Say you want to lazy load some images on your website. You don't want them to just pop into existence and scare the user. A nice fade in effect works much better.
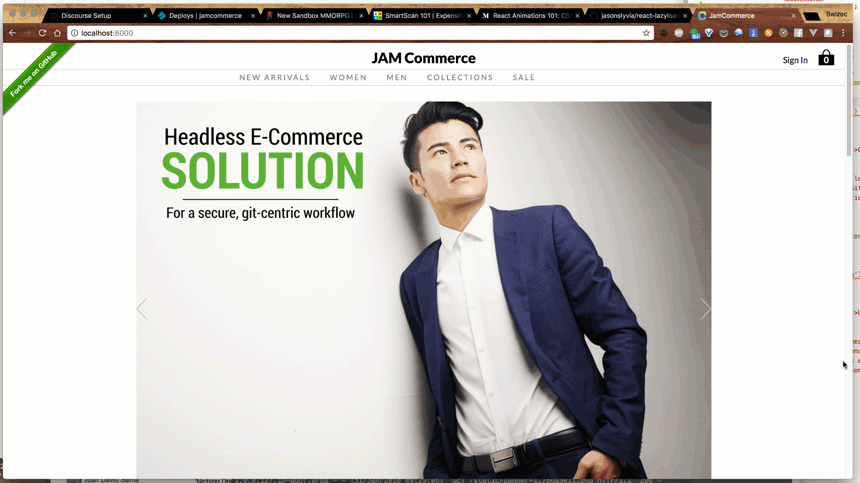
Here's the problem: There's no good pre-built React component for this. Or I suck at finding it.
There's a react-lazyload, which does the lazy loading part for you. It keeps components from mounting into the DOM until the user actually sees them.
This helps make your website faster to load. No need to load large images until a user can actually see them.
But the default experience can be jarring. When images finally load, they just pop into view.
react-lazyload
offers a fade-in example, but it's outdated and doesn't work with modern libraries. And it's cumbersome to use.
So I built a general FadeIn
component. I'll opensource it, but it needs some polish first, and I'd like your opinions on how to make it better.
FadeIn component
The component is just 40 lines of code. Pretty simple.
class FadeIn extends React.Component {
state = {
loaded: false,
}
onLoad = () => this.setState({ loaded: true })
render() {
const { height, children } = this.props,
{ loaded } = this.state
return (
<Lazyload height={height} offset={150}>
<transition in={loaded} timeout={duration}>
{(state) => (
<div
style={{
...defaultstyle,
...transitionstyles[state],
}}
>
{children(this.onLoad)}
</div>
)}
</transition>
</Lazyload>
)
}
}
FadeIn.propTypes = {
height: PropTypes.number,
children: PropTypes.func,
}
FadeIn
keeps local loaded
state to keep track of what to show. false
means "stay transparent", true
means "fade to full opacity".
It uses react-lazyload's LazyLoad
component to handle the lazy loading part, and Transition
from react-transition-group to drive the CSS transition for fading in. This is the part that's changed a lot since the official fadein lazyload example.
Using the children render function approach, you can ask FadeIn
to render anything you want. It gets wrapped in a div that handles the fade effect.
All you have to do is trigger the onLoad
callback once your content is ready. When your image is done loading for example.
<FadeIn height={400}>
{onLoad =>
<img class={cx('img')} src={subheader2} onload={onLoad}>
}
</FadeIn>
You render <FadeIn>
, give it a height so things don't jump around, and pass a children function that takes an onLoad
callback. When you're ready to trigger the transition, you call onLoad
and FadeIn
does its thing.
Did you know onLoad
was a built-in DOM callback that all browsers support? I had no idea.
onLoad
is important because without it the FadeIn transition might end before the image has even come down the pipe. That's the issue I was facing at first when I livecoded this.
Recommendations?
I want to opensource this. I know it's simple, but it could save people like an hour of their time. That's worth it, right?
What else does it need to do? What should I name it? Would you use it?
Continue reading about Fade in lazy loaded images with React and CSS – a quick guide
Semantically similar articles hand-picked by GPT-4
- What I learned making my first open source React component
- Livecoding #18: An abstract React transition component
- Towards a Gatsby+Suspense proof-of-concept
- React 18 and the future of async data
- Async React with NextJS 13
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️