A while ago I wrote about my school project that involves generating pretty trees and concluded the post with the idea that I now have to implement some way for the branch lengths to be a bit random to add more variability.
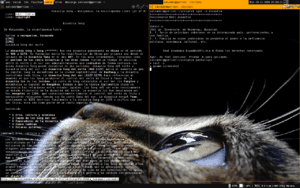
There is an easy and an awesome way to doing this. The easy way involves doing everything as usual and then simply adding a small random buzz, fuzz or whatever you could call it to the length of a branch. Obviously this approach would work and the branches wouldn't be of uniform length anymore ... but there's just no fun in that. The results it produces also aren't quite that awesome.
So I chose a different way.
First a little background on how branch lengths were calculated originally. Every time a branch is needed the basic brench length is multiplied with a factor chosen based on how deep inside the tree we are. So for example, if the trunk is of length 5 and we are on the third level of the tree the length would be 5*0.25, or a quarter of the trunk's length.
I wanted to expand on that by randomly selecting a multiplier from the list for every branch I'm looking at. As you can probably expect, this produced rather funny looking trees.
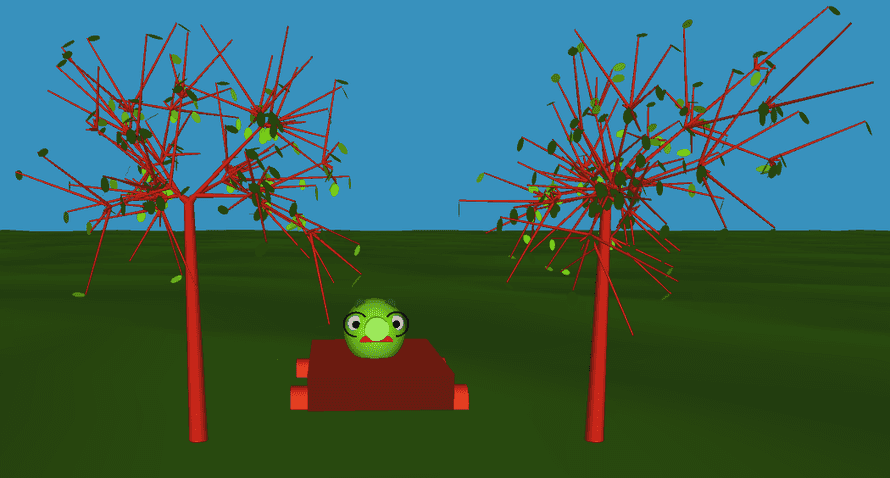
What's needed is a way to randomly choose the length of a branch, but probabilistically making sure that branches on deeper levels of the tree are shorter than the ones before them. This leads us to the idea of needing a weighed random that has a slightly higher probability of returning a certain length over others.
After a bunch of googling on how such a thing might be achieved I settled on a pretty simple solution. Basically produce a list of possible indexes, tweaked so there's more of those that need a higher probability, and then simply picking a random one. Something like so:
(nth choices
(rand-nth (0 0 0 0 0 0 0 0 0 0 1 1 1 1 1 1 1 1 2 2 2 2 2 2 3 3 3 4))
This, however, isn't very elegant or even pretty to look at. Changing it so the maximum of the probability distribution moves down the choices depending on how deep inside the tree we are would be painful.
My next step was changing the weighed random choice so it would generate such a horrible list on its own and use a provided function to calculated the weights for every specific index. This gives us a way to neatly define the probability distribution we want every time we make a random choice from a list.
Here's what the final function looks like
(defn weighed-random-choice [choices weight]
(defn indexes []
(flatten (map #(replicate (weight %1) %1)
(take (count choices)
(iterate inc 0)))))
(nth choices
(rand-nth (indexes))))
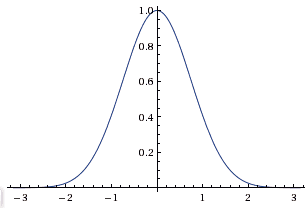
To be honest I still haven't quite figured out a good probability distribution to get perfectly looking trees, but here's my current weight function. I like to think of it as mathematically somewhat elegant, but it might be a bit slow to calculate at times ... seems to have quite an impact on generating trees when the maximum depth is big-ish. And I need it to have a bigger drop off on the left side where the longer branches are.
(defn weight [x pivot]
; cos(x + sin(x)*0.9)*0.5+0.5
(let [x (* x (/ Math/PI (count lengths)))
pivot (* pivot (/ Math/PI (count lengths)))]
(int (Math/floor (* 10
(+ 0.5 (* 0.5
(Math/cos (+ (- x pivot)
(* 0.9 (Math/sin (- x pivot))))))))))))
Pivot is where the highest probability density needs to be and usually denotes the current depth we are at. If you see anything wrong with my approach go ahead and tell me :)
Continue reading about Implementing a weighed random choice in Clojure
Semantically similar articles hand-picked by GPT-4
- Using prime numbers to generate pretty trees
- Haskell and randomness
- An elegant way to randomly change every list member in Haskell
- Checking for primes? Dumber algorithm is faster algorithm
- A message from your future self
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️