Today, I had a dumb idea: "Nested superagent calls sure look messy… maybe I should use fetch() instead…”
What happened next was a little bit of this:
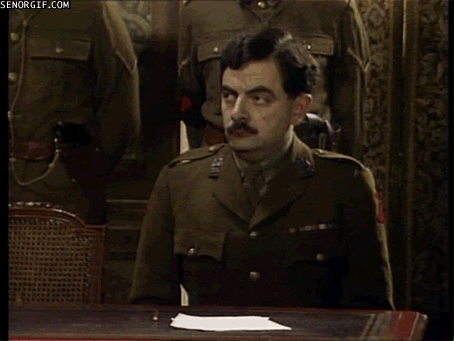
Followed by a lot of this:
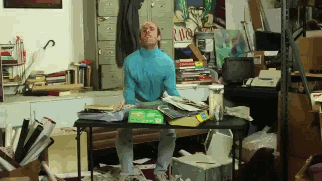
In case you don't know, superagent is a popular library for executing AJAX requests in JavaScript. Clean API, works well, makes life easy. I've been a fan for years.
fetch() is a new JavaScript API for the same. Clean API, Promise-based interface, solves a problem you thought was solved. Not yet an official standard, but supported in all modern browsers. You can use it in production code, if you're compiling with Babel and enable babel-polyfill
.
Both superagent and fetch() enable you to talk to a server. The first produces clean code that gets nesty if you need many things. The second produces clean code that is Promis-y and sometimes cumbersome.
For example, to get a JSON object with superagent, you'd do this:
superagent.get('/api/some.json')
.set('Accept, 'application/json')
.end((err, res) => {
// res.body contains parsed JSON
});
The same call with fetch() looks like this:
fetch("/api/some.json", { headers: { Accept: "application/json" } })
.then((res) => res.json())
.then((json) => {
// json contains parsed JSON
})
Both just 5 lines of code. It’s debatable which is cleaner. They look the same to me. ¯_(ツ)_/¯
Curated Fullstack Web Essays
Get a series of curated essays on Fullstack Web development. Lessons and insights from building software for production. No bullshit.
Where it gets interesting is when you have two calls that rely on each other. Observe:
superagent.get('/api/some.json')
.set('Accept, 'application/json')
.end((err, res) => {
const url = `/api/details/${res.body.details_id}.json`;
superagent.get(url)
.set('Accept', 'application/json')
.end((err, res) => {
// res.body contains parsed details
});
});
Vs.
fetch("/api/some.json", { headers: { Accept: "application/json" } })
.then((res) => res.json())
.then((json) => {
const url = `/api/details/${json.details_id}.json`
return fetch(url, { headers: { Accept: "application/json" } })
})
.then((res) => res.json())
.then((json) => {
// json contains parsed details
})
The promises approach does look cleaner ?
But there's a catch with fetch()
See the Accept
header? Superagent sends it as an Accept
header. Fetch sends it as accept
.
Your clean code stops working. You're doing everything right: you send the Accept
header, you send the Authorization
header for your API's token-based authentication, and yet…
TIL: Rails doesn't think HTTP headers are case-insensitive.
— Swizec Teller (@Swizec) October 31, 2016
fetch() knows they are and forces lowercase pic.twitter.com/3DyMz7mgc6
You fall into a rabbit hole…
Rails can still get the token out of Authentication header, but returns 401 anyway.
— Swizec Teller (@Swizec) October 31, 2016
This is what I get for trying to uses Promises.
You read through all of the relevant questions and answers on StackOverflow. You google and google. You spelunk through Rails's code on Github.
You abandon all hope…
Oh great, looks like it's actually Warden bug deep inside the bowels of Devise.
— Swizec Teller (@Swizec) October 31, 2016
All I wanted was to use fetch() 😭
You're about ready to start throwing things. Nothing makes sense, this is dumb, everything sucks, all you wanted was to Do The Right Thing™ and now you're stuck debugging huge frameworks.
?
By the way, Devise is a library for user authentication, and Warden is the core authentication library it wraps. No, I don't know why this happens in two libraries. Maybe historical reasons.
And then it hits you. You're being an idiot.
The difference between superagent and fetch() isn't that one sends your headers as-given and the other lowercases their names. The difference is that superagent sends a cookie and fetch() doesn't!
Oh god ... the REAL issue is that fetch() doesn't automatically send Cookies and I didn't notice.
— Swizec Teller (@Swizec) October 31, 2016
Fuck my life. pic.twitter.com/hWbxA8bvzV
Your API relies on tokens to authenticate the client, and on session cookies to identify users. ? It makes total sense, super common design. But ugh!!
You have to add credentials: 'same-origin'
to your fetch() settings object. Then it works. The API talks to you, the JavaScript client does its thing, users are happy.
Code looks like this:
fetch("/api/some.json", {
headers: { Accept: "application/json" },
credentials: "same-origin",
})
.then((res) => res.json())
.then((json) => {
const url = `/api/details/${json.details_id}.json`
return fetch(url, {
headers: { Accept: "application/json" },
credentials: "same-origin",
})
})
.then((res) => res.json())
.then((json) => {
// json contains parsed details
})
In real code, I suggest wrapping fetch() in a helper function that always adds API-specific options like Accept
and Authorization
headers and credentials
. Your future self will thank you.
No, I don't know why fetch() breaks the 20-year old convention that cookies are automatically included in requests.
Continue reading about How to waste hours of life with fetch() and a bit of brainfart
Semantically similar articles hand-picked by GPT-4
- A tiny ES6 fetch() wrapper that makes your life easier
- JavaScript can fetch() now and it's not THAT great
- How GraphQL blows REST out of the water
- Mocking and testing fetch requests with Jest
- REST API best practice in a GraphQL world
Want to become a Fullstack Web expert?
Learning from tutorials is great! You follow some steps, learn a smol lesson, and feel like you got this. Then you go into an interview, get a question from the boss, or encounter a new situation and o-oh.
Shit, how does this work again? 😅
That's the problem with tutorials. They're not how the world works. Real software is a mess. A best-effort pile of duct tape and chewing gum. You need deep understanding, not recipes.
Leave your email and get the Fullstack Web Essays series - a series of curated essays and experiments on modern Fullstack Web development. Lessons learned from practice building production software.
Curated Fullstack Web Essays
Get a series of curated essays on Fullstack Web development. Lessons and insights from building software for production. No bullshit.
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️