Epic stream Friend! We used a man-in-the-middle attack to snoop an app's API traffic, replayed it in a GraphQL client, and successfully put it inside an AWS Lambda 🤘
CodeWithSwiz is a weekly live show. Like a podcast with video and fun hacking. Focused on experiments and open source. Join live most Mondays
For Valentine's day The Girl got a Lovebox – an IoT device that accepts love notes, spins a 3D printed heart, and shows your note on a color screen. She loved it. 😍
And you know how this goes, right?
Day 1: Send 20 notes
Day 2: Send 2 notes
Day 3:
Day 4:
...
But if you could automate it to send cute notes and pictures from your relationship at random intervals 🤔
Got a thing for The Girl.
— Swizec Teller (@Swizec) February 15, 2021
It’s a box that receives love notes and photos. And there’s an API. Gonna lovecode a idea tomorrow ✌️ pic.twitter.com/1PAFENFydc
The plan
Lovebox's marketing team says "Send notes to your Lovebox from an app, it's cute and awesome and shows Your Person you thought of them!"
As a nerd that reads: "We have an API."
How else would it work? There must be a server. Apps send API requests to the server, server reads a lookup table, sends message to the box – a tiny computer – through another API.
- Read API traffic from app to server
- Find request that sends a message
- Replay request
- Create AWS Lambda that runs on schedule
- Send random notes
Step 0: Fail to hack the API
Lovebox has a webapp and that's a great first target. Log into the app, open Chrome's network tab, see a tonne of graphql requests, send a message.
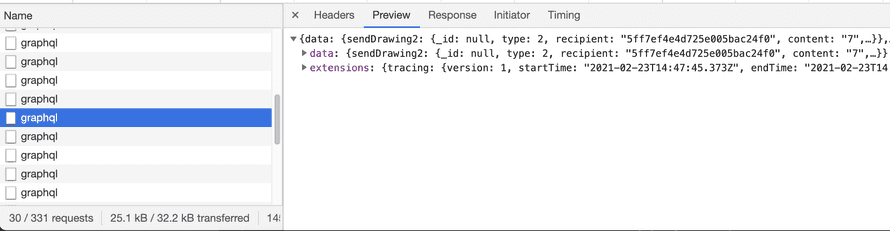
We got it!
Alas, the webapp doesn't work with v2 of the Lovebox. Old API, no support for color. When you click the upgrade button your box can get color pictures, but not requests from the old API. 💩
Snooping iOS traffic stumped us.
Step 1: Use Charles Proxy to snoop iOS traffic
Off stream I discovered Charles Proxy, a tool for "debugging" web traffic.
You run the app on your phone, enable proxying, set up SSL proxy, and use the Lovebox app. Charles Proxy convinces your iOS networking layer to send every request through the app.
SSL proxying is important. It lets you read the content of encrypted https connections and the target app doesn't even notice. Classic man-in-the-middle attack.
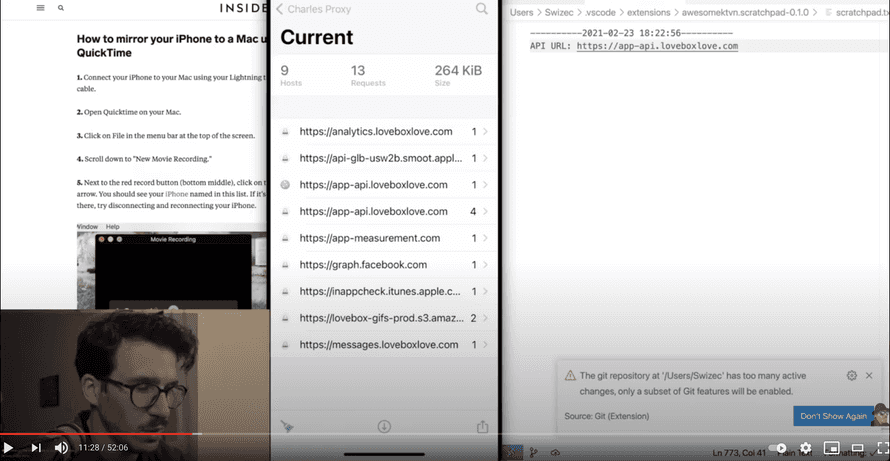
You get a request trace that you can export to the Charles Proxy desktop app.
