You know that feeling when you meet an old friend you haven't seen in years? You used to be so close but ...
"Long time huh ..."
"Yeah time flies ... how's the wife?"
"Girlfriend. She's good, just started a new job ..."
"Nice nice ... you still at that startup?"
"Yeah ... it's okay ... you?"
"Nah, new gig last year. Think I told you, dunno"
"Maybe you did, hard to keep track ... liking it?"
A beer or two later and you're back to being best buds. Suddenly it's 1am and a long yawn reminds you that you're not quite so young anymore.
Could it be? Am I really old enough to be gently hungover from two beers with @Smotko?
โ Swizec Teller (@Swizec) May 23, 2019
๐คจ
That's what messing around with jQuery felt like after all these years.
I was bored Monday night and decided to see if I still know how to build with jQuery. Nothing better to do, no pressing ideas, a bit of fun.
Also a favor for a friend who's preparing to talk about React to a bunch of people who've never seen it before ๐
It's funny, jQuery is so old now (2 years since new versions stopped coming) I feel like I almost have to introduce the darn thing. Used to be the backbone of the web, now it's in the dustbin of history.
Pushed web development forward for its entire 10 year run. Oh the memories ...
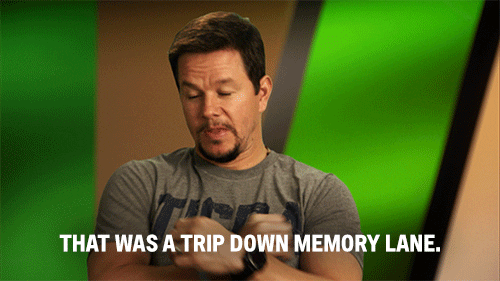
So, how does jQuery stack up to React and how does React stack up to Svelte? Let's see ๐
React app
Started with a React app. It's what I'm most familiar with right now.
We built a cat app. Fetches 5 cats from thecatapi, shows first cat. Click a button, get more cats.
When app runs out of cats, it fetches more.
function fetchCats(currentCount) {
const page = Math.ceil(currentCount / 5);
return fetch(
`https://api.thecatapi.com/v1/images/search?limit=5&page=${page}&order=Desc`
).then(response => response.json());
}
fetchCats
returns a promise with a bunch of cats. Pagination works based on current count of cats we've got.
Rendering a list of cats happens in a <Kitties />
component. 'tis but a loop
function Kitties({ cats, count }) {
return (
<>
{cats.slice(0, count).map(cat => (
<div key={cat.id}>
<img src={cat.url} alt="Cat image" />
</div>
))}
</>
);
}
Take list of cats
, count
how many the user wants to see, slice
the array, render each cat as an image. ๐
Driving this logic with React Hooks isn't bad. React's ergonomics are getting real good.
function App() {
const [showCount, setShowCount] = useState(1);
const [cats, setCats] = useState([]);
useEffect(() => {
if (cats.length < showCount) {
fetchCats(showCount).then(newCats => setCats([...cats, ...newCats]));
}
}, [cats, showCount]);
const moreCats = () = > {
setShowCount(showCount + 1);
};
return (
<div className="App">
<h1 >These are cats.</h1 >
<h2 >Cats are cool </h2>
<Kitties cats={cats} count={showCount} />
<button onClick={moreCats} >More cats! </button >
</div >
);
}
We got useState
for the count of cats, and a useEffect
to deal with fetching. Fetches on first load and makes sure to fetch more when showCount
is bigger than the current amount of cats.
React handles the rest.
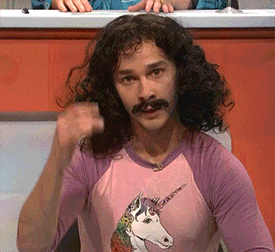
jQuery app
The hardest part of building the jQuery version was figuring out how to import jQuery in a modern compiled-JavaScript app. ๐
No seriously, I struggled, tried a bunch of things, and ended up with a good old script tag and a public CDN.
