Ever built code that's like a Jenga tower of conditional logic?
It always starts off easy. If A is true, show X, otherwise Y.
Oh but if B is true then show Z, unless A is also true, then you need Y and Z. And if C happens after A is true, but not after B is true, then you ...
Aaaaand you've got a mess. A piece of code that's going to be fun to maintain.
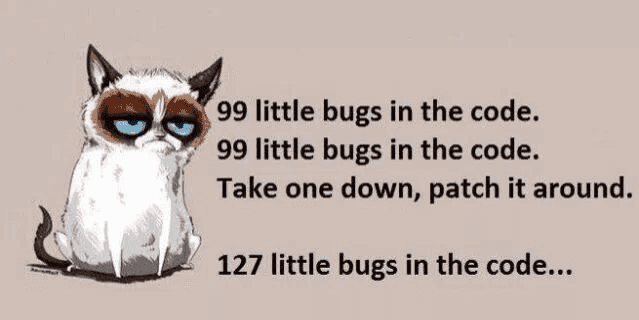
This happens a lot. Sometimes fast, sometimes after months of polishing your logic to include every edge case.
And that's okay. Messes happen.
It's what you do with your mess that matters.
fuck I think it's time to try XState, I got ifs up the wazoo
— Swizec Teller (@Swizec) July 30, 2020
How you create a mess
Here's a mess, I made it just for you ❤️
Check the checkboxes to get different colors. Looks silly in this example and I assure you it happens all the time.
Like when I was building an insurance intake form that shows fields depending on previous answers and what the API says is even possible in this particular case 😅
Messes happen when you're dealing with a combinatorial explosion. Every new condition doubles the number of possible states.
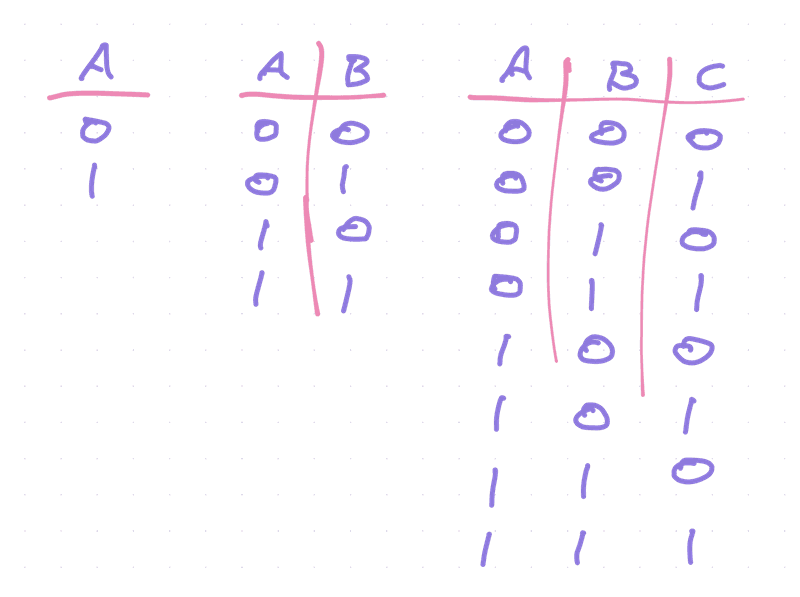
3 variables give us 8 possible states. 4 makes 16. 5 is 32.
That's booleans.
Imagine enums with 4 possible states. 3 of those and you're looking at 64 combinations.
🤯
Your first inclination is to write chains of conditionals. Cover the 3 or 4 happy-path cases and done.
The other 60 are edge cases you find in production through bug reports. You fix those.
And you create long and complex conditionals that nobody on your team can read. Each bug fix creates 5 new bugs.
Everything's connected, nothing makes sense.
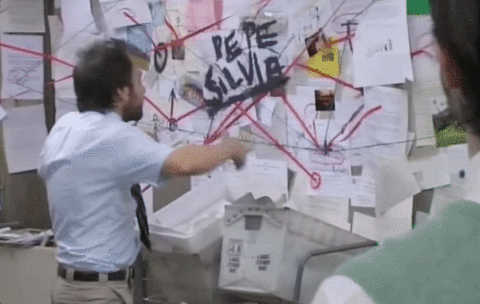
How you fix the mess with hashmaps
Your way out of this mess is a stable mapping of states to values. Easiest to do with a hashmap.
