This is hold my beer level of hackery. It didn't work but we learned lots in the process.
CodeWithSwiz is a weekly live show. Like a podcast with video and fun hacking. Focused on experiments and open source. Join live most Tuesday mornings
The problem
On ServerlessHandbook.dev there is a paywall. Every visitor can read the first 30% of every chapter. To read more you have to buy the book or unlock specific chapters with an email.
This works.
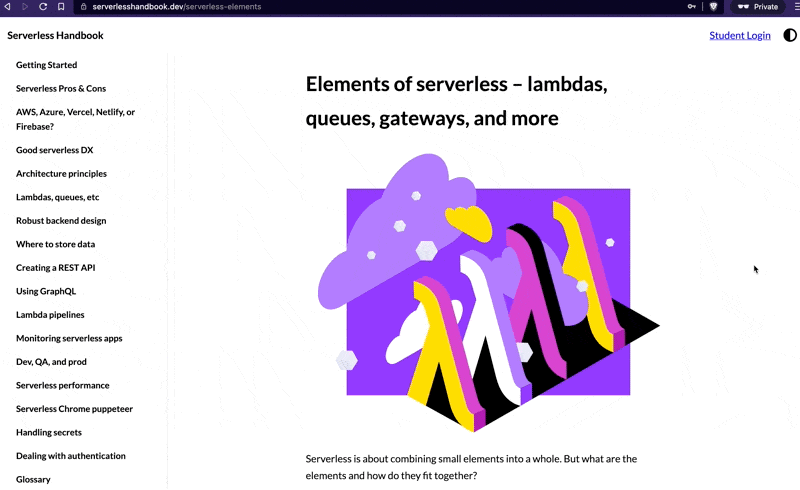
But it doesn't look good. Content's not styled.
You're supposed to see this:
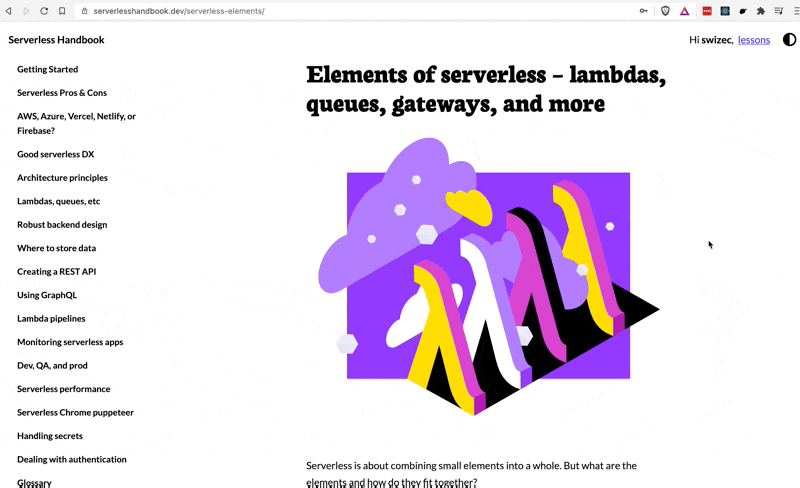
Current working solution
You get unstyled content because of how the paywall works.
// rendering the main content
{
contentUnlocked ? (
<main id="content">{props.children}</main>
) : (
<main id="content">
<SnipContent>{props.children}</SnipContent>
</main>
)
}
If content unlocked, show content. If content locked, snip.
The snipping is a hack:
export function SnipContent({ children }) {
const html = ReactDOMServer.renderToString(children).split(
'<div id="lock"></div>'
)[0]
return <div dangerouslySetInnerHTML={{ __html: html }} />
}
Take the children, render to a string, split by the lock, take first part, render back out as HTML.
When this happens, we throw out all the styling machinery. ThemeUI doesn't see these components, doesn't add CSS classes.
Wrapping in ThemeUI's root wrapper doesn't work either. It's a CSS-in-JS library and doesn't add global tag-based styling. Needs to see the details.
Attempt 1: Hack React's AST
We have those ThemeUI classes βΒ they're in the children
prop!
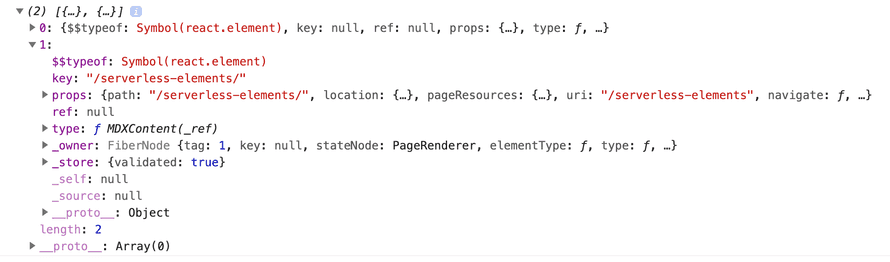
The children
prop is a portion of React's AST. An abstract syntax tree that represents the data structure of your React app. React uses this to run DOM events, deal with effects, and render.
Our content hides in the type: MDXContent
node. We weren't able to dig into that.
