This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding. Usually shorter than 500 words. Often with pictures. Livecoding happens almost every Sunday at 2pm PDT on multiple channels. You should follow My Youtube channel to catch me live.
This Sunday, I created an excuse to learn JavaScript async/await: Morty's Mindblowers.
Async/await is meant to be the bestest way to deal with asynchronous code in JavaScript. Even better than promises. And since the web is littered with async/await tutorials, it must be really hard, right?
¯\_(ツ)_/¯
No… just like Promises, it's nothing but a monad. A nice syntax sugarified monad, but still a monad. You can learn how to use async/await in 10 seconds.
I'm kind of disappointed in how simple async/await is.
— Swizec Teller (@Swizec) October 1, 2017
It's just a monad with some syntax sugar :D
Watch. Read 👇
If you know Promises, you know async/await. Congratz! 👏
Let's say you want to fetch a list of images from Imgur and narrow it down to videos. With Promises, it looks like this:
class Imgur {
static URL = "https://api.imgur.com/3/";
static CLIENT_ID = "c848e36012571f2";
static gifs(page) {
return fetch(`${Imgur.URL}gallery/hot/rising/${page}`, {
headers: { Authorization: `Client-ID ${Imgur.CLIENT_ID}` },
})
.then((res) => res.json())
.then((json) => {
if (json.success) {
return json.data.filter(({ type }) => type === "video/mp4");
} else {
throw new Error(json.data.error);
}
});
}
}
We have a static gifs()
method that uses fetch
to talk to Imgur and return a Promise. The promise resolves with either a list of Imgur videos, or it rejects with an API error.
Using static
lets us call this API without instantiating an object. Imgur.gifs()
for instance. Great for when you're using classes to group stuff and don't need objects.
Ok, so this code is pretty readable, right? Fetch data, then parse it, then do things.
Serverless Handbook for Frontend Engineers – free chapter
Dive modern backend. Understand any backend.
Serverless Handbook taught me high-leveled topics. I don't like recipe courses and these chapters helped me to feel like I'm not a total noob anymore.
The hand-drawn diagrams and high-leveled descriptions gave me the feeling that I don't have any critical "knowledge gaps" anymore.
~ Marek C, engineer
Start with a free chapter and email crash course ❤️
With async/await, that same code looks like this:
class Imgur {
static URL = 'https://api.imgur.com/3/';
static CLIENT_ID = '<your_id>';
static async gifs(page) {
const res = await fetch(`${Imgur.URL}gallery/hot/rising/${page}`,
{headers: { Authorization: `Client-ID ${Imgur.CLIENT_ID}`}}),
json = await res.json();
if (json.success) {
return json.data.filter(({ type }) => type === 'video/mp4');
}else{
throw new Error(json.data.error);
}
}
}
</your_id>
I don't know if that's more readable, but I can see the appeal. Your code looks just like any other code. No need to think about async stuff at all.
You have to put async
in front of your function name. That wraps it in a Promise. Anything you return is in a Promise monad. Time bubble to use the metaphor from my JavaScript Promises in 2 minutes article.
You peek into that monad using the await
keyword. It's like wrapping your code in a .then
callback.
That's really all there is to it. Once you say json = await res.json()
, anything that uses that json
variable gets wrapped in a .then()
call.
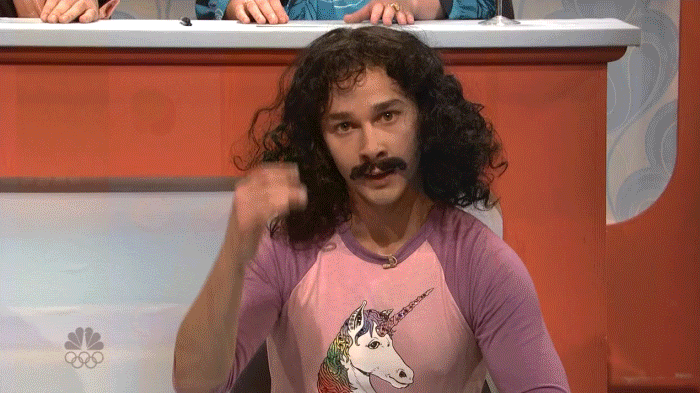
Any time you use the Imgur.gifs()
method, you have to say await
and your function must be wrapped in async
.
Oh, and you can wrap built-in React lifecycle hooks in async
and it works as expected. That part is neat. However, you can't have async getters. That would be cool.
So yeah, we got the JavaScript async/await stuff working in a couple minutes, then we spent some half an hour figuring out how Imgur's API works, and an hour or two trying to use flexbox to make the <video>
tag shrink and grow.
And in the end, we got Morty's Mindblowers. It uses cut scenes from an episode of Rick & Morty to show you random gifs from Imgur's frontpage.