You can start with a truth table to get your bearings. Great for boolean logic, difficult for enums. You run out of dimensions 😅
Truth table for the mess above looks like this:
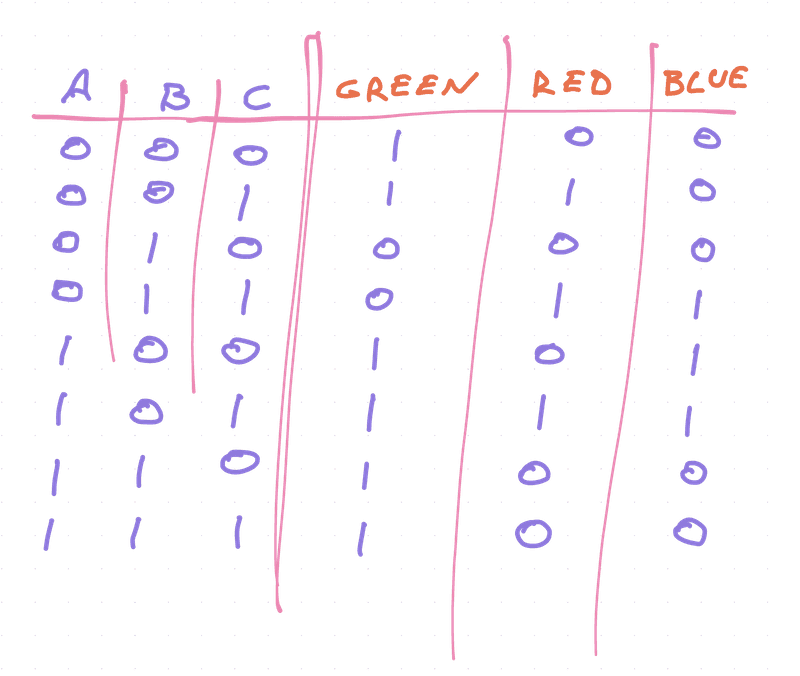
You can turn that into a hashmap of states. A piece of data that tells you how the code behaves.
const STATE_MAP = {
"0-0-0": { green: 1, red: 0, blue: 0 },
"0-0-1": { green: 1, red: 0, blue: 0 },
"0-1-0": { green: 0, red: 0, blue: 0 },
"0-1-1": { green: 0, red: 1, blue: 1 },
"1-0-0": { green: 0, red: 0, blue: 1 },
"1-0-1": { green: 1, red: 1, blue: 1 },
"1-1-0": { green: 1, red: 0, blue: 0 },
"1-1-1": { green: 1, red: 0, blue: 0 },
}
And you use it like this:
const key = `${Number(a)}-${Number(b)}-${Number(c)}`
const showGreen = STATE_MAP[key].green
const showRed = STATE_MAP[key].red
const showBlue = STATE_MAP[key].blue
😍
Isn't that more readable, easier to understand, and way easier to debug? Got a problem, change the map. Done.
How you fix the mess with state machines
What a hashmap can't do, is guard against impossible states. What if you don't want users to click A when B and C are true? 🤔
That's when you need a state machine, my friend. They're fun to build, tricky to get right, and different to think about than you're used to.
useReducer
is a state machine. Redux, too. useState
if you squint your eyes.
Here's a state machine for each of the useState
s in our smol mess example.
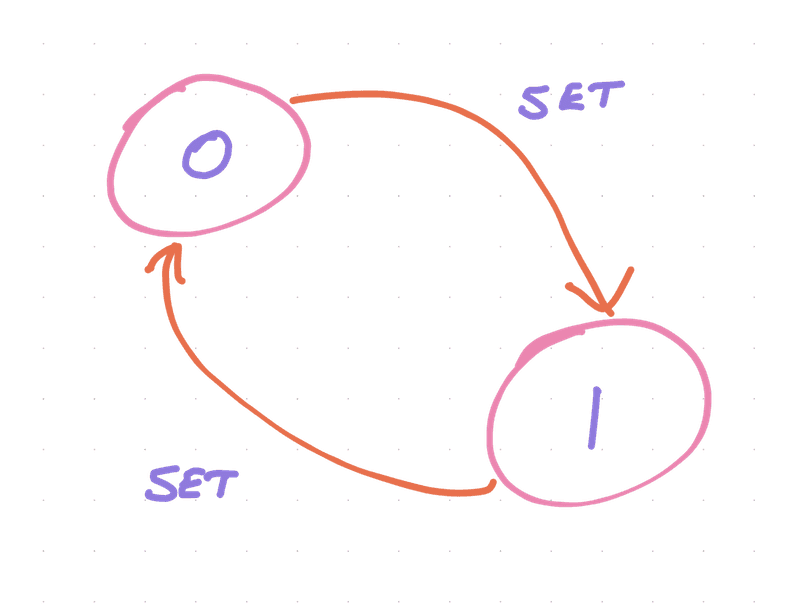
Circles are states, arrows are transitions. You can draw a state machine like this for almost anything[^1].
That's where XState shines – it's designed for state machines. It can even visualize them for you 😍
This is the working state machine for _one screen_ 🤯
— Swizec Teller (@Swizec) July 31, 2020
Thanks @DavidKPiano and team, my brain hurts a little less now. pic.twitter.com/VX8TdH2KIE
Yeah, no wonder that form broke my brain.
