Firebase continues to be 💩. No wonder people keep asking for support in useAuth.
If you do, I’ll switch to useAuth @nozzleio
— Tanner Linsley (@tannerlinsley) January 12, 2021
CodeWithSwiz is a weekly live show. Like a podcast with video and fun hacking. Focused on experiments and open source. Join live Mondays
Good progress this week! We got a Firebase UI login dialog to show up 🥳
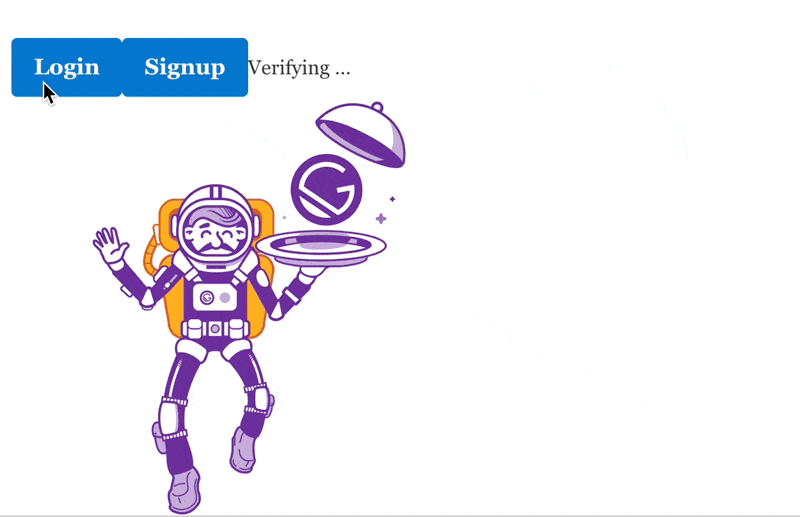
To be honest, I was expecting more from a module that adds 64kB[^1] of JavaScript. Guess styles come separately?
Where we at
Signup flow ends with an error. Return false
from a callback or provide a URL. Documentation mentions both the URL and the callback somewhat off-hand. Hard to find.
An issue from 2016 mentions the URL is required. 5 years later documentation and error message say it isn't. 🥲
signInSuccessWithAuthResult: function(authResult, redirectUrl) {
// User successfully signed in.
// Return type determines whether we continue the redirect automatically
// or whether we leave that to developer to handle.
return true;
},
We do get an earlier error about our callback config. 🤔
On the bright side, a user shows up in Firebase. That means login works and useAuth isn't resolving it correctly.
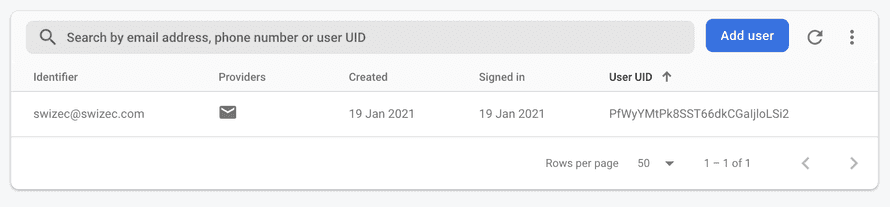
Huzzah!
How we got here
In part 1 of Firebase Auth we sketched out a new provider for useAuth. Configured imports, initialized FirebaseUI, set up the provider class.
This episode we:
- Improved imports
- Figured out how to initialize Firebase
- Discovered the
onAuthStateChange
observer - Got frustrated at Google's SEO where any error message you google points to Firebase docs that do not mention that error message
- Fiddled with TypeScript types
- Fired off the login flow
Improved imports
Here's the correct way to import Firebase and the Auth UI.
import { auth as FirebaseAuthUI } from "firebaseui";
import Firebase from "firebase/app";
import "firebase/auth";
Firebase is a library from the before times and likes to modify globals. In the name of "making it easy".
You import firebase/auth
into nothing. Refer to it through the Firebase
object.
Just like we used to do in 2010 when "JavaScript Bundler" meant "Joe with a pickaxe crafting a giant file with <script>
tags in exactly the right sequence"
Correctly initialize Firebase
We found the incantantion to init Firebase. You must create an app. A project is not enough.
Then you click the app and find a firebaseConfig
object.
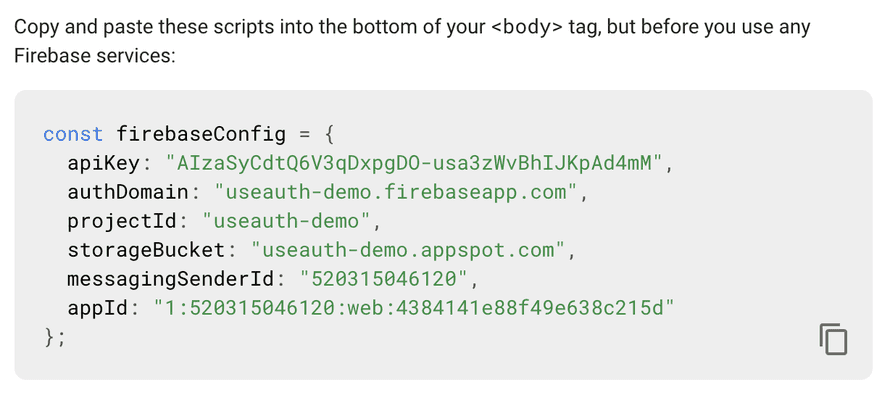
Don't worry about stealing those secrets, they're for the demo app. I intend to share them with the quick start guide.
