This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding ?. Always under 500 words and with pictures. You can follow my channel, here. New content almost every Sunday at 2pm PDT. There’s live chat, come say hai ?
This week, we wanted to build an example for the TransitionableComponent from last week — an animated piechart. Not because piecharts are exciting, but because someone asked me by email.
It did not go so well. After 1 hour and 45 minutes of fighting everything from Webpack to npm, we built this:
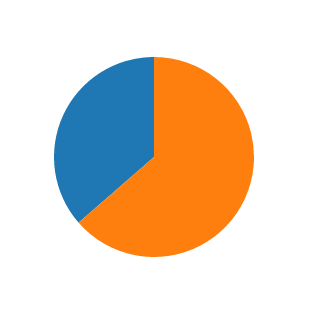
A not animated piechart that shows the ratio of British imports vs. exports in the year 1820. Exports are blue. The tiny dataset is from A Handbook of Small Data Sets.
We were going to build an animation that walks through the years in our dataset – 1820 through 1850 – and draws a 2-arc piechart for each year. If there was time, we’d add the ability for users to scroll through.
Building the piechart itself was quick: Fetch and parse data with d3.tsv('data.dat')
, call setState
and build a 2-entry array as data for the piechart. Our tiny dataset gives only one number, so we have to infer the other.
// src/App.js
componentDidMount() {
d3.tsv('data.dat')
.row(({imports, year}) => ({imports: Number(imports),
year: Number(year)}))
.get((data) => {
this.setState({data: data,
currentIndex: 0});
});
}
render() {
let pie = null;
if (this.state.data.length) {
const yearData = this.state.data[this.state.currentIndex],
pieData = [{value: yearData.imports},
{value: 100-yearData.imports}];
pie = <animatedpiechart data={pieData} x="400" y="300" r="100">;
}
// return an svg, add the pie, etc
}
}
</animatedpiechart>
That’s how you’d use the AnimatedPiechart
component once it works. The component itself wasn’t quick to build, too. It looks like this:
// src/AnimatedPiechart.js
class Arc extends Component { // this would be TransitionableComponent
render() {
const arc = d3.arc()
.innerRadius(this.props.innerRadius)
.outerRadius(this.props.outerRadius);
return (
<path d={arc(this.props.data)} style="{{fill:" this.props.color}}="">
);
}
}
const AnimatedPiechart = ({ x, y, r, data }) => {
let pie = d3.pie()
.value((d) => d.value)(data),
translate = `translate(${x}, ${y})`,
colors = d3.scaleOrdinal(d3.schemeCategory10);
return (
<g transform={translate}>
{pie.map((d, i) => (
<arc key={`arc-${i}`} data={d} innerradius="0" outerradius={r} color={colors(i)}>
))}
</arc></g>
);
};
</path>
A piechart is a collection of arcs. As such it doesn’t need to do much: use d3.pie()
to turn a dataset into a piechart, then walk through it and render arcs.
The arcs were supposed to use TransitionableComponent
to become animated, but … heh … it didn’t actually work as a library. Published to npm and everything. 29 people even tried to use it! Couldn’t even import ?
Calling import TransitionableComponent from 'react-transitionable-component'
produced a syntax error. Something like “Unexpected token: export in index.js”.
When you publish a library, it has to be compiled. Nobody wants to build their whole node_modules directory every time they run Webpack, so configs always exclude it.
I wonder how long build all your dependencies would take … I should try that one day ?
So, to make a library work as a library, you have to compile it. To compile it, you have to first know how to run Webpack. It took me an embarrassing amount of time to realize that webpack webpack.config.prod.js
does not run Webpack. webpack ––config=webpack.config.prod.js
does.
To save some time, we modeled our webpack config after what we found in react-scripts. Also known as create-react-app
. When I say “model”, I mean “copy file then delete cruft”.
You can delete everything to do with HTML and CSS. Unless your packaged component comes with its own styling, which I’m still on the fence about. Should open source components include stylings like jQuery plugins once did?
Our final webpack config that got the AnimatedPiechart project running comes out to 74 lines and is too long to paste here. You can see it on Github.
We also had to install 16 dependencies, which is insane. And we’re no closer to knowing how to correctly use d3 v4 modularity and avoid depending on the whole library. ?
But, you can use react-transition-group as a library now \o/
Join me next time, when we use TransitionableComponent
to build an animated piechart for real. Maybe even figure out how to do import Thing from 'd3-transform'
properly.
PS: the edited and improved versions of these videos are becoming a video course. Readers of the engineer package of React+d3js ES6 get the video course for free when it’s ready.
Continue reading about Livecoding #19: It’s hard to package a library
Semantically similar articles hand-picked by GPT-4
- Livecoding #21: Use Babel for libraries, not Webpack
- Livecoding #18: An abstract React transition component
- Silky smooth Piechart transitions with React and D3.js
- Livecoding #34: A Map of Global Migrations, Part 3
- Livecoding Recap: A new more versatile React pattern
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️