Support for Firebase Auth is one of the most requested features for useAuth and I figured what the heck, how hard can it be?
CodeWithSwiz is a weekly live show. Like a podcast with video and fun hacking. Focused on experiments. Join live Mondays
Friend, it can be very hard. Google docs are shit. The perfect example of For Engineers, By Engineers.
You find out everything that's possible. All the little trees, every detail. Want user/pass auth? Here's the code. Email link? Do this. OAuth with xyz provider? Here.
You know what they don't say?
How to set up fricken Firebase 😡
The setup
// Initialize the FirebaseUI Widget using Firebase.
var ui = new firebaseui.auth.AuthUI(firebase.auth())
Ok Google, how do I import this? Where's my API key?
Is there Google magic afoot and they can guess? Ask me after initialization like the Netlify Identity widget can?
Nope.
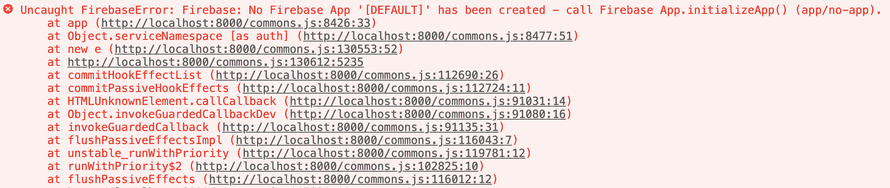
We later found the right docs to follow for initial setup. Even explains how to correctly import for a smaller bundle size.
pt2 will go swimmingly 💪
Creating an auth provider for useAuth
My favorite result of refactoring useAuth from React.Context to XState is that we can support multiple auth providers. Core logic is the same, details pluggable.
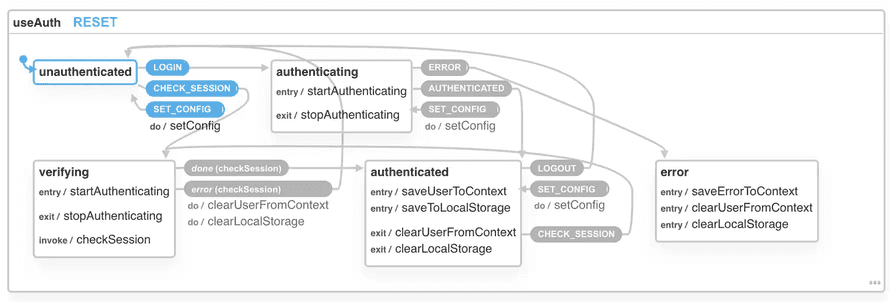
There's an abstraction layer and basic documentation on what to do. We're putting my docs to the test 😅
1. stub your provider
You start with copypasta of the abstract implementation.
// src/providers/YourThing.ts
// Auth Wrapper for Auth0
export class Auth0 implements AuthProviderClass {
private auth0: Auth0Client.WebAuth
private dispatch: (eventName: string, eventData?: any) => void
// Auth0 specific, used for roles
private customPropertyNamespace?: string
// Initialize the client and save any custom config
constructor(params: AuthOptions) {
// You will almost always need access to dispatch
this.dispatch = params.dispatch
// Auth0 specific, used for roles
this.customPropertyNamespace = params.customPropertyNamespace
// Init your client
this.auth0 = new Auth0Client.WebAuth({
...(params as Auth0Options),
})
}
// Makes configuration easier by guessing default options
static addDefaultParams(params: ProviderOptions, callbackDomain: string) {
const vals = params as Auth0Options
return {
redirectUri: `${callbackDomain}/auth0_callback`,
audience: `https://${vals.domain}/api/v2/`,
responseType: "token id_token",
scope: "openid profile email",
...vals,
}
}
public authorize() {
// Open login dialog
}
public signup() {
// Open signup dialog
}
public logout(returnTo?: string) {
// Logs user out of the underlying service
}
public userId(user: Auth0UserProfile): string {
// Return the userId from Auth0 shape of data
}
public userRoles(user: AuthUser): string[] | null {
// Return user roles from Auth0 shape of data
}
public async handleLoginCallback(): Promise<boolean> {
// Handle login data after redirect back from service
// Dispatch ERROR on error
// Dispatch AUTHENTICATED on success
// include the user object and authResult with at least an expiresIn value
}
public async checkSession(): Promise<{
user: Auth0UserProfile
authResult: Auth0DecodedHash
}> {
// verify session is still valid
// return fresh user info
}
}
2. fill abstract implementation
Fill in those methods to fit your auth provider. Here's what we tried for Firebase Auth. Don't know yet if it works.
// src/providers/FirebaseUI.ts
constructor(params: AuthOptions) {
// You will almost always need access to dispatch
this.dispatch = params.dispatch;
// Init your client
this.ui = new FirebaseAuthUI.AuthUI(firebase.auth());
}
public authorize() {
// Open login dialog
this.ui.start("#firebaseui-auth-container", {
signInOptions: [firebase.auth.EmailAuthProvider.PROVIDER_ID]
});
}
Don't quote me on that yet :)
3. use the provider
Use in your app. We created a Gatsby-based example on stream
// gatsby-browser.js
export const wrapRootElement = ({ element }) => (
<ThemeProvider theme={theme}>
<AuthConfig
authProvider={FirebaseUI}
navigate={navigate}
params={
{
/* ... */
}
}
/>
{element}
</ThemeProvider>
)
Render <AuthConfig>
and provide the new auth provider. We don't know yet which params Firebase Auth is going to use. Find out next time :)
4. contribute to useAuth 🙏🏻
To contribute the FirebaseUI auth provider to the core library we needed an extra step 👉 adding it to the library.
That happens in package.json
:
"source": [
"src/providers/auth0.ts",
"src/providers/NetlifyIdentity.ts",
"src/providers/FirebaseUI.ts",
"src/index.ts"
],
// ...
"files": [
"src",
"dist",
"auth0.js",
"netlify-identity.js",
"firebase-ui.js"
],
The "source"
config tells microbundle to build FirebaseUI.ts
as its own library. Creates a bunch of files in dist/*
that aren't connected with the rest.
The "files"
config tells NPM to keep ./firebase-ui.js
when publishing. That file is a hack.
// ./firebase-ui.js
// this is a hack to improve DX
// TODO: figure out a better way
const { FirebaseUI } = require("./dist/FirebaseUI")
module.exports = { FirebaseUI }
Only way I could find to:
- Reduce bundle size and let you use different providers without installing all of them
- Have understandable imports like
import FirebaseUI from "react-use-auth/firebase-ui"
If you know a better way please hit reply.
In conclusion
Understanding beats knowledge. Knowing how several details of Firebase work, does not mean you can make it fit together.
Keep that in mind next time you write docs for your team.
Cheers,
~Swizec
PS: it's beyond cool that TypeScript lets you define "An auth provider must conform to this API" and enforces it at the compiler level. Makes this way easier 😍
Continue reading about [CodeWithSwiz 19] Firebase Auth support in useAuth, pt1
Semantically similar articles hand-picked by GPT-4
- [CodeWithSwiz 21] useAuth beta support for Firebase 🎉
- [CodeWithSwiz 20] Adding Firebase support to useAuth, pt2
- React context without context, using XState – CodeWithSwiz 14, 15
- useAuth – the simplest way to add authentication to your React app
- useReducer + useContext for easy global state without libraries
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️