Codereviewer: "Why is this binding necessary?"
<Modal show={viewStore.modal.show}
closeOnOuterClick={true}
onClose={viewStore.closeModal.bind(viewStore)}
This code follows my Simple MobX-driven modals approach.
Swiz: "Without binding, the callback would bind to Modal object when called, if I'm not mistaken."
Codereviewer: "Would it? That doesn't seem very intuitive."
Swiz: "Welcome to JavaScript's dynamic scoping. Where have you been the past few years?…
But you're right, it does seem to work. I think MobX's @action
does a bind for us. It definitely wouldn't work with vanilla functions. I'll change the code code."
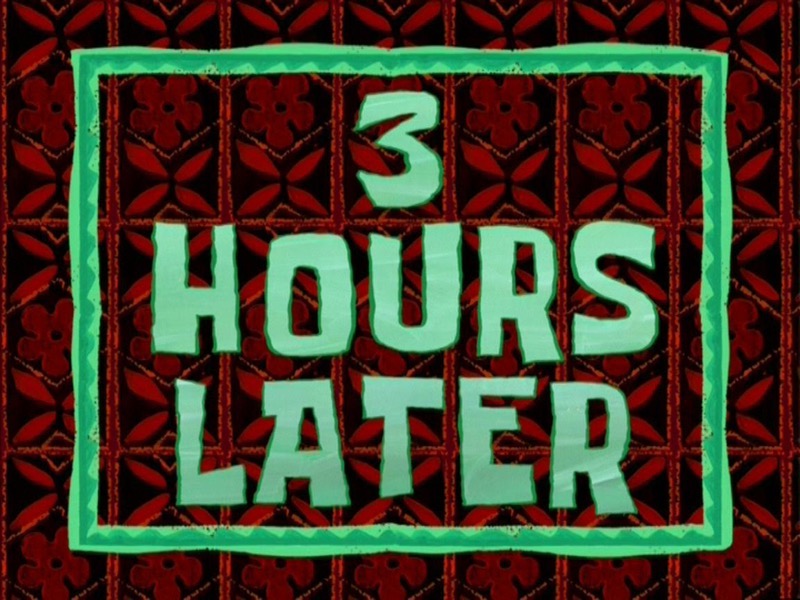
Nope, no, nah. I should not have done that. That did not work. @action
does not do any binding. Wrapping a function in a function does not create a closure with the correct local scope.
But it did work in that one case I tried. Just not in the other. ?
Let's investigate.
Here's a self-contained example in Codepen - a clicker.
See the Pen React, mobx, bind example by Swizec Teller (@swizec) on CodePen.
We have a Store
that holds the number of clicks
and contains an inc
action to increase the count.
class Store {
@observable clicks = 0
@action inc() {
this.clicks += 1
}
}
A not-very-smart functional stateless component renders the current count and a link to click. We use onClick
with a bounded action to detect clicks.
const Clicky = observer(({ store }) => (
<div>
Clicks: {store.clicks}
<br>
<a href="#" onclick={store.inc.bind(store)}>+1</a>
</div>
));
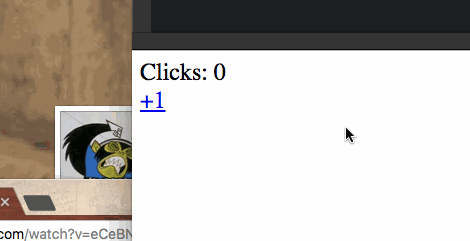
Click the link, number goes up. 14 lines of actual code.
Ok, we know it works with a .bind
. Will it work without one?
See the Pen React, mobx, bind example, pt2 by Swizec Teller (@swizec) on CodePen.
We expanded our Clicky
component with a link that uses an unbounded store.inc
action call as the onClick
callback.
const Clicky = observer(({ store }) => (
<div>
Clicks: {store.clicks}
<br>
<a href="#" onclick={store.inc.bind(store)}>+1 bound</a>
<br>
<a href="#" onclick={store.inc}>+1 unbound</a>
</div>
));
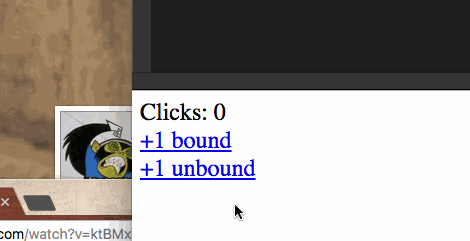
Well, that didn't work. ?
The error reads Uncaught TypeError: Cannot read property 'clicks' of undefined
. Looks like this
isn't defined inside our callback.
Good ol' dynamic scoping, unintuitive as always. If that Wikipedia article doesn't tell you much, don't worry. It's basically comp sci soup.
The gist of their 800-word explanation is this: with dynamic scoping, functions are scoped to where they're called, not where they're defined. It doesn't matter that inc
is defined inside Store
; it matters how React calls it.
So how does React call our click callback? Let's see.