Step 2: Dig through the API trace
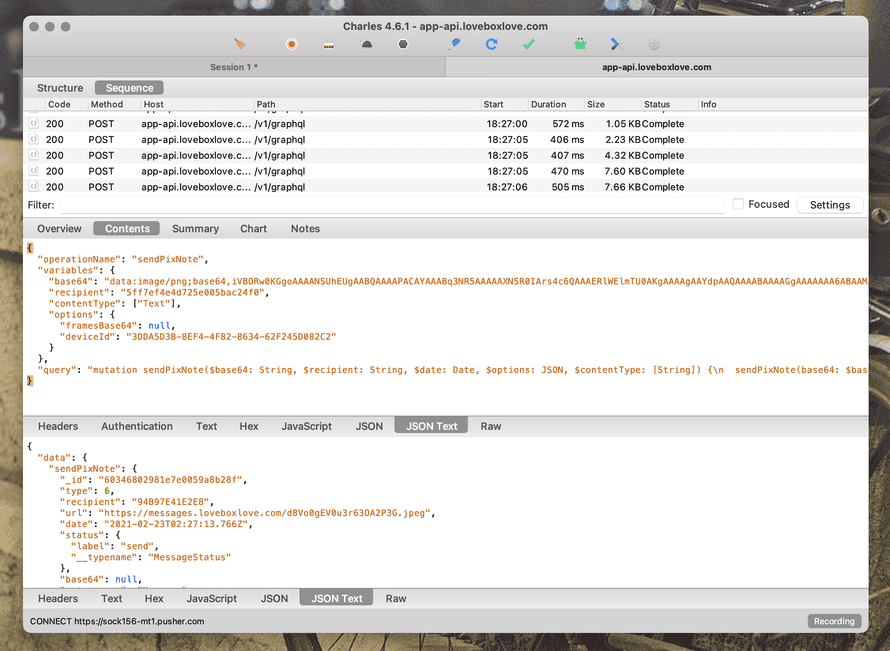
We dug through the trace and found the GraphQL request that sends a note – sendPixNote2
.
Looks like every note is sent as a base64-encoded PNG image. We tried pictures and text and they all looked like this.
The recipient
must be my Lovebox's ID, contentType
needs further exploration but ["Text"]
and ["Image"]
both worked, and deviceId
looks like my phone. Not sure that's important.
GraphQL ~~query~~ mutation in the request looks like this:
mutation sendPixNote(
$base64: String
$recipient: String
$date: Date
$options: JSON
$contentType: [String]
) {
sendPixNote(
base64: $base64
recipient: $recipient
date: $date
options: $options
contentType: $contentType
) {
_id
type
recipient
url
date
status {
label
__typename
}
base64
__typename
}
}
Nice thing about reverse-engineering GraphQL is that every request tells you what it's doing. Downside is you have to click on every request because they're all to the same endpoint.
Step 3: Replay requests in GraphQL client
Before you start coding, you gotta verify you can hack the API. We used a desktop GraphQL Client.
Start easy, can you send requests at all?
