Some days your code flows, your fingers fly, and you're god amongst nerds. Other days you're testing fetch()
requests.
So I'm writing this to save you some time. The definitive how to mock and test fetch requests guide for 2020.
First, a little background
Why even test requests?
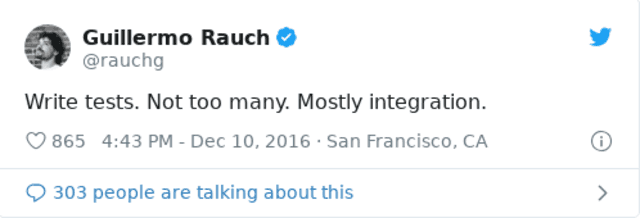
Integration testing. Also known as the only useful testing. 😛
Semantics aside, most bugs happen on the edges. The touch points between systems. Interfaces between modules.
What bigger in-between point than calling an API? Lots can go wrong. Server can fail, network down, strange response codes, or just corruption on the wire.
Take for example this isAuthenticated
method from a library I built at work.
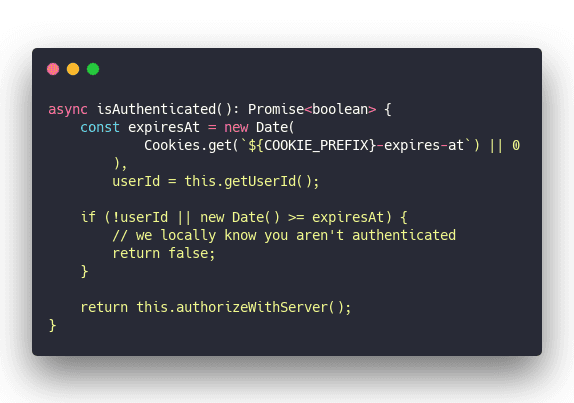
Is the user authenticated? Check local cookies. That's always gonna work.
And if cookies look correct, you gotta ask the server if they're actually correct. User might have been banned, deleted, access revoked, or just changed their password.
That API request is the most likely failure point.
Wanna really test this method? Gonna have to test the fetch.
Why mock fetch requests?
A pure unit testing approach says you should never mock requests to other systems.
You assume the request works and test that your function makes the request. Then you test the function that's called after the fetch.
And you enter the unit testing fallacy.
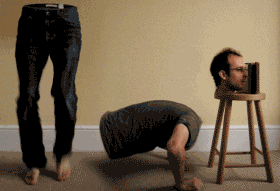
Besides, structuring your code to allow a pure unit testing approach makes it harder to read, understand, and tedious to work with. You'd need something like this:
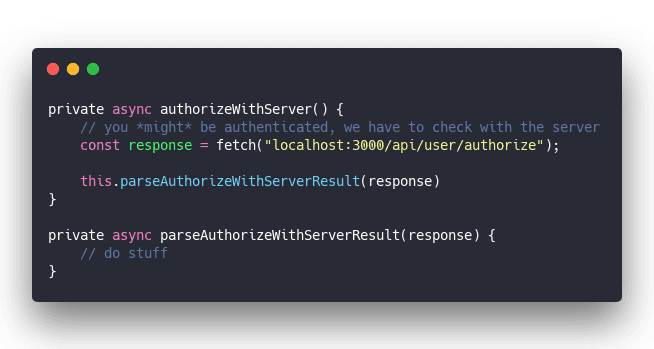
Yeah that's not gonna get out of hand 🙄
Keep your code simple and mock the request instead. Hook into the fetch()
API and manipulate what it returns. That is the way to testing bliss my friend.
How to mock fetch requests in Jest
After more hours of trial and error than I dare admit, here's what I found: You'll need to mock the fetch
method itself, write a mock for each request, and use a fetch mocking library.
install
The library that worked best for me was fetch-mock
.