<script src="https://code.jquery.com/jquery-3.4.1.slim.min.js" integrity="sha256-pasqAKBDmFT4eHoN2ndd6lN370kFiGUFyTiUHWhU7k8="
crossorigin="anonymous"></script>
People don't do that in real life anymore. It's all import this import that.
For the app itself we used a naive unoptimized approach much like many jQuery apps of old ๐ find div, empty div, render everything from scratch every time.
function renderCats(cats, count) {
$("#kitties").html("");
cats.slice(0, count).forEach(cat => {
$("#kitties").append($("<div >").append($("<img>").attr("src", cat.url)));
});
}
Notice the mess with creating a small component tree of <div><img></div>
? Painful. JSX improved this a lot. Almost can't believe we used to build complex apps like this.
You can see it's slow too.
Open the CodeSandbox, add a couple cats. You'll see the more cats there are the slower the app gets.
Not so with React because React avoids re-rendering components that don't change.
PS: check out this mess of attaching a button click handler and driving the cat fetching logic.
$("button").on("click", () => {
CatCount += 1;
if (CatCount > Cats.length) {
// Fetch more cats when we run out
fetchCats(CatCount).then(newCats => {
Cats = [...Cats, ...newCats];
renderCats(Cats, CatCount);
});
} else {
renderCats(Cats, CatCount);
}
});
๐คฎ
I like hooks.
Svelte app
The Svelte version wins in the immense straightforward simplicity of it all. I'm pretty blown away.
Check out the <Kitty />
component
<script>
export let id;
export let url;
</script>
<style >
img {
width: 300px;
}
</style>
<div>
<img src={url} alt="Kitty pic" />
</div>
Gets an id
and a url
, has some style
, and renders a div with an image. Pretty slick, looks almost like pure HTML.
Then you got the component that drives state and renders a bunch of kitties with a button.
<script>
import Kitty from "./Kitty.svelte";
let showCount = 1;
let cats = [];
function fetchCats(currentCount) {
const page = Math.ceil(currentCount / 5);
return fetch(
`https://api.thecatapi.com/v1/images/search?limit=5&page=${page}&order=Desc`
).then(response => response.json());
}
fetchCats(showCount).then(newCats => {
cats = [...cats, ...newCats];
});
function moreCats() {
showCount += 1;
if (showCount> cats.length) {
fetchCats(showCount).then(newCats => {
cats = [...cats, ...newCats];
});
}
}
</script>
The script looks similar to React Hooks. You got some variables with state and you got a moreCats
method that drives it.
Repeating that array merging logic twice is a little less elegant than the useEffect
hook, but probably easier to understand how it works ๐ค
Rendering is neat too
<main>
<h1 >These are cats.</h1 >
<h2>Cats are cool ๐ธ</h2>
{#each cats.slice(0, showCount) as {id, url}}
<Kitty id={id} url={url} />
{/each}
<button on:click={moreCats}>More cats! </button >
</main>
Similar to React, but we used {#each}
instead of JavaScript's .map
. Still not sure how I feel about that but it's pretty readable you gotta admit.
๐ค
Between you and me I think React wins this one. Maybe it's because I'm used to it.
But Svelte does look like it might be quicker to grasp for someone who's new to modern JavaScript.
What do you think?
Happy Friday,
~Swizec
Continue reading about Just for fun ๐ React vs. jQuery vs. Svelte, same ๐ฑ app
Semantically similar articles hand-picked by GPT-4
- Svelte takes the best of React and the best of Vue to make something awesome
- Why dataviz does better for your career than TODOapps
- Livecoding 52: First impressions of Vue
- How to rewrite your app while growing to a $100,000,000 series B
- React components as jQuery plugins
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below ๐
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails ๐ on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. ๐"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help ๐ swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers ๐ ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are โค๏ธ