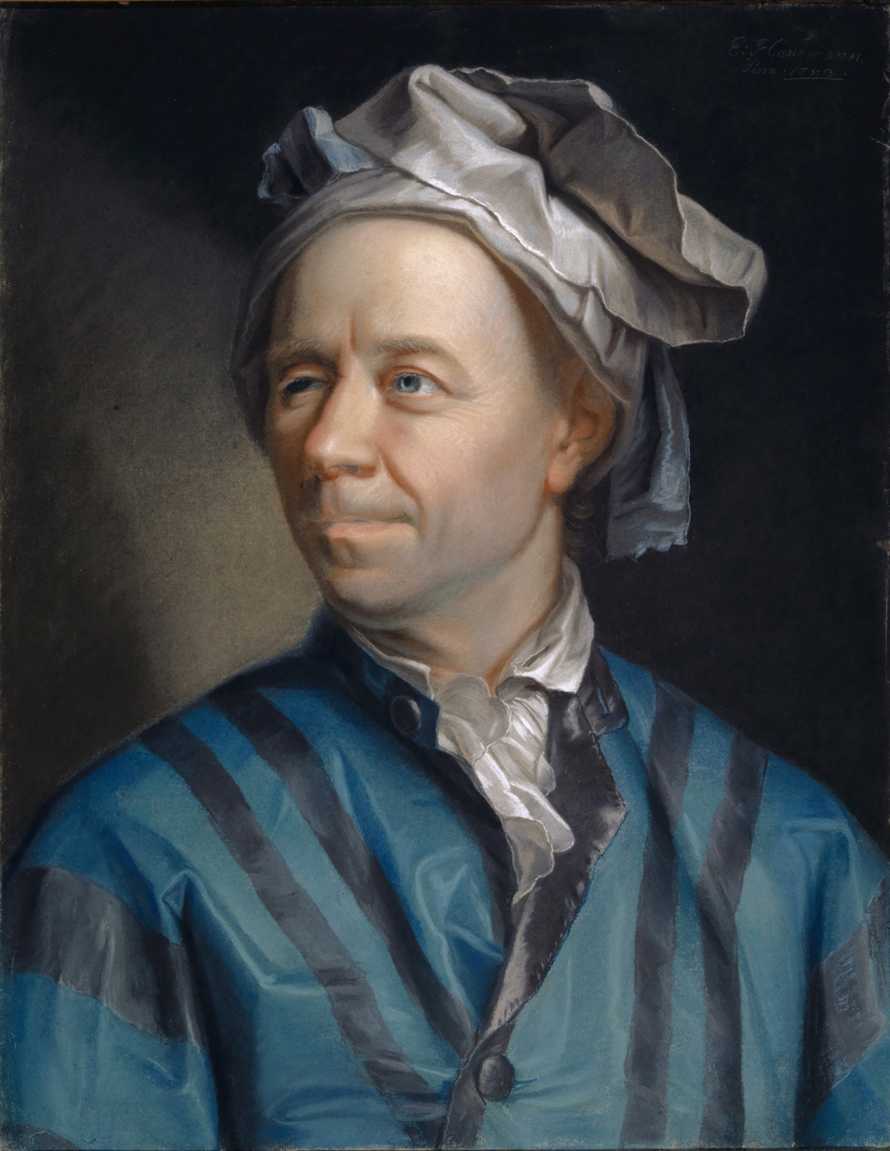
Yesterday I was a bit bored at the Theoretical Basis Of Computer Science class and the obvious solution was to try doing a bit of coding on an algorithm I'm trying to develop that aims to learn the proper syllabification for a language by reading it. Because I'm all sorts of cool I want to develop this thing in Clojure.
Naturally, the moment I started my severe lack of proficiency in clojure started showing through and I found myself spending more time online than coding. Somehow I ended up on stackoverflow where some guy suggested to another guy they go through the Project Euler problem set to get better at Clojure.
And so I did just that.
Have only managed to solve the first three problems so far, but by god this crap is fun. Maybe I'm just being way too dorky, but it's #WINNING!
Me being me, I've decided to motivate myself to solving these by posting a solution on this blog every time I solve a problem.
Here are the first three, probably less than elegant, but they're mine and I love them!
;If we list all the natural numbers below 10 that are multiples of 3 or 5, we get 3, 5, 6 and 9. The sum of these multiples is 23.
;Find the sum of all the multiples of 3 or 5 below 1000.
(defn nums [max n]
(loop [cnt (+ n n) acc [n]]
(if (>= cnt max) acc
(recur (+ cnt n) (concat acc [cnt])))))
(defn answer [max]
(println (reduce + (set (concat (nums max 3) (nums max 5))))))
(answer 1000)
;Each new term in the Fibonacci sequence is generated by adding the previous two terms. By starting with 1 and 2, the first 10 terms will be:
;1, 2, 3, 5, 8, 13, 21, 34, 55, 89, ...
;By considering the terms in the Fibonacci sequence whose values do not exceed four million, find the sum of the even-valued terms.
(defn term [p-2 p-1]
(+ p-2 p-1))
(defn fib [max p-2 p-1 acc]
(if (>= p-1 max) acc
(fib max p-1 (term p-2 p-1) (concat acc [p-1]))))
(defn fibonacci [max p-2 p-1]
(fib max p-2 p-1 [1]))
(defn bla [a]
(if (even? a) a 0))
(defn answer [max]
(reduce + (map #(if (even? %1) %1 0) (fibonacci max 1 2))))
(println (answer 4000000))
; The prime factors of 13195 are 5, 7, 13 and 29.
; What is the largest prime factor of the number 600851475143 ?
(defn any? [l]
(reduce #(or %1 %2) l))
(defn prime? [n known]
(loop [cnt (dec (count known)) acc []]
(if (< cnt 0) (not (any? acc)) (recur (dec cnt) (concat acc [(zero? (mod n (nth known cnt)))]))))) (defn next-prime [primes] (let [n (inc (count primes))] (let [lk (if (even? (inc (last primes))) (+ 2 (last primes)) (inc (last primes)))] (loop [cnt lk p primes] (if (>= (count p) n) (last p)
(recur (+ cnt 2) (if (prime? cnt p) (concat p [cnt]) p)))))))
(memoize next-prime)
(defn n-primes [n]
(loop [cnt 1 p [2]]
(if (>= cnt n) p
(recur (inc cnt) (concat p [(next-prime p)])))))
(defn factor [n factors primes]
(if (== n 1) factors
(loop [p primes]
(if (== 0 (mod n (last p))) (factor
(/ n (last p))
(concat [(last p)] factors)
p)
(recur (concat p [(next-prime p)]))))))
(println (factor 600851475143 [] (n-primes 1)))
The third one took particularly long to figure out because I was going about it all wrong. What I later realized was that I don't at all have to spend time finding the next prime for the sequence of already known primes because factorization doesn't have to be that complicated. Could just have gone through the number and anything it would divide by would already be a guaranteed prime.
Continue reading about Project euler is a fun way to become a better geek
Semantically similar articles hand-picked by GPT-4
- Checking for primes? Dumber algorithm is faster algorithm
- Learning me a Haskell
- Comparing clojure and node.js for speed
- Collatz, Haskell and Memoization
- Lychrel numbers
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️