I made a terrible thing Sunday night.
The theme that I bought for the new React Indie Bundle page has decorations built with SVG. You slap them into the page as <svg>
tags, and it makes your sections look nice.
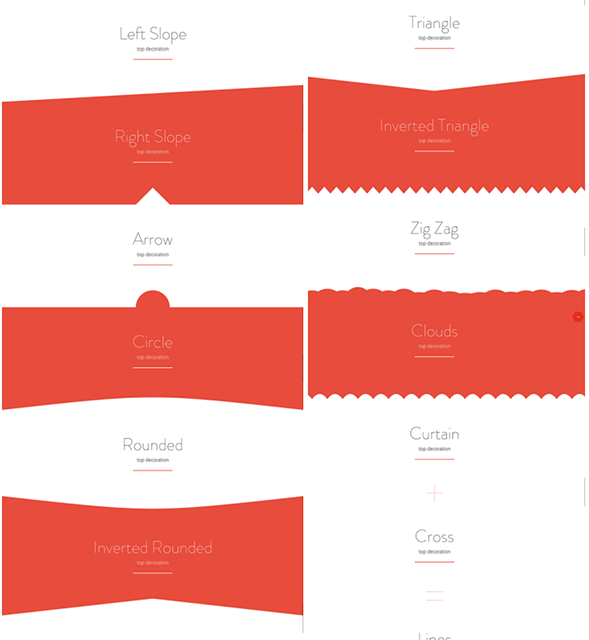
You're supposed to slap them into the page as <svg>
tags. It's great that create-react-app
has built-in support for importing svgs, right?
Well… that's meant for images. import arrow from 'decorations/arrow.svg
gives you a relative URL. Something like public/decorations/arrow.svg
that you're meant to use as a src
prop for an image tag.
import arrow from 'decorations/arrow.svg`
// ...
const Arrow = () => (
<img src={arrow}>
);
This, of course, does not work with with the Angle theme. That one wants you to use SVG as a first-class citizen of the DOM. So what's a guy to do? ?
Well… heh… I built a python script that takes SVG and crudely turns it into a React component. This is a terrible idea, but with a lot of manual massaging, it worked. ?
Here it is:
import os
# from http://stackoverflow.com/questions/4303492/how-can-i-simplify-this-conversion-from-underscore-to-camelcase-in-python
def dash_to_camelcase(value):
def camelcase():
yield str.lower
while True:
yield str.capitalize
c = camelcase()
return "".join(c.next()(x) if x else '-' for x in value.split("-"))
for subdir, dirs, files in os.walk('./top'):
for file in files:
path = os.path.join(subdir, file)
name, ext = file.split('.')
with open(path, 'r') as f:
svg = f.read()
svg = svg.replace('<svg', 'import React from "react"; export const %s = () => (<svg' % dash_to_camelcase(name), 1) \
.replace('class=', 'className=') \
.replace('preserveaspectratio=', 'preserveAspectRatio=') \
.replace('viewbox=', 'viewBox=') \
.replace('stroke-width=', 'strokeWidth=') \
.replace('', ')')
with open(os.path.join(subdir, name+'.js'), 'w') as out:
out.write(svg)
The script traverses a directory and assumes all the files it finds are SVGs. This is the first red flag. Then it reads the SVG as text and performs some search & replace operations:
<svg
becomes a React import, and an exported function declarationclass
,preserveaspectratio
,viewbox
, andstroke-width
are fixed to follow React's prop naming rules</svg>
gets a)
to close the function body.
This is red flag number 2 through 6, maybe 7. Why? Because this is terrible, and I should feel bad. You can't parse XML with Regex, which this is a simplified instance of, and for the love of god use a parser like lxml.
Several problems showed up:
- Some files had two
<svg>
tags. I had to manually edit them every time I re-ran the script because bugs ? - Some files had typos in their props, like
stroke-width = "
. This is valid XML, but my "parser" couldn't handle it ➡ manual edits - Files with two
<svg>
s have to be wrapped in a<div>
to fulfill React's "one child per component" policy. This needs manual edits because my "parser" knows nothing.
2 hours after I started, I was done. Happy to have successfully turned 30 svgs into React components, I could now use the two that I needed. Excellent.
Yak shaving at its finest.
This could make a useful Webpack plugin, though. Import SVG as a React component? That sounds like something everybody needs ?
Continue reading about I made a python script that converts SVG to React ?
Semantically similar articles hand-picked by GPT-4
- This is Yak Shaving
- Build an animated pure SVG dynamic height accordion with React and D3
- Build responsive SVG layouts with react-svg-flexbox
- How to export a large Wordpress site to Markdown
- How to debug unified, rehype, or remark and fix bugs in markdown processing
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️