Dude, open source is hard. People really do this every day!? 🤨
I open sourced my react-lazyload-fadein component this weekend. 75 stars already. I guess people like it 💪
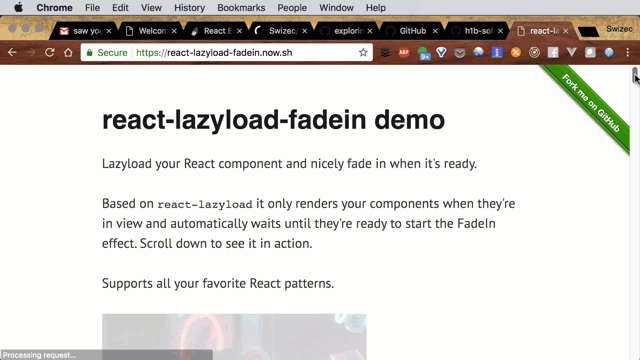
It builds on top of react-lazyload to give you a nice fade-in effect when your component is ready. Lazyload means users load less stuff and your page appears faster because you're not rendering complex expensive components until they're visible. Fade-in means they don't pop onto the page and startle your user.
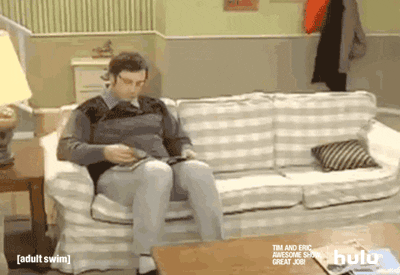
Building the component was pretty quick. Learn the new API for React transitions, realize images have an onLoad
event, package it up in a component. Easy 👌
Then open sourcing it… that took 4 hours. Because open source is hard.
You can see half of the process on YouTube. The other half got banned because of the music.
Here are a few lessons I learned:
Use nwb to get started
Is there a create-react-app for components? I want to make an opensource component but not sure how to start.
— Swizec Teller (@Swizec) February 18, 2018
create-react-component exists but seems way old
Maybe @thekitze @lukeed05 @kentcdodds have suggestions?
When you start a new React app, you use create-react-app
. It sets everything up for you. Babel, webpack, a dev server, some HTML, production builds, all the things.
But you don't need most of that when you're building a component. You wouldn't want to minify before publishing to npmjs, for example. You definitely don't need to add a bunch of HTML and CSS.
I mean, probably. You might need styling.
Point is, you are trying to publish an isolated component. You still need some build step for shipping and some way of running the component when testing. But you don't need CRA.
nwb new react-component react-bla
This sets you up. A src
folder for your code, a demo
folder for your demo app (plus dev server and hot loading, yay\o/), a test
folder that you should use and I didn't, some build stuff for shipping…
Basically, it gives you everything you need so you can focus on your component and don't have to worry about the details.
Make a demo page with docs
You need a demo page so you can show off your component in action. People are visual creatures, and they don't like to read descriptions.
Show them what you built.
react-lazyload-fadein, for example, has a demo page that shows off different ways you can use it. The page is long enough for you to see the fade-in effect.
The demo page doubles as docs. People can scroll to the integration they like and see how to build it right away.
That part took a while because nwb's demo pages are quite basic. No Gatsby machinery to slurp in your README file and make a page.
There's an idea 🤔
Decent docs take time
People will find out about your component in different ways. Some will see it on GitHub, others will come to your demo page, many will see it on npmjs.com.
Your documentation has to live in at least two places:
- Your README.md file
- Your demo page
Both GitHub and npmjs.com give special rights to your README. It shows up as your component's homepage.
So it better be good. Add a gif or two. All the code samples someone needs to get started. Explain why they'd wanna use it.
This duplication is annoying and creates work for you. But it's worth it, I think. Especially if someone could figure out how to slurp in the README file and make that demo page for you 🤔
Support different patterns
The React community is a bit silly and loves to invent new patterns to bicker about. Function as children, render props, import this way or that way.
Your component should support everything that's practical. That makes you a good citizen in other people's codebases.
Someone likes to do import FadeIn from 'react-lazyload-fadein'
? They can.
They prefer import { FadeIn } from 'react-lazyload-fadein'
? They can do that, too.
Wanna use function as children, my favorite? Yep, it works.
<FadeIn>{(onload) => <MyThing onLoad={onload} />}</FadeIn>
Using render props in your codebase? That works too.
<FadeIn render={(onload) => <MyThing onLoad={onload} />} />
You shouldn't have to compromise your code style to use my component. My job isn't to encourage this or that pattern. My job is to give you a tool that you can use to make your life easier.
And you know how hard it is to support both patterns?
render() {
const { children, render } = this.props;
return (
<div>
{children && children(this.onLoad)}
{render && render(this.onLoad)}
</div>
)
}
Yeah, sooooo hard. Just do it.
Do it more often
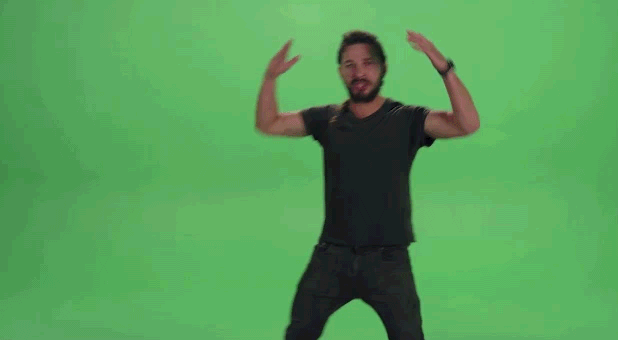
People don't want to learn to fish. People want fish.
Building things and writing about them is great. Great for your skills, great for your career, great for just about everything. Gives you more luck.
Even just tweeting or posting on FB about what you're working on is better than keeping mum and chugging away. Ask anyone.
You know what's even better?
Giving people tools.
You're not just telling them what to do, you're ensuring they have less to do. Everyone loves having less to do.
I should do it more often, too. :)
This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding. Usually shorter than 500 words. Often with pictures. Livecoding happens almost every Sunday at 2pm PDT on multiple channels. You should subscribe to My Youtube channel to catch me live.
Continue reading about What I learned making my first open source React component
Semantically similar articles hand-picked by GPT-4
- Fade in lazy loaded images with React and CSS – a quick guide
- Livecoding #27: New React Indie Bundle page almost done
- Why dataviz does better for your career than TODOapps
- Livecoding #18: An abstract React transition component
- Livecoding #26: A new page for a new React Indie Bundle
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️