Use them in the auth provider constructor:
// src/providers/FirebaseUI.ts
export class FirebaseUI implements AuthProviderClass {
// ..
constructor(params: AuthOptions & FirebaseOptions) {
// ..
this.firebase = Firebase.initializeApp(
firebaseConfig,
"useAuth"
);
Obvious in retrospect. Surprisingly hard to find.
The 2nd argument – "useAuth"
– tells Firebase to initialize this as a separate app. Avoid overwriting any global Firebase config you might have.
Final API will have to let you choose. 🤔
onAuthStateChange
observer
onAuthStateChange
was a great find! Lets you hook into the authentication flow and update your state machine.
// src/providers/FirebaseUI.ts
this.firebase.auth().onAuthStateChanged(this.onAuthStateChanged);
private onAuthStateChanged(user: Firebase.User) {
console.log("HAI", user);
}
Start in exploration mode 👉 print current user when state changes. First you get a null
, then you go through the signup flow and get ... something.
Wow when Firebase returns the authenticated user in a callback ... I guess *some* of those are user properties 🤨 pic.twitter.com/0jtGUgjrGI
— Swizec Teller (@Swizec) January 19, 2021
Minified private TypeScript fields, I'm told. You call .toJSON()
to get the real value.
Reload the page after signup and callback prints this again. Login works 🤘
We'll use it next time to update useAuth's understanding of current user.
Fire off the login flow
To fire off the login flow you need to pass all the config. Bit weird but sure.
public authorize() {
// Open login dialog
this.dispatch("LOGIN");
this.ui.start("#firebaseui-auth-container", {
signInOptions: this.signInOptions,
signInFlow: "popup",
signInSuccessWithAuthResult: function(
authResult: any,
redirectUrl: string
) {
console.log({ authResult, redirectUrl });
this.dispatch("AUTHENTICATED", {
user: this.firebase.auth().currentUser,
authResult
});
return false;
}
});
}
useAuth calls this method when user clicks the login button. We put XState into the logging-in state with dispatch('LOGIN')
then fire up the UI.
Full options listed here, if you scroll down.
On success, we print the auth result and current user. Tell useAuth and it handles the UI. Redirecting back to destination or at least re-render.
But it never gets there 🤔
Thank you
Aaaaand I figured out what's wrong! You have to wrap signInSuccessWithAuthResult
in a callbacks:{}
object. Because of course you do.
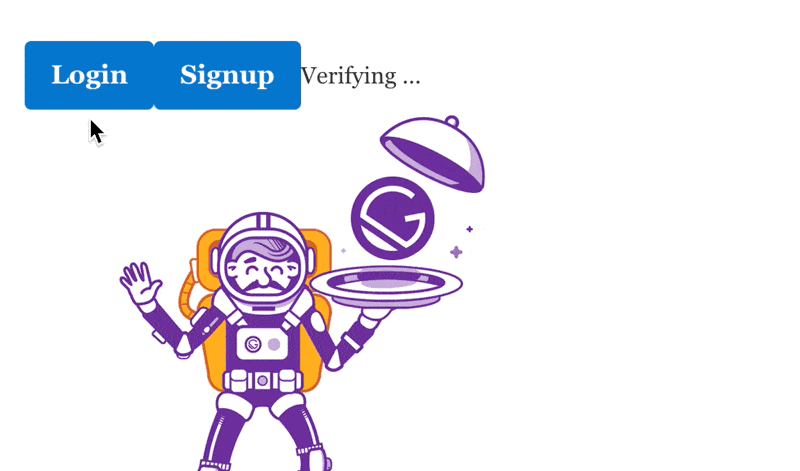
You're a great rubber ducky my friend.
Cheers,
~Swizec
[^1] size difference measured by removing imports, deploying to Vercel, and looking at network tab.
Continue reading about [CodeWithSwiz 20] Adding Firebase support to useAuth, pt2
Semantically similar articles hand-picked by GPT-4
- [CodeWithSwiz 21] useAuth beta support for Firebase 🎉
- [CodeWithSwiz 19] Firebase Auth support in useAuth, pt1
- useAuth – the simplest way to add authentication to your React app
- React context without context, using XState – CodeWithSwiz 14, 15
- useReducer + useContext for easy global state without libraries
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️