This exploration will feed into a new VR/3D chapter in my React dataviz book. Preorder it now 👉
My goal was to build a 3D scatterplot that you can explore. Stand inside your data and look around.
Wouldn't that be cool?
Instead, I got a squished Baymax healthcare companion. 🤔
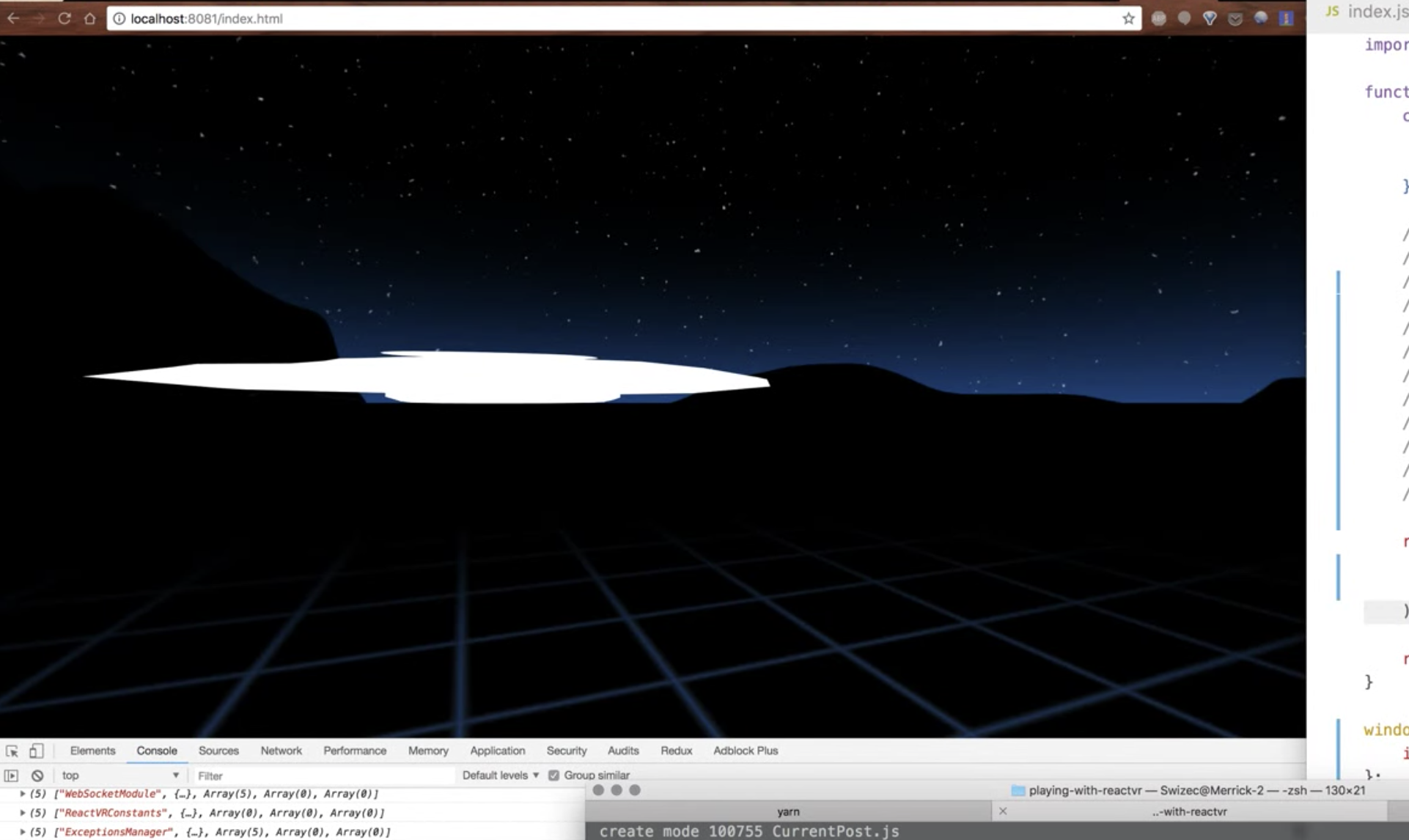
ReactVR, recently renamed to react-360, doesn't have 3D modeling primitives like spheres and boxes. Instead, it asks you to import 3D models and build your scenes with that.
The base example is a 3D viewer of models from Google's Poly library.
And that works great 👇
You can import a model, display it with a React component, and look around using VR goggles and the Oculus browser. Just gotta make sure there's a URL that shows your VR app.
To display a 3D model…
To display a 3D model in VR space, you put it in a react-360 <View>
component, add some light, and an <Entity>
.
class Baymax extends React.Component {
render() {
return (
<view>
<ambientlight intensity={1.0} color="#ffffff">
<pointlight
intensity={0.4}
style={{ transform: [{ translate: [0, 4, -1] }] }}
>
<entity source={{ obj: baymax }}></entity>
</pointlight>
</ambientlight>
</view>
);
}
}
Lighting doesn't do much in my <Baymax>
example. I'm not sure why. Maybe because the whole model is white 🤔
Where it gets interesting is the scale transformation. In theory, you can scale your models on all 3 axes. In practice, the scaleZ
transform is currently not implemented and throws an error. Even though it's in the docs. Oops.
