How many forms have you built? 1? 2? 5 bazillion?
Lemme guess, every project involves 1 or 2 forms and they're all the friggen same. You got forms coming out the wazoo.
Render fields. Connect to state. Add field validation. Detect submit. Add form validation. Send fetch request. Repeat.
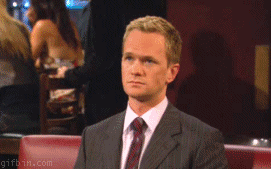
How many times can you go through that mind-numbing software bureaucracy before you say "Enough already!"
And no, that doesn't mean "Give it to the most junior engineer on the team coz they still think it's exciting" either. That's shitty. (and yes every company does it)
Build a form generator
Here's what you do instead my friend, you notice a pattern and you solve it once and for all. For every future project. For every engineer on the team. For yourself.
That's how you level up.
"How do I level up as a developer?"
— Swizec Teller (@Swizec) April 17, 2020
See common patterns at your day job? That crap everyone writes over and over again. Always the same, bored out of their mind?
Turn those into a tool.
Next time you're building a form, grab some extra time, turn it into a library.
Sure, libraries like formik and react-form and redux-form exist. There's a bunch for every frontend framework.
And they're not what I'm talking about.
What you need is a form generator. A piece of code that takes JSON configuration and spits out a form.
A form that looks like your forms. Follows your design system, has your validation principles, looks like you built it.
Form generators vs. forms
Take a basic form for example. Name and email.
Handles 2 input fields, validates email as you type, verifies both fields are filled out before submitting.
58 lines of boring code and there's a bunch of cases I didn't cover.
const Form = () => {
const [name, setName] = useState("")
const [email, setEmail] = useState("")
const [emailValidation, setEmailValidation] = useState("")
const [formValidation, setFormValidation] = useState("")
function changeName(event) {
setName(event.target.value)
setFormValidation("")
}
function changeEmail(event) {
const email = event.target.value
setEmail(email)
if (!isValidEmail(email)) {
setEmailValidation("Not valid email")
} else {
setEmailValidation("")
}
setFormValidation("")
}
function onSubmit(event) {
event.preventDefault()
if (isValidEmail(email) && name.length > 0) {
// submit
setFormValidation("")
} else {
setFormValidation("Missing fields")
}
}
return (
<form onSubmit={onSubmit}>
<label>
Name <input type="text" value={name} onChange={changeName} />
</label>
<br />
<label>
Email <input type="email" value={email} onChange={changeEmail} />
</label>
<p>{emailValidation}</p>
<p>{formValidation}</p>
<button type="submit">Submit</button>
</form>
)
}
Every engineer on your team goes through that every time they build a form. And they build a lot.
This is how they feel 👇
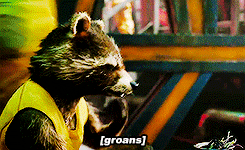
Wouldn't you?
Here's what you do after someone ;) builds a form generator for your team:
const fields = [
{name: 'name', label: 'Name', validate: val => val.length > 0},
{name: 'email', label: 'Email', validate: isValidEmail}
]
// ...
<Form fields={fields} onSubmit={onSubmit} />
😍
How you build a form generator
A form generator doesn't have to be complicated my friend, it just needs to get the job done.
You'll need 3 parts:
- A generic
<Field>
component - A generic
<Form>
component - Couple of loops
Here's a basic example that supports text fields only.
You start with a <Field>
component like this.
const Field = ({ name, label, validate }) => {
const [value, setValue] = useState("")
const [validation, setValidation] = useState("")
function changeValue(event) {
const val = event.target.value
setValue(val)
if (validate(val)) {
setValidation("")
} else {
setValidation(`Invalid ${label}`)
}
}
return (
<>
<label>
{label} <input type="text" value={value} onChange={changeValue} />
</label>
<p>{validation}</p>
</>
)
}
This component handles a complete field. Renders an input with a label, stores state, and performs validation as you type. Even renders errors.
You can use render props for more flexibility. But that's often too flexible. Aim to build a generator that spits out perfect forms for your company. Every time.
The <Form />
component is a glorified loop.
const Form = ({ fields }) => {
const [formValidation, setFormValidation] = useState("")
function onSubmit(event) {
event.preventDefault()
// ..
}
return (
<form onSubmit={onSubmit}>
{fields.map((field) => (
<>
<Field {...field} key={field.name} />
<br />
</>
))}
<p>{formValidation}</p>
<button type="submit">Submit</button>
</form>
)
}
Loop through fields, render each one. Add a submit button, <form>
element, and a place to put errors.
onSubmit
The onSubmit
method handles submissions. Validates all fields, runs any form-level validations, and does the thing.
Something like this:
function onSubmit(event) {
event.preventDefault()
const values = fields
.map((field) => ({
...field,
value: document.getElementsByName(field.name)[0].value,
}))
.filter((field) => field.validate(field.value))
.map((field) => ({ name: field.name, value: field.value }))
if (values.length === fields.length) {
alert(JSON.stringify(values))
setFormValidation("")
} else {
setFormValidation("missing fields")
}
}
This one iterates through the fields and grabs their values straight from the DOM. A little dirty but it works ✌️
You might want to find a way to communicate values from <Field />
components with callbacks or something. Maybe hoist state up a level or add a React context ... depends what you need.
Invalid fields are thrown out and the rest collected into an array of { name, value }
objects. If that array is same length as the number of fields, the form is valid.
My submit alerts. Yours should call the onSubmit
prop function of some sort. Let the user of your form builder define what happens :)
🤯
An afternoon of brain-bending once, a lifetime of perfect forms.
What are you waiting for? Go save your team from this pain, be the hero they need.
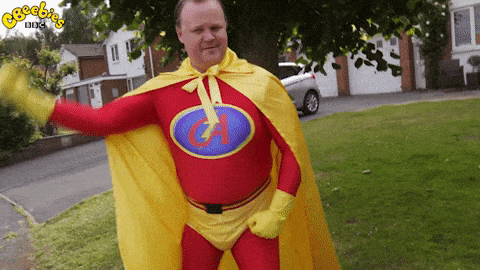
Cheers,
~Swizec
Continue reading about Why you should build a form generator
Semantically similar articles hand-picked by GPT-4
- Why react-hook-form is my new favorite form library
- Delightful state management with hooks and Constate
- Build a new design system in a couple afternoons
- 5 apps with the modern web stack
- How to populate react-redux-form with dynamic default values
Learned something new? Want to become a React expert?
Learning from tutorials is easy. You follow some steps, learn a smol lesson, and feel like you got dis 💪
Then comes the interview, a real world problem, or a question from the boss. Your mind goes blank. Shit, how does this work again ...
Happens to everyone. Building is harder than recognizing and the real world is a mess! Nothing fits in neat little boxes you learned about in tutorials.
That's where my emails come in – lessons from experience. Building production software :)
Leave your email and get the React email Series - a series of curated essays on building with React. Borne from experience, seasoned with over 12+ years of hands-on practice building the engineering side of growing SaaS companies.
Get Curated React Essays
Get a series of curated essays on React. Lessons and insights from building software for production. No bullshit.
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️