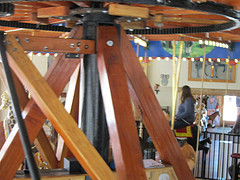
For loops are kind of a big deal.
So big in fact that it's virtually impossible to code any sizeable chunk of code without running into some sort of for loop ... sure, there's such awesome things as pure functional languages where for loops don't look like for loops. But I'm fairly certain most of those eventually turn into some sort of for loop deep down in the bowels of assembler and machine code.
It is therefore fairly easy to assert that everyone needs to know for loops like the back of their hand. But how well do you really know them?
A few days ago I ran into a silly thing with a couple of friends. We somehow got reminded that C counts the head of a for loop as part of the loop's scope, which means variables defined therein don't really affect anyone outside the loop. But at the same time, it seems like many (most?) other languages don't behave like this.
It seemed only reasonable to go write a for loop in many languages and see what happens.
C
#include<stdio class="h">
int main() {
int i = 5;
for (int i=0; i<2; i++) {
printf("%d\n", i); // prints 0, 1
}
printf("%d\n", i); // prints 5
}
</stdio>
PHP
<?
$i = 5;
for ($i=0; $i<2; $i++) {
echo "for:$i\n"; // prints 0, 1
}
echo "after:$i\n"; // prints 2
$i = 5;
for ($j=0; $j<2; $j++) {
$i = $j+1;
echo "for:$i\n"; // prints 1, 2
}
echo "after:$i\n"; // prints 2
?>
Java
// does not compile
public class For {
public static void main(String[] args) {
int i = 5;
for (int i; i<2; i++) {
System.out.println("for:"+i);
}
System.out.println("after:"+i);
}
}
Scala
object forVarReuse extends App {
var i=5;
for (i<-1 to 2) {
println("for:"+i); // prints 1, 2
}
println("after:"+i); // prints 5
}
Perl
$i = 5;
for (local $i=0; $i<2; $i++) {
print "for:$i\n"; # prints 0, 1
}
print "after:$i\n"; # prints 2
$i = 5;
for ($j=0; $j<2; $j++) {
local $i = $j+1;
print "for2:$i\n"; # prints 1, 2
}
print "after:$i\n"; # prints 5
Ruby
i = 5
for $i in 1..2
print "for:", $i, "\n" # prints 1, 2
end
print "after:", i, "\n" # prints 5
JavaScript
var i = 5
for (var i = 0; i < 2; i++) {
console.log("for:" + i) // prints 0, 1
}
console.log("after:" + i) // prints 2
i = 5
for (var j = 0; j < 2; j++) {
var i = j + 1
console.log("for2:" + i) // prints 1, 2
}
console.log("after:" + i) // prints 2
CoffeeScript
i = 5
console.log i for i in [0,1] # prints 0, 1
console.log i # prints 1
i = 5
count = (i for i in [0,1])
console.log count # prints [ 0, 1 ]
console.log i # prints 1
Python
i = 5
for i in range(2):
print "for:", i
print "after:", i
i = 5
print [i for i in range(2)] # prints [0, 1]
print "after:", i
Conclusion
The interesting part is how differently languages of the curly-braces family behave. Some consider the for loop its own scope, many don't. It's interesting to compare JavaScript and Perl. They both have a way to tell a variable to be defined in the local scope, but JavaScript simply doesn't let you do that with fors, apparently only functions have scope of their own.
Ruby is similar to Perl in this regard. That little dollar sign can change the scope and that's what let us pull off the trick since loops are important enough to warrant scope at all.
The biggest disappointment is Python. I was honestly expecting the list comprehension to have its own scope, but alas.
Continue reading about A for loop is not a for loop is not a for loop
Semantically similar articles hand-picked by GPT-4
- Freelance teaching is great, or why C/Java/etc. are horrible teaching tools
- Loops are the hardest
- Why people making compilers are superheroes
- My language is better than yours
- Your code doesn't matter
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️