Using XState
Now, how do you use XState to fix the mess above?
First you need to define the state machine. Flip your mindset from mapping states to transitioning between states.
I like to do this in the XState visualizer. Helps you see what you're doing.
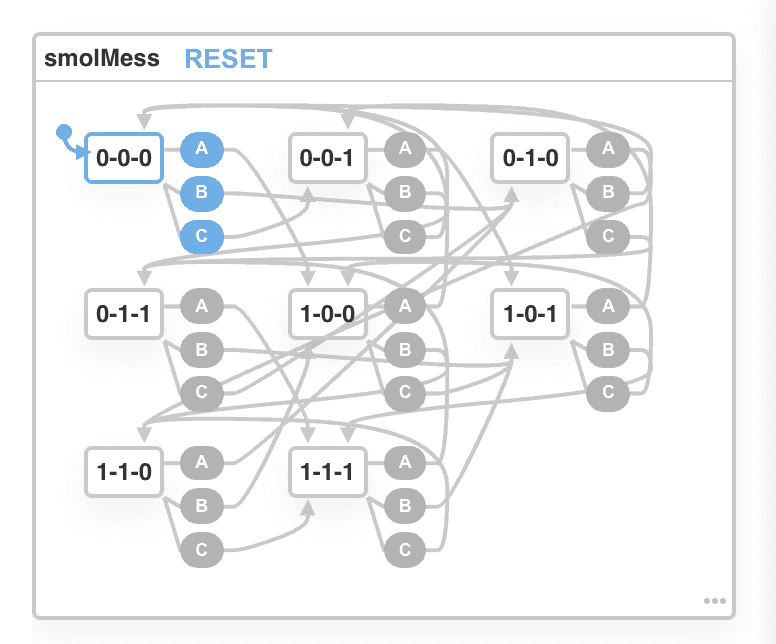
👆 Click to see the full machine in XState visualizer
You define XState machines by listing states, their meta data, and performable actions. Each action points to the next state.
const smolMessMachine = Machine({
id: 'smolMess',
initial: '0-0-0',
states: {
'0-0-0': {
on: {
A: '1-0-0',
B: '0-1-0',
C: '0-0-1'
},
meta: { green: 1, red: 0, blue: 0 }
},
'0-0-1': {
on: {
A: '1-0-1',
B: '0-1-1',
C: '0-0-0'
},
meta: { green: 1, red: 0, blue: 0 }
},
This looks like a lot of work compared to a hashmap, I know, but it's invaluable when you want to prevent some transitions. Or you need to run side-effects when states are entered/exited.
XState can do all that, it's great.
Putting this in our smol mess, you get this:
const [state, send] = useMachine(smolMessMachine)
Hooks the machine up to your component. state
is the current state machine state, send
is like dispatch – sends actions.
Checkboxes use send
instead of setX
<label>
<input type="checkbox" checked={a} onChange={() => send("A")} />A
</label>
And you read current state like this:
// 0-0-0 👉 [false, false, false]
const [a, b, c] = state.value.split("-").map(Number).map(Boolean)
const showGreen = !!state.meta[`smolMess.${state.value}`].green,
showRed = !!state.meta[`smolMess.${state.value}`].red,
showBlue = !!state.meta[`smolMess.${state.value}`].blue
Your code would have better-named states than my example. No need to translate with .map :)
The meta data nests under your state machine name because you can have multiple running in parallel. That's when XState becomes pure wow 😉
And unless I made a mistake, all 3 codesandboxes should behave the same. But 2 of those are way easier to debug ✌️
Happy hacking
Cheers,
~Swizec
[^1] finite state machines can't solve every solvable problem. Pushdown automata (state machines with a stack) are more powerful and Turing machines (state machine with an infinite memory) can solve anything
PS: you can use XState in server code, too
Continue reading about When your brain is breaking, try XState
Semantically similar articles hand-picked by GPT-4
- When your brain is breaking, try Stately.ai
- A new VSCode extension makes state machines shine on a team
- Refactoring a useReducer to XState, pt1 – CodeWithSwiz 11
- Reader question: useReducer or XState?
- Why null checks are bad
Learned something new? Want to become a React expert?
Learning from tutorials is easy. You follow some steps, learn a smol lesson, and feel like you got dis 💪
Then comes the interview, a real world problem, or a question from the boss. Your mind goes blank. Shit, how does this work again ...
Happens to everyone. Building is harder than recognizing and the real world is a mess! Nothing fits in neat little boxes you learned about in tutorials.
That's where my emails come in – lessons from experience. Building production software :)
Leave your email and get the React email Series - a series of curated essays on building with React. Borne from experience, seasoned with over 12+ years of hands-on practice building the engineering side of growing SaaS companies.
Get Curated React Essays
Get a series of curated essays on React. Lessons and insights from building software for production. No bullshit.
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️