Making fake demo graphs is always fun. Here you are, making a graph without data, generating random datapoints, trying to make it as realistic as possible.
Two days ago I was failing at the same.
It sounds simple, right? To produce a random graph, you generate random data. Something like this:
var data = _.range(250).map(function () {
return Math.round(Math.random() * 100);
});
And your graph will look like this:
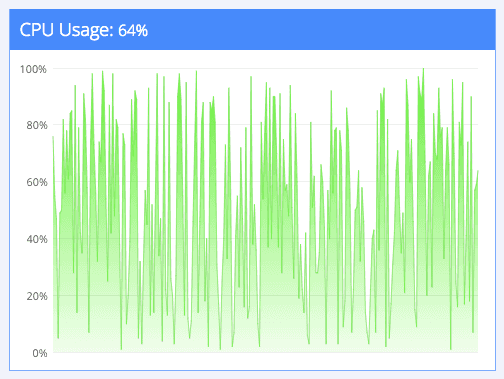
Well, that doesn't look realistic at all. Crazy spikes, deep valleys ... if a real-world system behaved like this you would be very concerned for its wellbeing. This won't do for a demo.
And besides, you wanted a live graph anyway.
So you move data generation into the backend and hook it up with socket.io. Then you make a function that generates one datapoint every 250ms
.
Data generation looks like this:
io.on("connection", function (socket) {
setInterval(function () {
socket.emit("cpu", update());
}, 250);
function update() {
return Math.round(Math.random() * 100);
}
});
Your graph now looks like this:
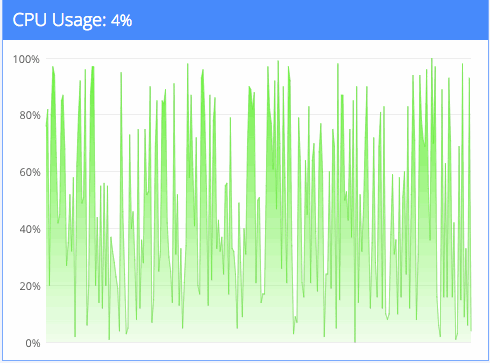
Yay! It moves!
But still too random to look realistic. A CPU that behaves like that is in serious trouble!
What if instead of producing random values, we produced random changes to existing values? That could work. Remember the previous state, make a tiny random change.
Data generation now looks like this:
io.on('connection', function (socket) {
var previous = 0;
setInterval(function () {
previous = update(previous);
socket.emit('cpu', previous);
}, 250);
function (previous) {
previous += Math.random()*10-5;
if (previous > 100) previous = 100;
if (previous < 0) previous = 0;
return Math.round(previous);
}
});
And the graph looks like this:
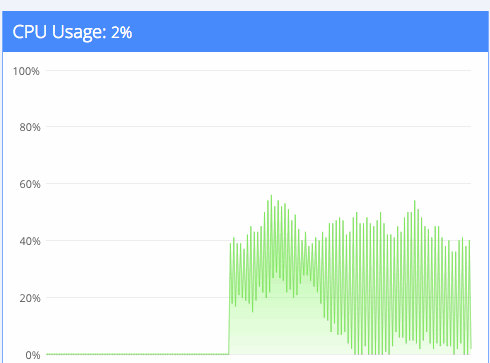
Or like this:
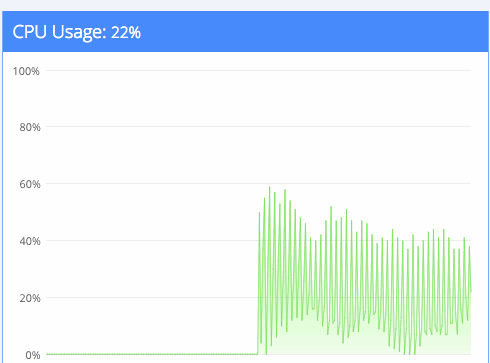
Or even like this:
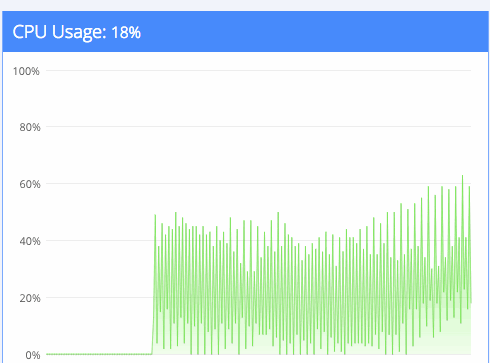
Wait ... what? That's even worse than before! How is this possible, the value changes on each step are tiny?
And I wrecked my brain and wrecked my brain and then wrecked my brain some more. Refreshed the page many times. Rewrote the data generating function many times. Nothing I tried worked. The graph looked more sporadic than ever.
Can you spot the error?
The clue lies in those graphs moving faster and faster with each refresh. Something fucky is going on - the uncleared setInterval.
You see, that backend node.js process is persistent. The 250 millisecond interval doesn't just go away when you refresh the page. Oh no, it's still there, waiting for you, stalking its prey, hunting.
And because socket.io does evertyhing in its power to be more reliable than not, it will reconnect old sockets because hey, you probably just have spotty wi-fi. But we also started a new setInterval
on every connection
event.
Yeah.
That's not a single random function acting funny, that's a whole bunch of them!
If we fix the backend code to look like this:
io.on('connection', function (socket) {
var previous = 0;
var interval = setInterval(function () {
previous = update(previous);
socket.emit('cpu', previous);
}, 250);
function (previous) {
previous += Math.random()*10-5;
if (previous > 100) previous = 100;
if (previous < 0) previous = 0;
return Math.round(previous);
}
socket.on('disconnect', function () {
clearInterval(interval);
});
});
The graph looks like this:
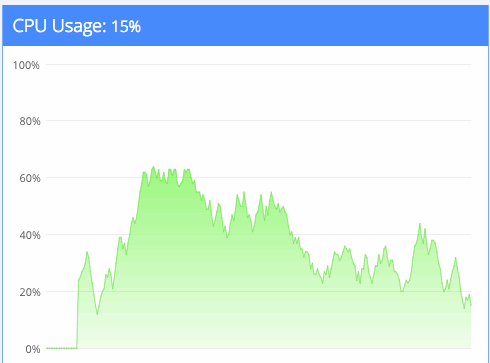
And all is right in the world.
You're happy, your boss is happy, your graph looks like it could be real but isn't. Acting.
Continue reading about Kids, always remember to clear your intervals
Semantically similar articles hand-picked by GPT-4
- Quick scatterplot tutorial for d3.js
- Chrome's console.log is the slowest
- Sexy animated spirographs in 35 sloc of d3.js
- Testing socket.io apps
- Screw web performance, just wait a little 😈
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️