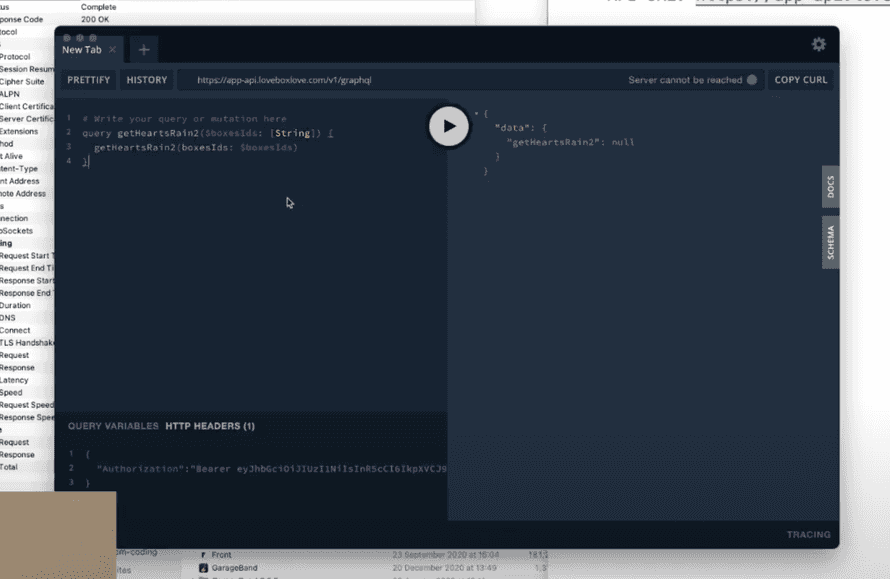
Add an authorization header with a JWT token Authorization: Bearer ...
– copypasta'd from our API trace – use the right GraphQL endpoint, and press play.
it worked 🎉
Step 4: Your first modified request
We tried a few more requests and confirmed that sending works. sendPixNote
using the same data made our heart spin.
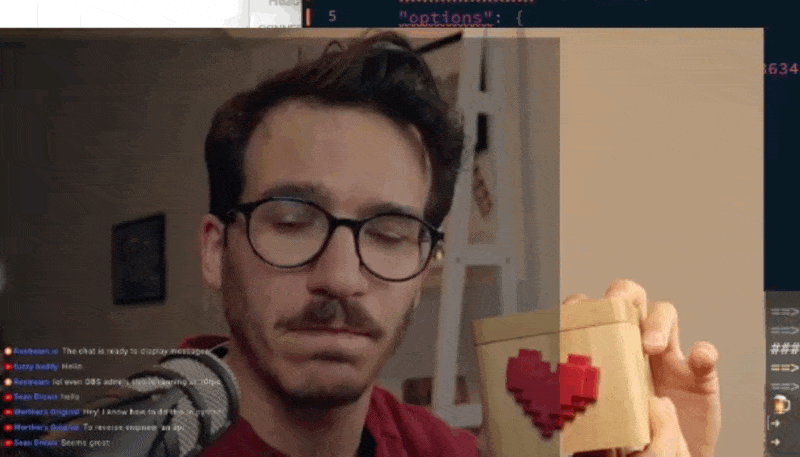
Fantastic! Now you know it's gonna work.
Next we tried a modified request with a custom payload. Found an image online, converted to base64, pasta'd in there.
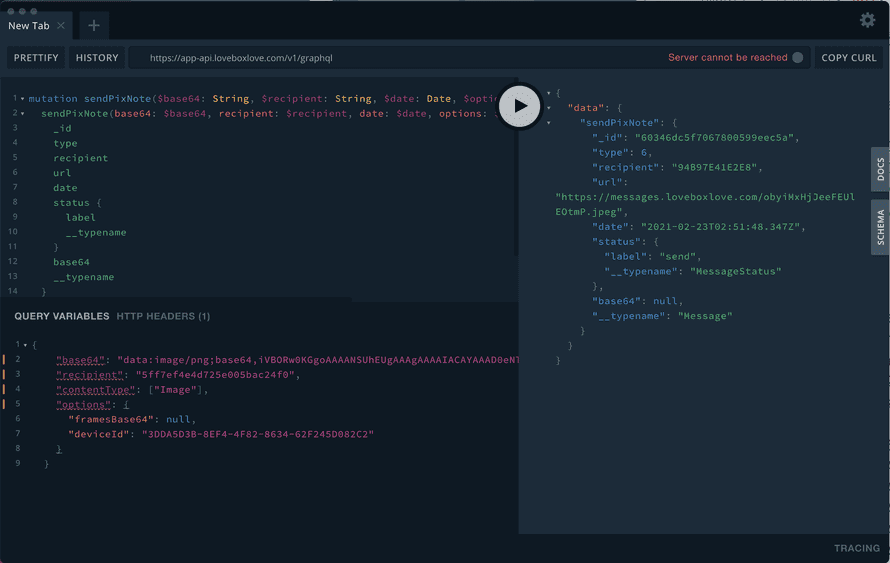
Apollo's GraphQL Playground client lets you do that by changing variables bottom left. Much easier than futzing around with REST.
