I'm trying something new. Today the video is stronger than the post. Watch it ?
On Tuesday, I implemented a JavaScript queue backed by local storage. It's goal in life is to ensure we don't lose events when tracking usage funnels on our app.
Yes, losing an event that takes 600ms to send is an edge case.
Yes, we still want to make sure we don't lose anything.
Plus, with a queue we have more flexibility to send bursts of events to our backend. Firing an XHR request every time a user clicks a button feels excessive.
That's why it's going in a queue, and the queue will eventually process it. At first, 1 event is 1 request, and we have a good path forward to send in batches.
Because we store the queue in local storage, you don't lose events even if you close your tab before something finishes saving. Hopefully you come back a second time so we get a chance to process your queue. If you don't, we can't. ?
Simple, right?
Yep. Stringify a JSON array. Save to local storage. Pull out, JSON parse, add stuff, put back in.
And what if the same user runs multiple tabs? What if some events that you want to track happen without user interaction? And just like that, tabs step on each other's toes, and you lose events because local storage is a shared resource.
Fast mutex to the rescue!
Here's how the Lamport lock algorithm works:
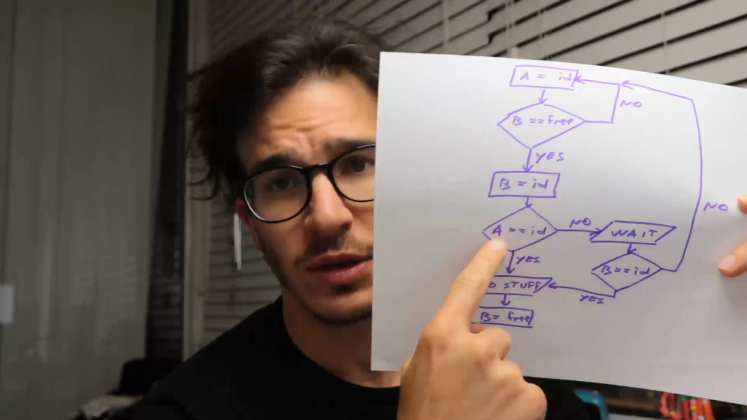
You need 2 shared memory locations, A
and B
.
When a new thread needs the lock, it first sets A
to its id
. Then it checks if B
is free. If B
is not free, we retry. If B
is free, we set B
to id
. Then we check that A
is still equal to id
.
If A
has changed, we're potentially facing a lock contention. So we wait long enough for others to realize we're doing stuff and back off. Then we check that B
is still id
. If B
is not equal to id
, then we've lost the lock and we go back to beginning. If B
is equal to id
, it means we've won the lock and we do stuff.
It sounds confusing because it is confusing. Study the flowchart. Drawing it helped me understand how this works. And I'm not sure I could prove that it works.
Here's what the Lamport lock looks like when implemented with JavaScript promises.
get lock() {
return this._lock(0);
}
_lock(retries) {
const A = `${this.name}_lock_A`,
B = `${this.name}_lock_B`;
const _retry = (resolve, reject) => {
window.requestAnimationFrame(() =>
this._lock(retries + 1).then(resolve, reject)
);
}
const _free = (name) => {
const val = this._getItem(name);
return Number(val) === 0 || val === undefined || val === null;
}
this._setItem(A, this.id);
return new Promise((resolve, reject) => {
// retries > 10, force lock
if (_free(B) || retries > 10) {
this._setItem(B, this.id);
if (this._getItem(A) === this.id) {
// no contention, do stuff
resolve();
this._setItem(B, 0);
}else{
// possible contention
window.requestAnimationFrame(() => {
if (this._getItem(B) === this.id) {
// won lock, do stuff
resolve();
this._setItem(B, 0);
}else{
_retry(resolve, reject);
}
});
}
}else{
_retry(resolve, reject);
}
});
}
Done with promises so it's easier to use. You'd do something like this when writing to local storage:
this.lock.then(() => writeStuff());
That's elegant, right? I think it is.
But what does all that lock code do? Well, it implements the Lamport lock algorithm with one small addition.
