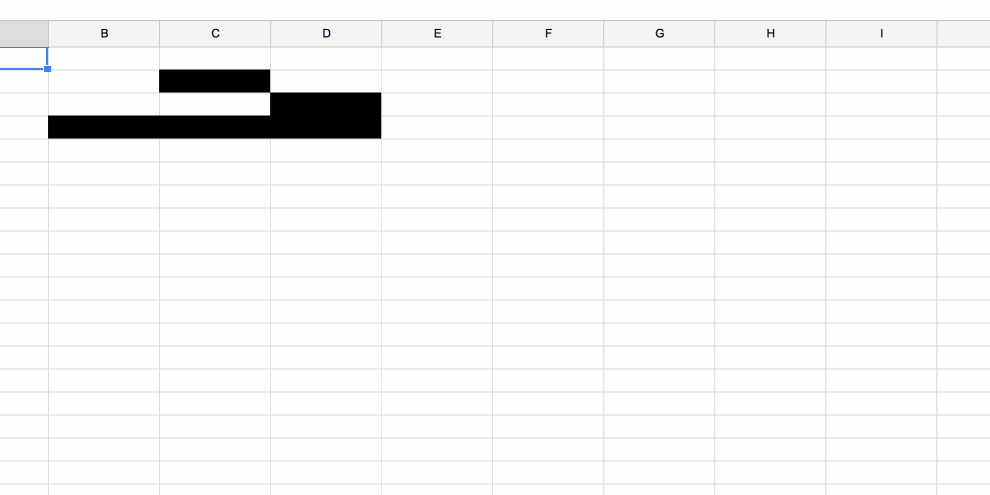
Why? Because.
Have you really never wanted to create Conway’s game of life in a google doc spreadsheet? Really, never? Okay, it’s a pretty weird thing to do. And it’s not as fun as I hoped.
You can play with it, here
I’m giving a 30min presentation on “How to JavaScript” to our not-engineers – the operations and business development teams. But how? It’s not like you can teach somebody JavaScript from scratch in 30 minutes. The most you can do is show them enough to want to learn more.
That’s easier, but still … how? You can’t just show them a bunch of for loops and functions and hope it sticks. You have to get them excited.
The answer I’m trying to answer is: “How can this make my life better?”
They all spend a lot of time in Google Docs. They run a lot of day to day processes in there. Google Docs has scripting capabilities.
See where I’m getting at?
Conway’s Game of Life inside Google Docs, of course. It highlights the basics of reading and manipulating properties of individual cells in spreadsheets, and the game itself is easy to explain:
- Any live cell with fewer than two live neighbours dies, as if caused by under-population.
- Any live cell with two or three live neighbours lives on to the next generation.
- Any live cell with more than three live neighbours dies, as if by over-population.
- Any dead cell with exactly three live neighbours becomes a live cell, as if by reproduction.


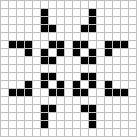
I showed one of them some gifs and he said “Oh dude, I’m excited! That looks so cool”. All the confirmation I needed to go waste 3 hours of my life figuring out how to build this! ?
Scripting Google Docs is hell.
You can’t alert, there’s no console.log, everything is slow, and ES6 works only partially. You get destructuring, but no string templates. I dared not try arrow functions.
Timers also don’t exist. Which means you have to manually step through the game loop by clicking a button. ?
One silver lining is this 3rd party logger called BetterLogger, which lets you Logger.log
things to a separate sheet in your spreadsheet. It’s not very smart, but it helps a lot.
And if you thought DOM was slow, wait ’till you try Docs access. Naively searching through 30x30 cells and counting neighbors took many seconds. Maybe 20 seconds per game tick.
That can be optimized, though. Just access the spreadsheet less.
The first step is to set global vars and add a menu:
// global vars
var maxX = 30,
maxY = 30,
Alive = "#000000";
// almost everything needs a reference to this
var Sheet = SpreadsheetApp.getActiveSheet();
// add menu
function onOpen() {
var spreadsheet = SpreadsheetApp.getActiveSpreadsheet();
var menuItems = [
{ name: "Step game", functionName: "stepGame" },
{ name: "Clear game", functionName: "clearGame" },
];
spreadsheet.addMenu("Conway's game of life", menuItems);
}
Docs runs this code when you open your document. It adds a menu that looks like this:
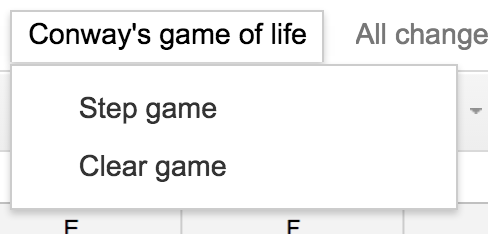
Step Game
runs an iteration of the game loop, Clear Game
clears all cells. Black background means a living cell and white background means a not-living cell.
On each game step, we build a map of living cells, then run the game rules on each. Like this:
function stepGame() {
var range = Sheet.getRange(1, 1, maxX, maxY);
var numRows = range.getNumRows(),
numCols = range.getNumColumns();
var lifeMap = [];
for (var y = 1; y <= numRows; y++) {
lifeMap[y] = [];
for (var x = 1; x <= numCols; x++) {
lifeMap[y][x] = range.getCell(y, x).getBackground() == Alive;
}
}
for (var y = 1; y <= numRows; y++) {
for (var x = 1; x <= numCols; x++) {
conwayGame(lifeMap, y, x);
}
}
}
You can think of the spreadsheet as 2-dimensional memory. First dimension is the row, second is the column. You can store styling information, the value itself, and notes. Docs lets you access directly on both dimension, but it’s slow.
Having 2D memory also means there will be a lot of these nested for loop constructs in your code. You’re limited to O(n2) algorithms at best.
Applying Conway rules to each cell looks like this:
function conwayGame(lifeMap, y, x) {
var neighborPos = [
y > 1 ? y - 1 : 1,
x > 1 ? x - 1 : 1,
y < maxY ? y + 1 : maxY,
x < maxX ? x + 1 : maxX,
];
var livingNeighbors = countLife(lifeMap, neighborPos);
if (lifeMap[y][x]) {
livingNeighbors -= 1;
}
if ((livingNeighbors < 2 || livingNeighbors > 3) && lifeMap[y][x]) {
deactivate(Sheet.getRange(y, x));
} else if (livingNeighbors == 3) {
activate(Sheet.getRange(y, x));
}
}
We count living neighbors, discount the current cell if it’s alive, then decide whether to deactivate
or activate
it. Counting the neighbors literally means walking through a 3x3 array around the current cell and counting cells that have a black background.
Activating or deactivating is a call to cell.setBackground('black')
and cell.setBackground('white')
.
That’s kind of it … I’m embarrassed it took me 3 hours …
Continue reading about Conway’s game of life in Google Docs
Semantically similar articles hand-picked by GPT-4
- Teaching beginners
- Advent of Code Day 22 – Sporifica Virus
- Livecoding #16: canvas.drawImage performance is weird but magical
- Why dataviz does better for your career than TODOapps
- (ab)Using d3.js to make a Pong game
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️