
.listen(app.get("port"), function () {
console.log("Express server listening on port " + app.get("port"))
}))
var io = require("socket.io").listen(server)
io.set("log level", 0)
// the important parts of echo server
io.sockets.on("connection", function (socket) {
socket.on("echo", function (msg, callback) {
callback = callback || function () {}
socket.emit("echo", msg)
callback(null, "Done.")
})
})
After not forgetting to load /socket.io/socket.io.js into the index page, I can now run the server, point my browser to http://localhost:3000 and play around in the console like this:
> var socket = io.connect("http://localhost:3000")
undefined
> socket.on("echo", function (msg) { console.log(msg); })
SocketNamespace
> socket.emit("echo", "Hello World")
SocketNamespace
Hello World
Automating the test
Typing commands into a console, even clicking around a webpage is a rather arduous and boring process. The easiest way I've found to automate this is using Mocha and socket.io-client.
First thing we're going to need is requiring everything and making sure the socket.io server is running.
var chai = require('chai'),
mocha = require('mocha'),
should = chai.should();
var io = require('socket.io-client');
describe("echo", function () {
var server,
options ={
transports: ['websocket'],
'force new connection': true
};
beforeEach(function (done) {
// start the server
server = require('../app').server;
done();
});
See, simple :)
Now comes the interesting part, the actual test making sure our server does in fact echo what we ask it to.
it("echos message", function (done) {
var client = io.connect("http://localhost:3000", options)
client.once("connect", function () {
client.once("echo", function (message) {
message.should.equal("Hello World")
client.disconnect()
done()
})
client.emit("echo", "Hello World")
})
})
The idea behind this test is simple:
- Connect client to server
- Once there's a connection, listen for echo event from the server
- Emit echo event to the server
- Server responds and triggers our listener
- Listener checks correctness of response
- Disconnects client
Disconnecting clients after tests is very important. As I've discovered, not disconnecting can lead to the socket accumulating event listeners, which in turn can fire completely different tests than what you expect. It also leads to tests that pass 70% of the time, but fail in random ways.
In the end, our efforts are rewarded by a happy nyan cat.
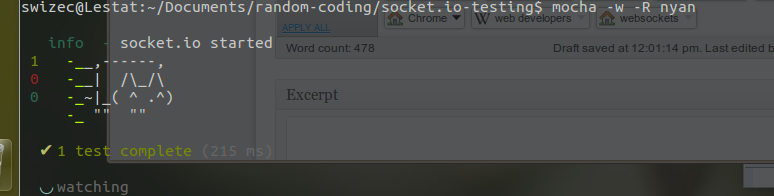
PS: you can see all the code on github.
Maria Ramos from Webhostinghub.com/support/edu has translated this post into Spanish.
Continue reading about Testing socket.io apps
Semantically similar articles hand-picked by GPT-4
- Testing Backbone apps with Mocha
- Elegantly using socket.io in backbone apps
- Blackbox testing node.js apps
- Simple trick that lets you code twice as fast
- Learning WebRTC peer-to-peer communication, part 2 – connecting 2 browsers on different devices
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️