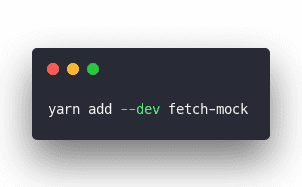
mock
You tell Jest to use a mock library like this:
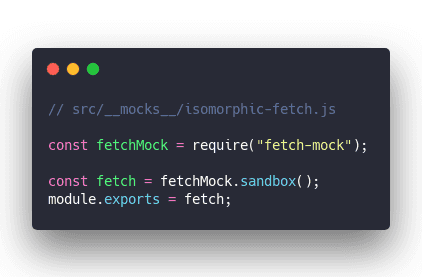
Jest imports this file instead of isomorphic-fetch
when running your code. Same approach works to replace any other library.
Put a file of <library name> in src/__mocks__
and that file becomes said library. In this case we're replacing the isomorphic-fetch
library with a fetch-mock
sandbox.
So when your code says
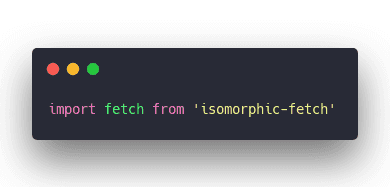
It's actually getting the sandbox 🤘
You'll need to import a fetch to support mocking – can't rely on the global window.fetch
I'm afraid. You can't use that in test environments anyway since it doesn't exist.
types
Using TypeScript in tests, you'll have to jump through another hoop: TypeScript doesn't understand that isomorphic-fetch is mocked and gets confused.
You have to tell the type system that "Hey, this is actually fetch-mock. All is well."
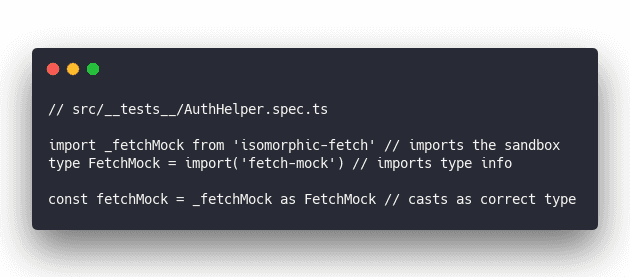
Convoluted but how else is TypeScript supposed to know isomorphic-fetch
is actually fetch-mock
...
PS: I'm assuming Jest because it's become the industry standard for JavaScript testing in the past few years.
And now it works ✌️
All you gotta do now is mock a request and write your test.
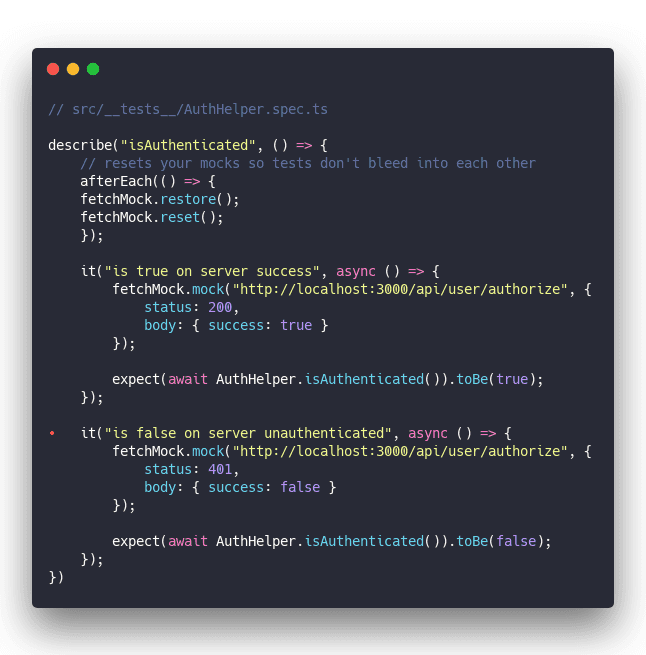
Each of those tests is saying "When you fetch() this URL, return this object. No network calls are made, no servers harmed, no ambiguity about what happens. Just solid tests doing exactly what you want.
And now you know your code works. Bliss 😌
Happy Friday,
~Swizec
Continue reading about Mocking and testing fetch requests with Jest
Semantically similar articles hand-picked by GPT-4
- How to waste hours of life with fetch() and a bit of brainfart
- How to configure Jest with TypeScript
- The Big Mac index and Jest fetch testing
- JavaScript can fetch() now and it's not THAT great
- A tiny ES6 fetch() wrapper that makes your life easier
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️