This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding. Shorter than 500 words. With pictures. You can follow my channel here. New content almost every Sunday at 2pm PDT. There’s live chat. ?
We did it! A few thousand elements smoothly animated with React and canvas! Hooray! \o/
It’s not perfect. We had to throw the baby away with the bath water and drop down a layer below React. The updateChildren
issue from last time proved too hard to solve. Too hard for me to solve at least.
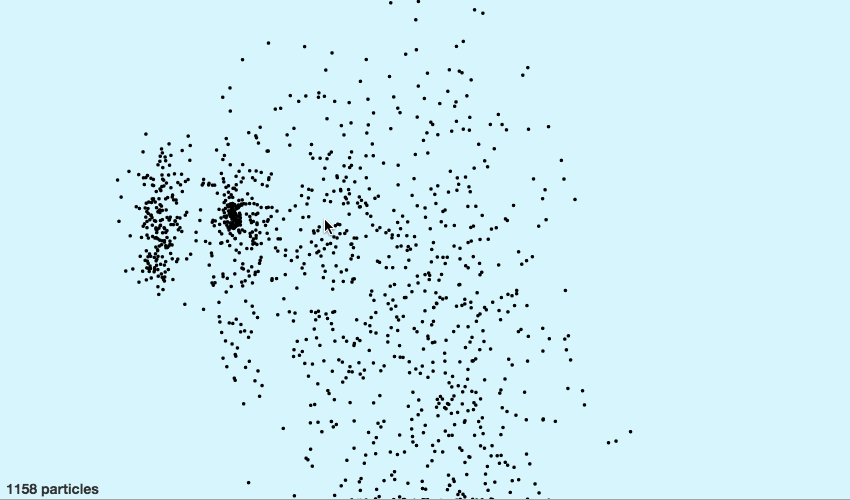
We moved our particle rendering logic into componentDidUpdate
and rolled up our sleeves. Without React’s help, we were forced to manually keep track of new and old particles.
The upside is that we can move existing particles instead of re-creating them. This saves cycles. The downside is that we were unable to remove irrelevant particles efficiently, so they pile up in memory.
It’s a garbage collection problem in a way. Animation works well the first time you try it. But if you wait for all the particles to vanish and try again, it’s super slow. That’s because it keeps redrawing particles that are no longer there. Oops.
The meat of our code looks like this:
componentDidUpdate() {
let layer = this.refs.the_thing,
particles = this.props.particles,
for (let i = 0; i < particles.length; i++) {
let { id, x, y } = particles[i];
if (this._particles[id]) {
// move particle
this._particles[id].position({
x: x,
y: y
});
}else{
// create new particle
let c = new Circle({
radius: 1.8,
x: x,
y: y,
fill: 'black'
});
this._particles[id] = c;
layer.add(c);
}
};
layer.batchDraw();
Lovely. new Circle
creates a particle, .position
updates its position. layer.batchDraw
does drawing stuff with the Konva library.
This is great, but it’s not perfect. When I stress-tested it later, I discovered an issue. At 4,000 particles, the animation becomes sad. At 7,000 particles, it’s disgusting.
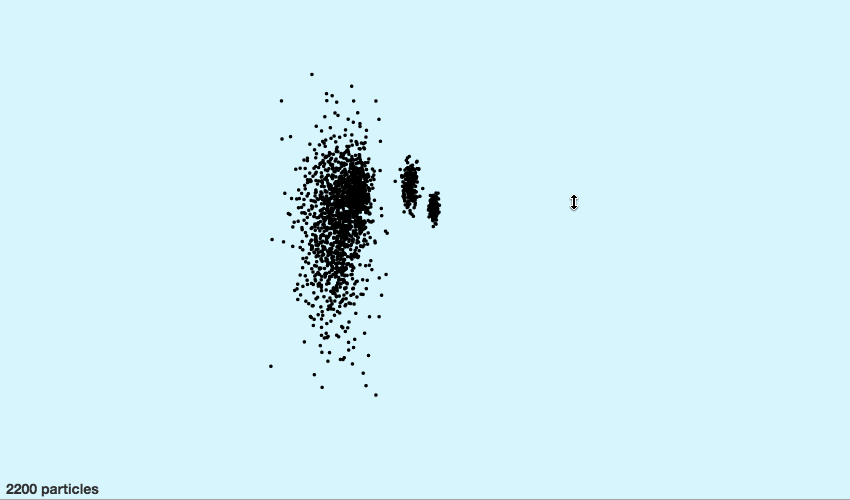
So sad.
Just for shits and giggles, I checked the original pure SVG implementation from a few weeks ago. To my surprise, it works better. Better! React renders and updates pure SVG faster than our hard-earned canvas code does.
It takes 7,000 elements to bog down the pure SVG implementation.
Ugh. So annoying. Back to the drawing board.
There’s a sliver of hope. Take a good look at those gifs.
React+SVG works well up to about 3,000 particles, then quickly bogs down, and has a hard time reaching more than 6,500 particles on screen.
React+canvas+Konva works a bit worse at 3,000 particles, but looks like it’s slowing down slower. If that makes sense. It’s not much worse at 5,000 particles than at 3,000. React+SVG at 5,000 looks terrible compared to 3,000.
This is promising. It implies that canvas can handle it, but we’re doing something stupid. Maybe Konva is slow, or maybe we’re misusing it.
We’ll find out this Sunday :)
PS: the edited and improved versions of these videos are becoming a video course. Readers of the engineer package of React+d3js ES6 get the video course for free when it’s ready.
Continue reading about Livecoding #14: Mostly-smooth animation up to 4,000 elements with React and canvas
Semantically similar articles hand-picked by GPT-4
- Livecoding #13: rendering React components with canvas
- Livecoding #12: towards animating 10k+ elements with React
- Livecoding #15: Reaching the limits of canvas redraw speed
- Livecoding #16: canvas.drawImage performance is weird but magical
- Declarative `canvas` Animation with React and Konva
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️