But I guess that's the problem with models you import from the web because you don't know how to make your own. Their scales are out of whack.
The Baymax model, for example, looks great when it's 200 meters away. Yes, units for all vectors in ReactVR and react-360 are in meters. Love it.
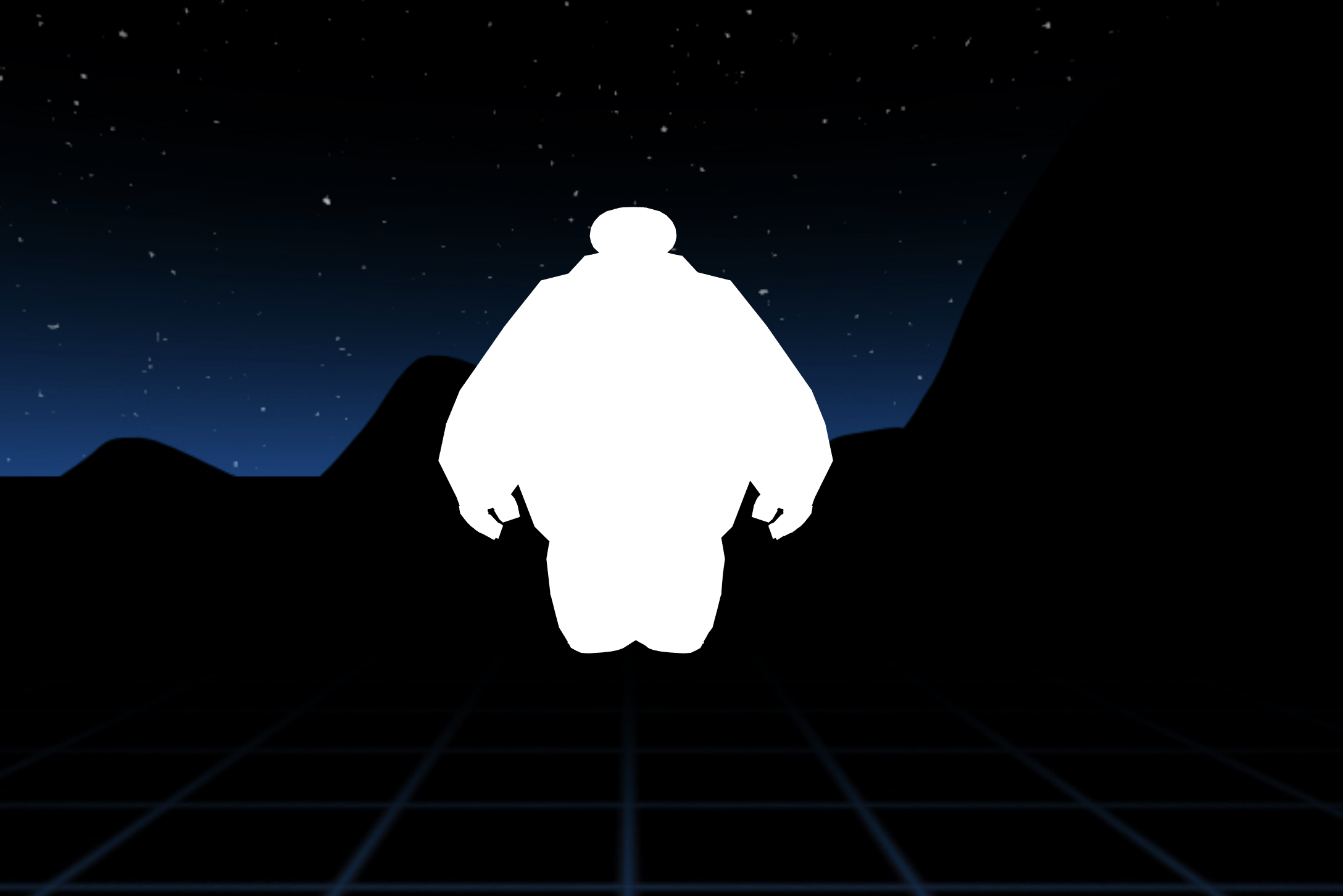
To position a react-360 view…
Positioning react-360 views happens through something they call roots. You can think of it as an anchor point for your components that you build on top of.
This goes in your client.js
and you can have as many roots as you need.
r360.renderToLocation(r360.createRoot("Baymax"), new Location([0, -30, -200]));
Location is a 3D vector based off of the user's position. In this case, that's 0
meters to the left or right, 30
meters down, and 200
meters back. Go 200
meters in the other direction, and your user has to turn their head to see your scene.
And that's where react-360 starts to shine. 360 degrees on 3 axes is a lot of space to play around with. Seriously. A ton of space. You can't even imagine until you start to play around.
react-360 shines at placing 2D views in 3D space
What I believe react-360 is truly optimized for is putting 2D panels in 3D space and giving your users more room to use different controls.