And the Lovebox displayed a blue fish.
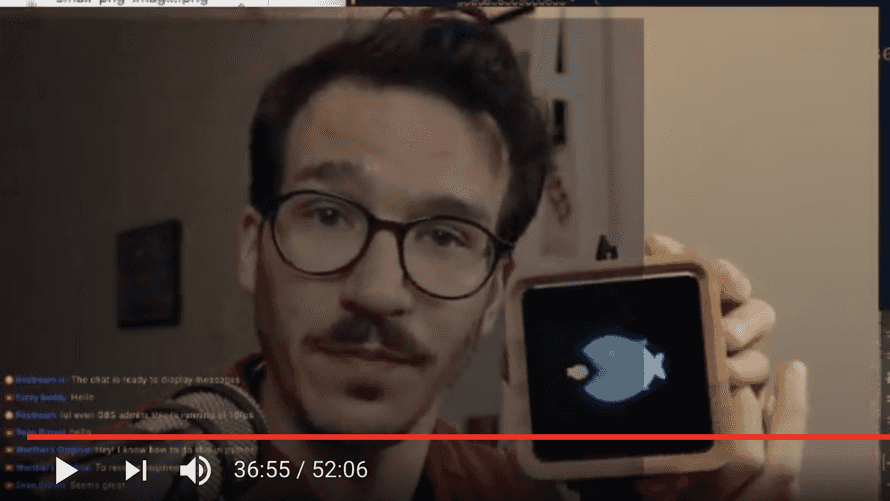
Huzzah!
Step 5: Copy request into an AWS Lambda
Request works, next we gotta move into a Lambda. We used Prisma's minimalist graphql-request library.
// src/lovebox.ts
import { request, gql, GraphQLClient } from "graphql-request"
const client = new GraphQLClient("https://app-api.loveboxlove.com/v1/graphql", {
headers: {
// TODO: figure out how to login
Authorization: "Bearer ...",
},
})
// copypasta the variables
const variables = {
base64: `data:image/png;base64,...`,
recipient: "...",
contentType: ["Image"],
options: {
framesBase64: null,
deviceId: "...",
},
}
export const sendNote = async () => {
const res = await client.request(
gql`
// copypasta the mutation
`,
variables
)
console.log(res)
}
sendNote
is an async method invoked by AWS Lambda as a cloud function. It takes the initialized and authenticated GraphQLClient
, feeds it the same mutation and variables we had before, prints the result.
Yes I pasted the whole base64 string for a 16KB image. Redacted 😅
Configure in serverless.yml
as a function with no triggers.
# serverless.yml
service: lovebox-lambda
provider:
name: aws
runtime: nodejs12.x
stage: dev
functions:
sendNote:
handler: dist/lovebox.sendNote
package:
exclude:
- node_modules/typescript/**
- node_modules/@types/**
Name the project lovebox-lambda
, set basic provider config, declare a function, exclude typescript cruft on deploy.
Later we'll add a cronjob trigger.
Step 6: Test your AWS Lambda locally
$ sls invoke local -f sendNote
Well shit that’s satisfying #codeWithSwiz pic.twitter.com/j5UYUAl6L5
— Swizec Teller (@Swizec) February 23, 2021
It worked! 🥳
Next episode we'll take this lambda, hook it up to an S3 bucket, and automate the shit out of my boyfriend duties.
Cheers,
~Swizec
[sparkjoy|code-with-swiz-24-lovebox1]
Continue reading about Reverse engineer a GraphQL API to automate love notes – CodeWithSwiz 24
Semantically similar articles hand-picked by GPT-4
- Send daily random photos from an S3 bucket using AWS Lambda – CodeWithSwiz 25
- Can you automate love?
- Serverless file upload to S3 with NextJS and AWS Lambda – CodeWithSwiz 27
- My favorite serverless project
- CodeWithSwiz: Privacy-focused embeds for YouTube, Twitter, et al
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️