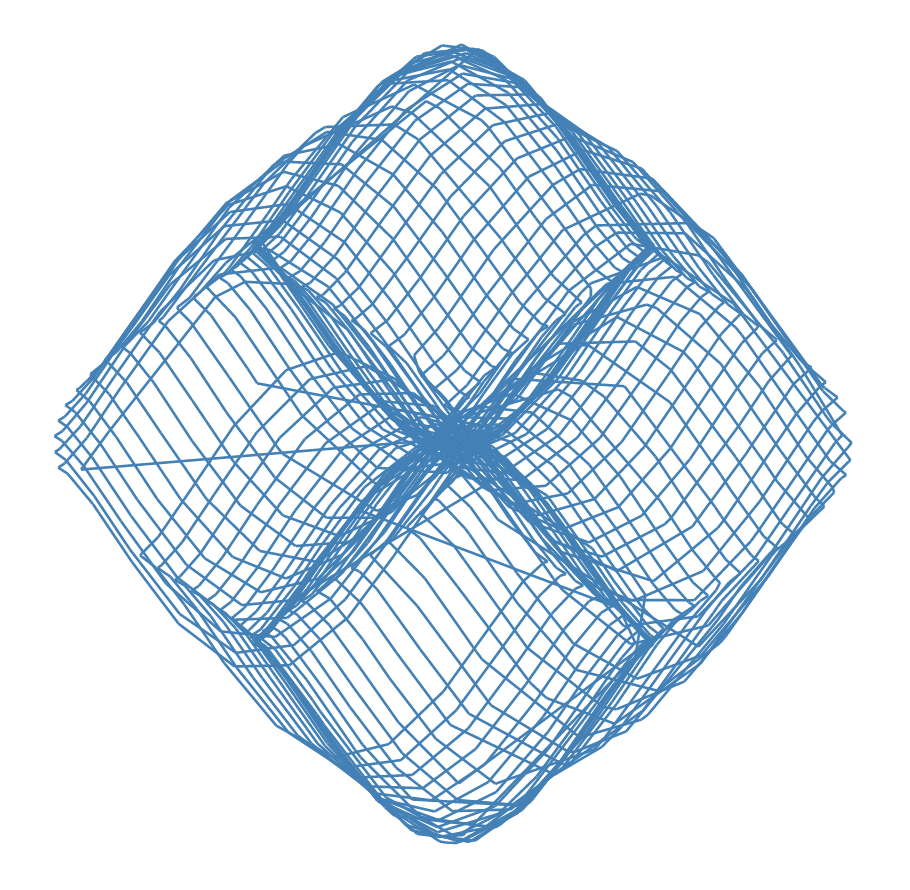
Continue reading about Sexy animated spirographs in 35 sloc of d3.js
Semantically similar articles hand-picked by GPT-4
- Quick scatterplot tutorial for d3.js
- (ab)Using d3.js to make a Pong game
- A Dancing Rainbow Snake – An Example of Minimal React and D3v4 transitions
- Flotr2 - my favorite javascript graph library
- JavaScript’s most popular dataviz library
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️