Our threads – browser tabs – can die before releasing the lock. The user closes the tab, JavaScript error, things like that. That's why we limit retries to <10
.
Yes, that's an arbitrary value. No, saving to local storage should never take more than 10 requestAnimationFrames. It's a fast, synchronous operation.
Look at the code and the flowchart side-by-side. I made sure they're the same ?
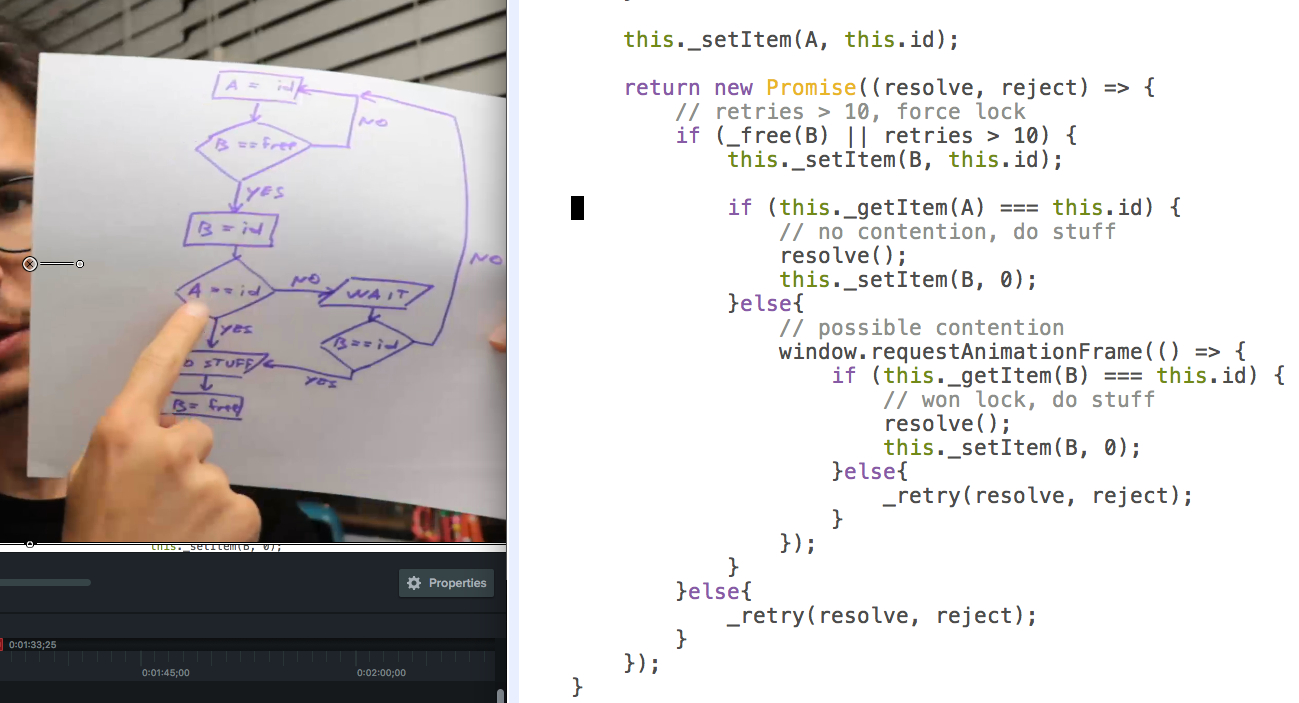
Don't believe me that it works? Read Lamport's original paper; it includes correctness proofs.
Watch the video to see it in action.
Thanks to Dan Pupius for the idea.
Continue reading about A Fast Mutex Lamport Lock with JavaScript Promises
Semantically similar articles hand-picked by GPT-4
- Logging 1,721,410 events per day with Postgres, Rails, Heroku, and a bit of JavaScript
- Livecoding recap: Blockchain-redux now shares blocks between clients in real-time
- Building an interactive DOM benchmark, preliminary results
- The commonest javascript bug
- Waiting for Godot with callbacks, promises, and async
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️