A spelunk through a React event
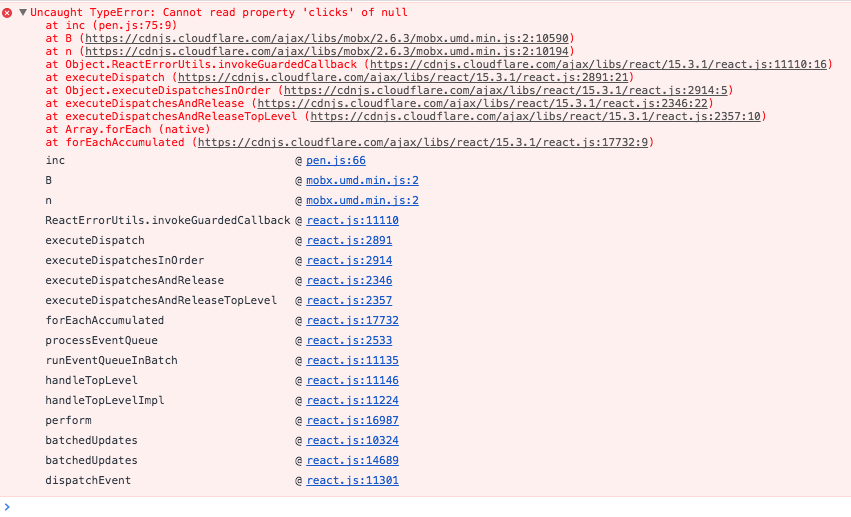
It starts with dispatchEvent
.
dispatchEvent: function (topLevelType, nativeEvent) {
if (!ReactEventListener._enabled) {
return;
}
var bookKeeping = TopLevelCallbackBookKeeping.getPooled(topLevelType, nativeEvent);
try {
// Event queue being processed in the same cycle allows
// `preventDefault`.
ReactUpdates.batchedUpdates(handleTopLevelImpl, bookKeeping);
} finally {
TopLevelCallbackBookKeeping.release(bookKeeping);
}
}
Looks like a function that takes native events from the browser and emits them into React's engine in batches. That's good for performance, great for debugging, and makes everyone's lives easier.
Our next step in the chain is batchedUpdates
.
var transaction = new ReactDefaultBatchingStrategyTransaction()
var ReactDefaultBatchingStrategy = {
isBatchingUpdates: false,
/**
* Call the provided function in a context within which calls to `setState`
* and friends are batched such that components aren't updated unnecessarily.
*/
batchedUpdates: function (callback, a, b, c, d, e) {
var alreadyBatchingUpdates = ReactDefaultBatchingStrategy.isBatchingUpdates
ReactDefaultBatchingStrategy.isBatchingUpdates = true
// The code is written this way to avoid extra allocations
if (alreadyBatchingUpdates) {
callback(a, b, c, d, e)
} else {
transaction.perform(callback, null, a, b, c, d, e)
}
},
}
Told ya it was good for performance. Just look at that comment – "components aren't updated unnecessarily".
I have no idea how this thing works. It smells like some sort of currying.
But we know our next step, eventually, is transaction.perform
.
perform: function (method, scope, a, b, c, d, e, f) {
!!this.isInTransaction() ? "development" !== 'production' ? invariant(false, 'Transaction.perform(...): Cannot initialize a transaction when there is already an outstanding transaction.') : _prodInvariant('27') : void 0;
var errorThrown;
var ret;
try {
this._isInTransaction = true;
// Catching errors makes debugging more difficult, so we start with
// errorThrown set to true before setting it to false after calling
// close -- if it's still set to true in the finally block, it means
// one of these calls threw.
errorThrown = true;
this.initializeAll(0);
ret = method.call(scope, a, b, c, d, e, f);
errorThrown = false;
} finally {
try {
if (errorThrown) {
// If `method` throws, prefer to show that stack trace over any thrown
// by invoking `closeAll`.
try {
this.closeAll(0);
} catch (err) {}
} else {
// Since `method` didn't throw, we don't want to silence the exception
// here.
this.closeAll(0);
}
} finally {
this._isInTransaction = false;
}
}
return ret;
},
?
That's a lot of code. Let's focus on the bits that matter:
perform: function (method, scope, a, b, c, d, e, f) {
// ...
ret = method.call(scope, a, b, c, d, e, f);
// ...
A-ha! The second argument is scope
. React specifically set that to null
when calling transaction.perform
, and function.call
is a JavaScript way to define a function's scope at point of invocation.
The
call()
method calls a function with a giventhis
value and arguments provided individually.
I don't know why React goes out of its way to set callback scope to null
, but I'm sure it has something to do with correctness. Better to throw an explicit error and tell the engineer to fix their code than to get the wrong this
by accident and fuck shit up.
And I'm not sure when, if ever, that scope
argument would be something other than null
.
¯_(ツ)_/¯
What did we learn
Bind your callbacks.
Add a .bind
or a fat arrow wrap -> () => callback()
. Both work.
Continue reading about Do you even need that bind?
Semantically similar articles hand-picked by GPT-4
- Simple MobX-driven modals
- How to structure your MobX app for the real world
- Backbone → React: Handling state with MobX
- Backbone → React: it's a people problem after all ?
- Livecoding #25: Adding MobX to a vanilla React project
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️