Enjoy 🤘
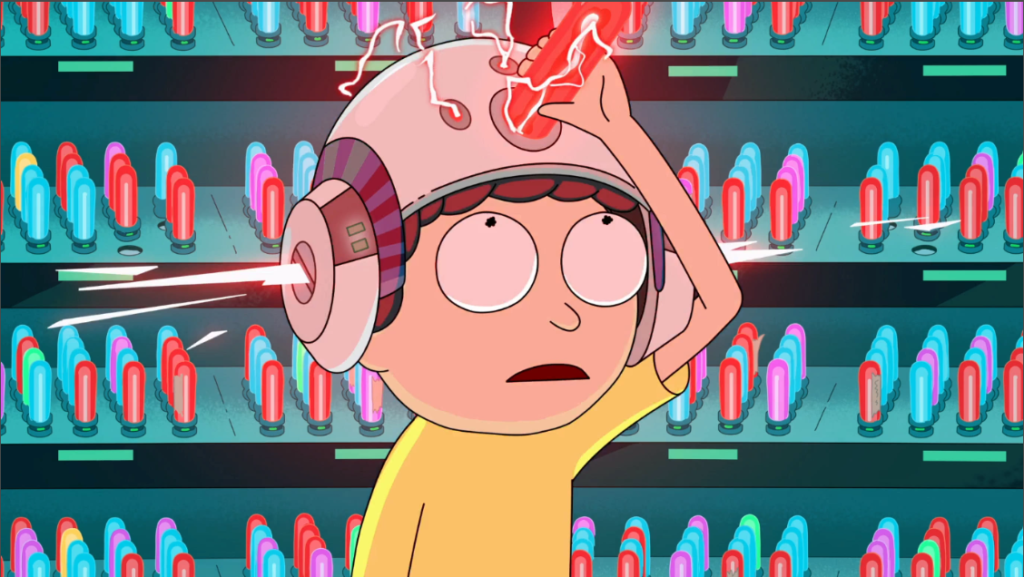
Continue reading about Livecoding Recap 48: JavaScript async/await and Morty's Mindblowers
Semantically similar articles hand-picked by GPT-4
- Using YouTube as a data source in Gatsbyjs
- JavaScript promises are just like monads and I can explain both in less than 2 minutes
- Async, await, catch – error handling that won't drive you crazy
- Livecoding Recap 48- Why contributing to big opensource projects is still hard
- Build a Chrome extension from idea to launch in an afternoon
Want to dive into serverless? Not sure where to begin?
Serverless Handbook was designed for people like you getting into backend programming.
360 pages, 19 chapters, 6 full projects, hand-drawn diagrams, beautiful chapter art, best-looking cover in tech. ✌️
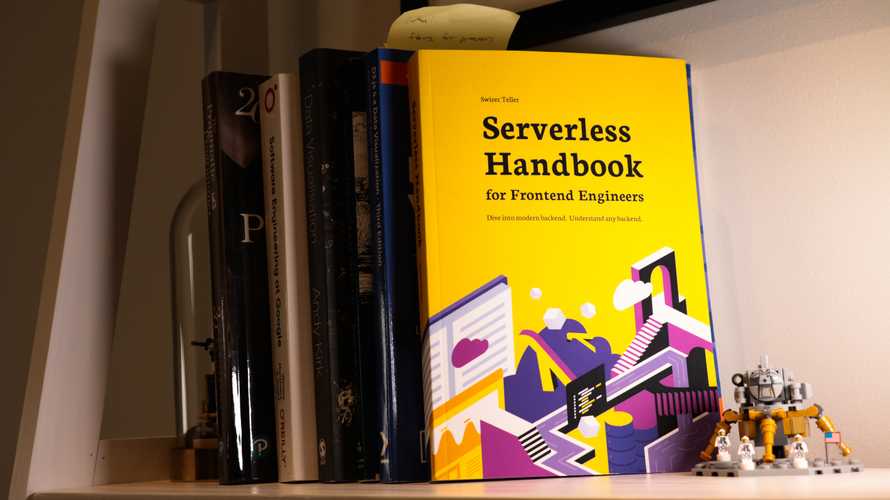
Learn how to choose the right database, write cloud functions, think about scalability, gain the architecture mindsets for robust systems, and more.
Leave your email to start with a free chapter and email crash course 👇
Serverless Handbook for Frontend Engineers – free chapter
Dive modern backend. Understand any backend.
Serverless Handbook taught me high-leveled topics. I don't like recipe courses and these chapters helped me to feel like I'm not a total noob anymore.
The hand-drawn diagrams and high-leveled descriptions gave me the feeling that I don't have any critical "knowledge gaps" anymore.
~ Marek C, engineer
Start with a free chapter and email crash course ❤️
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️