For a brief moment it looked like we might be able to hack React's Fiber implementation, but that was too much. If I understand correctly, fibers are the syntax tree that React uses, but they're based on functions calling functions. Not a hackable data structure.
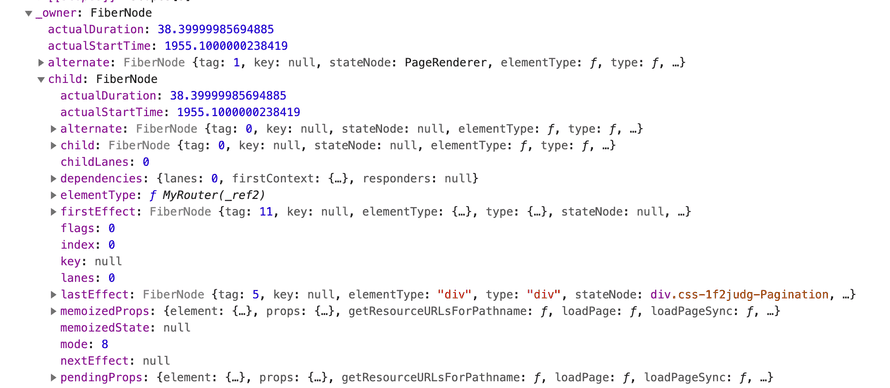
Attempt 2: Render to HTML, parse back to JSX
Next idea π what if we render to HTML, snip the content, parse back to JSX, and pass that back to the main rendering machine?
import parseToReact from "html-react-parser"
// ...
const html = ReactDOMServer.renderToString(children).split(
'<div id="lock"></div>'
)[0]
const snippedChildren = parseToReact(html)
console.log(snippedChildren)
return snippedChildren
html-react-parser is a library that takes any HTML and parses it to a JSX string.
And it worked! We got an understandable data structure of our content π₯³
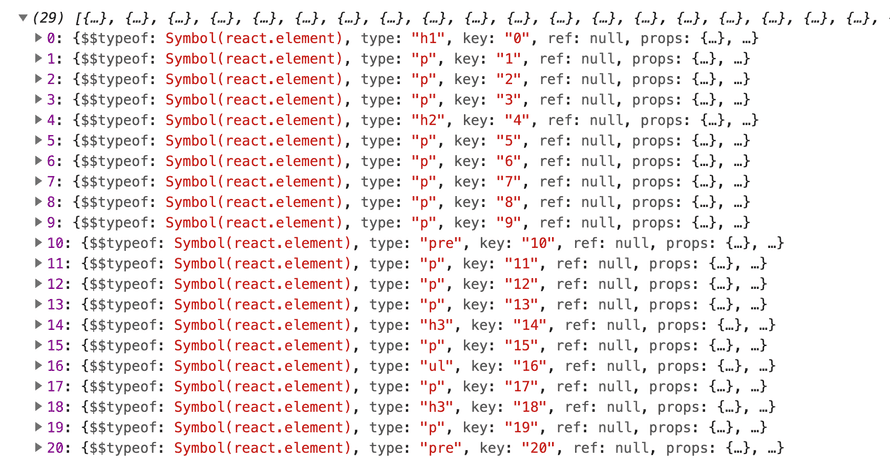
You can look at that and understand what's going on! React components have normal types, there's props, it all makes sense.
Except it doesn't style. ThemeUI doesn't run through these, doesn't add CSS props, doesn't do squat. π₯²
Attempt 3: Render as MDX
What if you took the HTML or the parsed JSX and shoved that in a MDX renderer yourself?
import { MDXProvider } from "@mdx-js/react"
import { MDXRenderer } from "gatsby-plugin-mdx"
// ...
return (
<MDXProvider>
<MDXRenderer>
{snippedChildren} // or {html}
</MDXRenderer>
</MDXProvider>
)
Cryptic error from the bowels of Gatsby. Something undefined.
MDXProvider
specifies components for MDX. Where the styling and any custom machinery hooks into.
MDXRenderer
takes a compiled MDX source and renders it as React.
And that's the rub π compiled MDX source. We don't have that. We have random HTML or React components. MDX chokes and dies.
You could compile with mdx(data)
from the @mdx-js/mdx
package, but that depends on Node.js libraries that don't work inside React components. You have to guarantee running on the server.
Including the full MDX runtime in your client code would work ... and destroy your Lighthouse scores. It's a big pile of code.
What I feared: The real solution
We're going to need a real solution βΒ an MDX/Remark plugin that knows how to grab the snipped content from our data source. π©
Next time!
Cheers,
~Swizec
Continue reading about Hacking the React AST for fun and profit β #CodeWithSwiz ep34
Semantically similar articles hand-picked by GPT-4
- A fun security bug with static rendering β CodeWithSwiz #31
- Sometimes your worst code is your best code
- Your first NextJS app β CodeWithSwiz
- Building a small CMS with NextJS, pt2 β CodeWithSwiz
- Exploring NextJS with a headless CMS, pt4 β CodeWithSwiz
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below π
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails π on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. π"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help π swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers π ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are β€οΈ