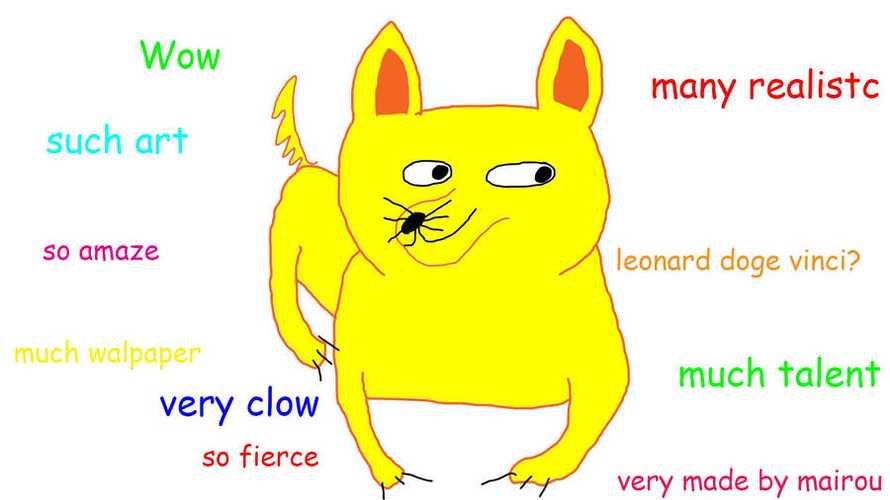
This is a puzzle you can run into if you’re not careful, and sometimes, despite your best efforts, even if you are careful. It happened to me when I had a settings object that I passed into a view. The view then did things. When I made a new view from the settings object, it looked like the view I had just cleared. O.o
Here’s a contrived example:
See the Pen Set - ES6 by Swizec Teller (@swizec) on CodePen.
You have a Counter
class that:
- takes initial state as an argument
- implements the
inc()
method for+1
-ing state - returns current count on every
inc()
call
This allows you to instantiate as many counters as you want, starting from any number you want. They can only count up. The implementation is straightforward. They’re the kind I’ve seen many times in the wild.
You take initial state, save it in an instance property, then proceed to use it like it was your very own. The implementation is simple to reason about, easy to extend when further properties are needed, and quick to build.
Curated JavaScript Essays
Get a series of curated essays on JavaScript. Lessons and insights from building software for production. No bullshit.
There’s just one problem: Instantiating multiple counters from settings stored in memory makes them share state.
Wat? ?
Look, here’s the example again. Pay close attention to the output.
See the Pen Set - ES6 by Swizec Teller (@swizec) on CodePen.
1, 2, 3
, not 1, 2, 1
as you would expect. ?
The rabbit hole goes deeper: Call counter.state == newCounter.state
, and it prints true
. Different objects, same state. Literally the same. Not same value, the exact same memory address.
Strange behavior, but it makes sense once you remember that in JavaScript, objects are always stored as references, not as values. And that sentence is hard to understand if you’ve never had a teacher who forced you to learn C and play with pointers.
You’re about to learn something awesome and core to software engineering!
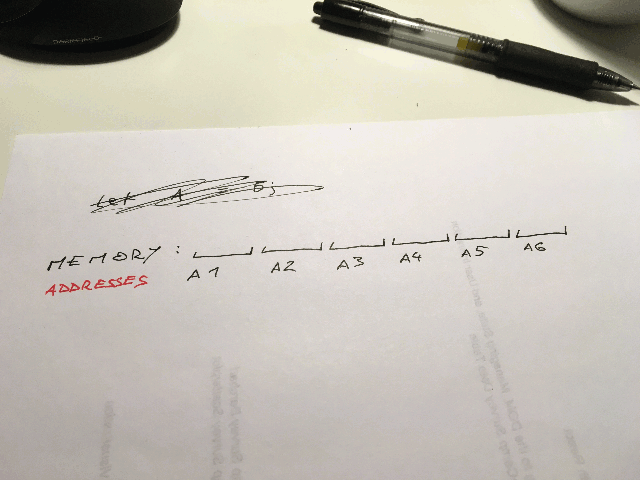
We have memory with 7 addresses - A1
through A7
. For simplicity, we’re giving our variables the same names.
When we set A1
to 5
, a 5
appears at address A1
in memory. Set A2
to 6
and 6
appears at address A2
. Simple values are stored at memory locations as the values.
When we set A3
to an array - [1, 2]
, we need two memory locations to store that value. So we set A3
to the address of our array – A4
. At address A4
we store the value 1
, and put 2
right next to it at A5
.
Now JavaScript can do a memory optimization. When we set A6
to equal A3
, it doesn’t have to create a new array at a new memory address. That would be wasteful. Instead, it gives A6
the same address reference - A4
.
Both A3
and A6
point to the exact same memory location. Change one, and the other changes as well.
Savvy?
To avoid this problem, force JavaScript to make a copy before saving things into local object state. Use Lodash’s _.copyDeep
if there’s a lot to copy, or explicitly set each property to build a new object from scratch.
Err on the side of explicit.
Continue reading about A puzzle in JavaScript objects
Semantically similar articles hand-picked by GPT-4
- A cool JavaScript property you never noticed
- How JavaScript linters cause bugs
- Do you really need immutable data?
- Avoid spooky action at a distance
- React can update state during render
Want to become a JavaScript expert?
Learning from tutorials is great! You follow some steps, learn a smol lesson, and feel like you got this. Then you go into an interview, get a question from the boss, or encounter a new situation and o-oh.
Shit, how does this work again? 😅
That's the problem with tutorials. They're not how the world works. Real software is a mess. A best-effort pile of duct tape and chewing gum. You need deep understanding, not recipes.
Leave your email and get the JavaScript Essays series - a series of curated essays and experiments on modern JavaScript. Lessons learned from practice building production software.
Curated JavaScript Essays
Get a series of curated essays on JavaScript. Lessons and insights from building software for production. No bullshit.
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️