My first webinar! How I hate the word, but that's what it was.
The plan was to get a bunch of prospective tech interns together on a stream, teach them a React concept, and give them something to build. Sunday night, I'm seeing what they build and figuring out who I want to work with.
The goal?
To judge people's slope vs. y-intercept. Are they fast learners, or do they just know a lot? I want to hire someone who learns fast, not someone who's already a pro. That way I'm working with a future pro and helping them get there.
Imagine y
is your skill and x
is time. Slope always beats out y-intercept in the long run.
a little bit of slope makes up for a lot of y-intercept pic.twitter.com/ZiJc29jyOA
β Swizec Teller (@Swizec) June 14, 2018
What interns learned
We learned about the new React context API and built a silly little app that shows off two benefits of context: 1. Shared state between components 2. No prop drilling
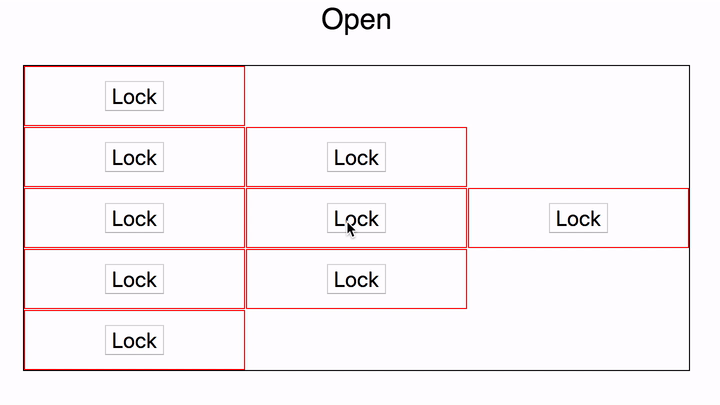
Hereβs the source on GitHub.
We used React.createContext()
to create a context:
const LockContext = React.createContext()
```
And a top level component to hold the state and callback we wanted to share. It's like a Redux or MobX store, you want your state and your callbacks to travel together.
```javascript
class ContextualThing extends React.Component {
state = {
locked: false
};
toggleLock = () =>
this.setState({
locked: !this.state.locked
});
render() {
const { locked } = this.state;
return (
<div>
This is a lock
<p style={{ fontsize: "2em" }}>
{locked ? <b>Locked!</b> : "Open"}
</p>
<lockcontext class="provider" value={{ locked, togglelock: this.togglelock }}>
{[1, 2, 3, 2, 1].map(n => <togglerow n={n}>)}
</togglerow><table></table>
</lockcontext>
</div>
);
}
}
When state and callbacks go hand in hand, they're easier to manage. Helps you think in state machines.
But I forgot to mention the state machine part in the webinar π
Each ToggleRow
renders as many table cells as you ask it to:
const ToggleRow = ({ n }) => (
<row>
{new Array(n).fill(0).map((_) => (
<cell>
<locktoggle></locktoggle>
</cell>
))}
</row>
);
Notice the lack of prop drilling. <ToggleRow>
gets only a number, n
. Nothing about callbacks or the locked state. It doesn't even pass any props into <LockToggle>
.
That's because LockToggle
is a smart context component π
const LockToggle = () => (
<lockcontext class="consumer">
{({ locked, toggleLock }) => (
<button onclick={toggleLock} style={{ fontsize: "1.5em" }}>
{locked ? "Unlock" : "Lock"}
</button>
)}
</lockcontext>
);
LockToggle renders a context consumer, which takes a function as children render prop. This render prop gets the necessary locked
state and toggleLock
callback in its arguments and returns a button that flips the lock.
Et voilΓ : shared lock/toggle between components without prop drilling.
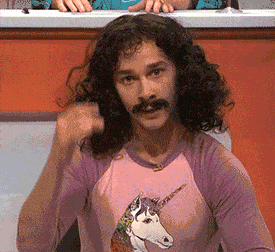
I also answered a lot of questions about when you should use context versus Redux or MobX. Watch the stream π
What Swiz learned
Here's what I got out of the experience in a nutshell 1. Waiting for people to show up is nerve wracking 2. Lightly advertise in advance and people will show up 3. Start the stream on time. no need to be there the first 10 minutes 4. You can wing it if you know your shit 5. Good way to practice conference talks, I think 6. Maybe have some slides 7. Drawing ideas out on paper while explaining them is a great interactive way to produce slides in real time 8. The delay between what you say and what people hear is massive 9. Giving the audience time to ask questions is nerve wracking
What's next
More webinars I think. Great way to develop new content and a low friction approach to video production. That gives me ideas. π€
Plus, answering questions from the audience is a great way to see what you forgot to cover. That's slope right there!
Continue reading about Intern process part 2 β (my first) webinar, about React context
Semantically similar articles hand-picked by GPT-4
- Hire these interns
- Online workshops are MORE interactive than in-person workshops
- Tech intern job
- Reactathon 2018 was a hoot β€οΈ
- The audience loved my talk but I didn't
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below π
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails π on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. π"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help π swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers π ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are β€οΈ