I did it! ?? I figured out a way to reliably interop between modern unidirectional data flow React components and old Backbone views. It ain’t pretty, but it works.
There are three components in that page:
- a
CurrentCount
component that shows current counter state; it’s pure React - a
ButtonWrapper
component that shows counter state and does+1
on click; it’s a wrapped Backbone view - a
ReactButton
component that does+10
on click; it’s also pure React
I know this is a trivial example, but it shows a powerful concept. That +1
button is still the same Backbone view from Tuesday. It stores state in a local Backbone Model, it uses a Handlebars template, and it remains the same idiomatic Backbone View it’s always been. Yet it interops with the React app, blissfully unaware that something fucky’s going on.
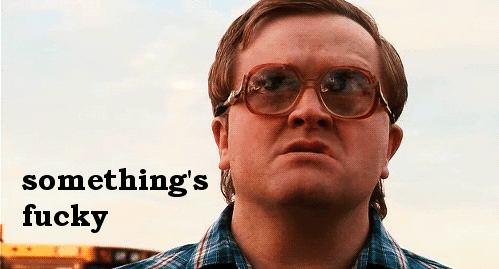
The Backbone view
All three components share the same MobX data store, which has a single observable value called N
. It looks like this:
class CounterStore {
@observable N = 0;
}
In MobX, stores are classes with observable properties. They often have methods and computed values as well, but this example is too simple.
@observable
is a decorator that compiles into something like makeObservable(this.N, 0)
, which in turn uses ES6 to add magical getters and setters that fire up the MobX engine whenever you access – dereference – the observable value. Doing it yourself would look like this:
class CounterStore {
N = 0;
set N(val) {
this.N = val;
// notify all observers that N has changed
}
get N() {
// add call site to list of observers
return N;
}
}
MobX saves you from writing that logic yourself, and it adds a bunch of smartness to make it fast and efficient. I don’t really know how the engine works, but after reading the docs and some of Michel Weststrate’s Medium posts, I’m convinced it’s amaze.
Benchmarked Immutables against Observables in TodoMVC. More details at @ReactAmsterdam conf! #reactjs #redux #mobxjs pic.twitter.com/tL536DWR6f
— Michel Weststrate (threads.net@weststratemichel) (@mweststrate) April 8, 2016
So that’s the store - no boilerplate involved. The two pure React components don’t involve much boilerplate either.
@inject('counterStore')
class ReactButton extends Component {
buttonClicked() {
this.props.counterStore.N += 10;
}
render() {
return (
<div>
<p>React Button:</p>
<button onclick="{action('inc-counter'," this.buttonclicked.bind(this))}="">Jump click count +10</button>
</div>
);
}
};
@inject('counterStore') @observer
class CurrentCount extends Component {
render() {
const { N } = this.props.counterStore;
return (<p>Current count in counterStore: {N}</p>)
}
}
The @inject
decorator takes props from a React context and adds them to a component. I don’t know how MobX-specific this is, but it reduces our boilerplate. Instead of giving each component a store={this.props.store}
type of prop, we can wrap the whole thing in a <Provider>
and give everyone access.
At the end of the day, you always realize that all your components need access to your application state. Global singletons for things everyone needs make life easier. Trust me.
The @observer
decorator comes from MobX’s React bindings. It automagically makes the render()
method listen to store changes, but only those changes that it uses.
This is key. It’s what makes MobX fast. It’s also what leads to confusion when you’re doing things that are not idiomatic React, like inserting Backbone views into React components.
This is weird. If I add console.log to the render() method, MobX works as expected. Take it out, and observer isn't updating.@mweststrate?
— Swizec Teller (@Swizec) September 21, 2016
Thanks to Michel Weststrate for helping me out. His tip about using autorun
saved the day ??
And with that out of the way, I had a Backbone view wrapped in a React component, including full data interop. Change local Backbone state, and the global data store finds out, changes the data store, and the Backbone view updates.
It looks like this:
@inject('counterStore') @observer
class ButtonWrapper extends Component {
constructor(props) {
super(props);
this._init();
autorun(this._render.bind(this));
}
_init() {
this.button = new BackboneButton({
N: this.props.counterStore.N
});
this.button.model.on('change:N', action('inc-counter', (_, N) => {
this.props.counterStore.N = N;
}));
}
componentDidUpdate() { this._render(); }
componentDidMount() { this._render(); }
_render() {
this._cleanup();
this._init();
this.button.setElement(this.refs.anchor).render();
}
componentWillUnmount() { this._cleanup(); }
_cleanup() {
this.button.undelegateEvents();
}
render() {
return (
<div>
<p>Backbone Button:</p>
<div class="button-anchor" ref="anchor">
</div>
);
}
}
</div>
Like I said, it ain’t pretty ?
It works like this:
-
React renders an anchor
div
-
on component mount or update, it runs Backbone view rendering in
_render
-
on component unmount, it cleans up Backbone’s DOM event listeners
-
inside
_render
it:
-
cleans up Backbone DOM event listeners
-
instantiates a new Backbone view with
_init
-
tells the view to render inside that anchor
<div>
-
when initializing the view in
_init
it:
- creates a new
BackboneButton
instance and gives it the value ofN
from our store - listens for changes to
N
on the view’s internal state and communicates them upstream with a MobX action
This approach is a leaky abstraction. The _init
method has to know intimate details about the Backbone view you’re wrapping. There’s no way to get around that because MobX needs those getters and setters to observe state changes.
As soon as you pass a value, MobX loses track. I tried passing the whole store into a Backbone view and using it directly as a model, but that didn’t work. Backbone Model’s set()
and get()
methods circumvent native getters and setters, which means MobX can’t track changes or uses.
Another issue is that because our Backbone views aren’t pure, the UI could sometimes look wrong to the user. It won’t be stale, but it won’t show all side-effects from user actions either.
But we can deal with that later. The important part is that we have a way forward! A way to go from Backbone to React without resorting to a full complete rewrite of everything from scratch. \o/
Continue reading about Backbone → React: Handling state with MobX
Semantically similar articles hand-picked by GPT-4
- Backbone → React: it's a people problem after all ?
- Backbone → React – Step 1
- How to structure your MobX app for the real world
- Using HOCs to DRY up your code
- Livecoding #25: Adding MobX to a vanilla React project
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️