This week I tried a new stack for building webapps and found it pretty great. This isn't like a tutorial or anything, just a few thoughts and some code.
What's the most annoying part of building a webapp? The server-client separation.
Your application runs on different computers and now you have to deal with that split. Data and core business logic tends to live on the server. UI and light logic live on the client.
To get them talking, you need an API layer. This is where shit hits the fan.
Common types of APIs
REST is the gold standard with well-known advantages and drawbacks. The biggest issue is that APIs tend to balloon in size and are often built for 1 specific use-case in 1 specific client.
GraphQL made a good run at being the new gold standard, but never quite caught on. Great for complex reads, clunky for writes. Easy to write database-killing queries.
RPC โ remote procedure call โ is more of a vibe than a technology. You call an API with a specific function/task in mind. Many if not most REST and GraphQL APIs that I've seen are secretly RPC in shape.
You are almost guaranteed to build an RPC-shaped API, if you're building 1 specific server for 1 specific client. Sometimes RPC-over-REST is even the right choice! Like when a bunch of business logic needs to happen from a user action.
What's tRPC
tRPC is a ... framework? ... for building end-to-end type safe RPC APIs.
That means you get full type support with autocomplete on the server and the client. And runtime validation that your inputs and outputs match your API spec ๐
Best part: Without manually specifying or copying types in a bunch of places. TypeScript infers almost everything.
What's tRPC like to use
Here's example code from a project I'm building for AI Summit. We're trying to match conference attendees based on interests and suggest they meet. Excited to see if this works ๐ค
I rolled into an existing project and can't give you the full tutorial. Just the parts I know about โย adding an endpoint and calling it from React.
Creating an endpoint
To create endpoints you make a new tRPC router:
export const matchingRouter = createTRPCRouter({});
You define your endpoints as keys in that object. Each endpoint is a resolver function that gets an input and returns a response.
getMatches: publicProcedure
.input(z.string())
.query(async ({ input, ctx }) => {
const matches = // read data from a database or whatever
// it's just TypeScript, you can do whatever
return matches;
}),
Here I'm using zod to specify my input type and perform run-time validation. The tRPC + Zod integration is nice enough that VSCode knows that the input
argument is a string ๐
You can specify complex shapes and VSCode flawlessly picks up on that.
Fetching your endpoint
To fetch your endpoints, you'll need a tRPC client. In this case optimized for Next.js.
export const api = createTRPCNext<AppRouter>({
config() {
return {
// ...
};
},
ssr: false,
});
You then use queries same as you would with React Query. I think tRPC even uses React Query under the hood.
api.matching.getMatches.useQuery("clnb896di0002c949ugw700yc", {
onSuccess: (data) => {
console.log("hai", data);
},
});
tRPC gives you autocomplete for your API structure and the query ensures your component re-renders when new data becomes available. Does all the re-fetching and caching for you too.
What does an API call look like?
API calls are based on HTTP because we're on the web. No surprises here.
It does look like tRPC batches your API calls to reduce network traffic. That's neat.
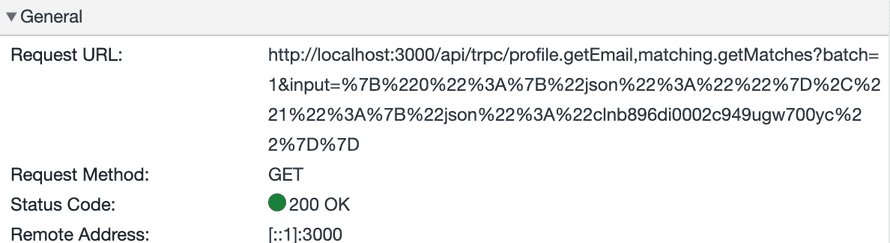

Ideally you'd never care about any of this. tRPC hides the details and makes your code work.
Good for writes?
Yep! tRPC writes don't feel clunky at all.
You define the router as .mutation
instead of query and make calls with useMutation
instead of query. Everything else works the same.
tRPC good or bad?
I liked it!
Super productive to work with, easy to jump into. Not sure I'd use it for a large project with a deep backend or when you have a bunch of clients that need to read the same data but in different shapes.
Feels like tRPC works best when you use the backend-for-frontend pattern. But I love that there's no fiddling with API definitions or any of that stuff.
Cheers,
~Swizec
Continue reading about A few thoughts on tRPC
Semantically similar articles hand-picked by GPT-4
- Better tooling won't fix your API
- REST API best practice in a GraphQL world
- TanStack Router โ modern React for the rest of us
- How you can start using GraphQL today without changing the backend
- Is hot dog taco?
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below ๐
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails ๐ on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. ๐"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help ๐ swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers ๐ ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are โค๏ธ