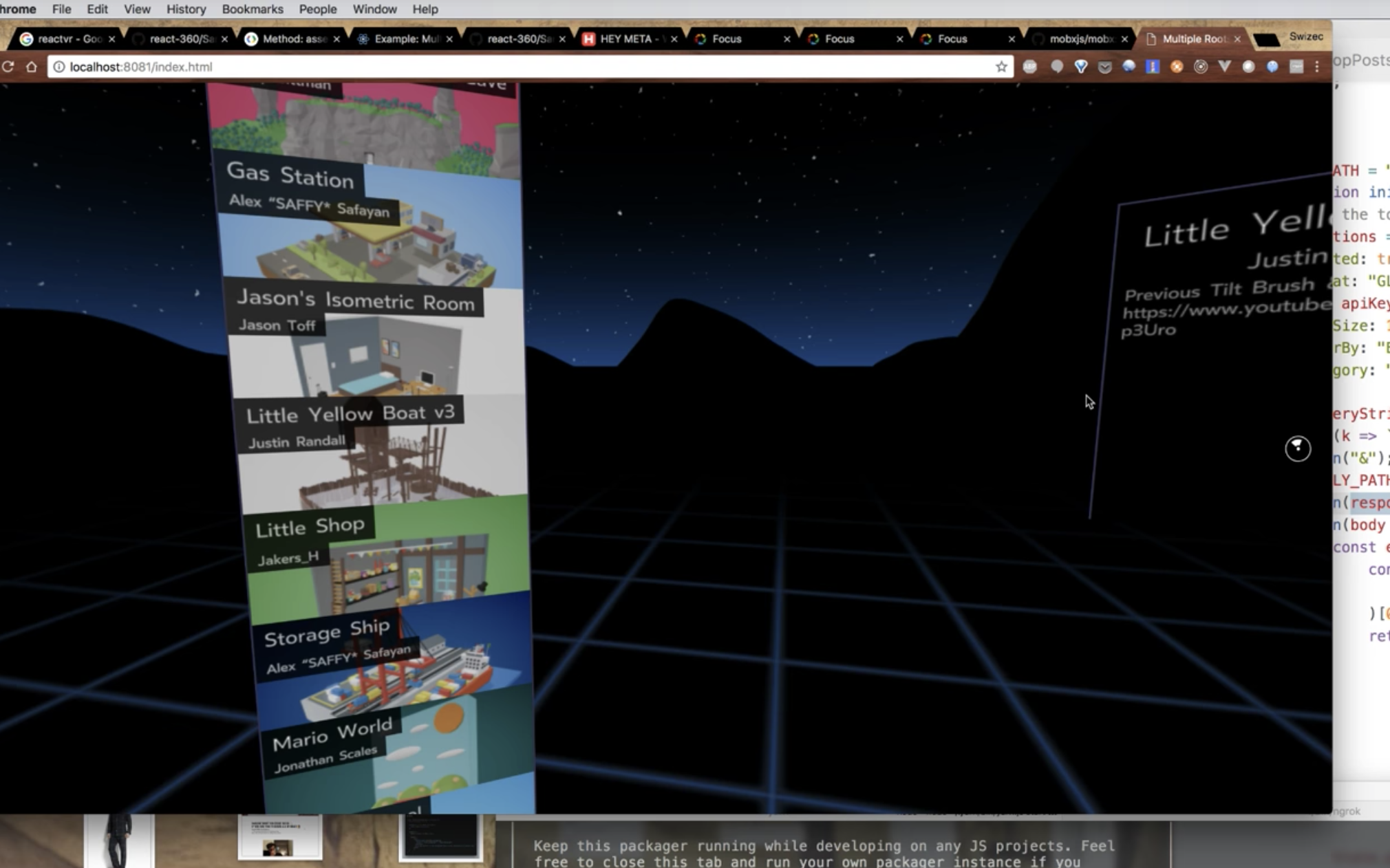
It's kind of a shame that this is the case, but there's a lot you can do with 2D in 3D. Imagine a data dashboard for your infrastructure that uses full 3-axis 360 views to place charts all around you instead of trying to squish everything into a small browser window.
I don't know about you, but I always run out of space when making monitoring dashboards 😅
Code looks just like React Native by the way. You have <View>
s and <Text>
s and <Image>
s and <VrButton>
s. You put them together and voila, an interface.
This builds that panel on the left 👇
const TopPosts = props => {
if (!props.posts) {
return (
<view style={styles.wrapper}>
<text>Loading...</text>
</view>
);
}
return (
<view style={styles.wrapper}>
<text style={styles.postButtonName}>
Posts: {props.posts.length}
</text>
{props.posts.map((post, i) => (
<postbutton key={post.id} index={i} name={post.name} author={post.author} preview={post.preview}>
))}
</postbutton></view>
);
};
Take a list of posts from Google's Poly store, render a <View>
, put some <Text>
inside to know the count, then iterate through the data and render a <PostButton>
for each entry.
The <PostButton>
component is a <VrButton>
that gives us clickability and callbacks. It contains an image and two text labels. Pretty neat.
You place the panel in 3D space with a root, a surface, and a render to surface.
const leftPanel = new Surface(300, 1200, Surface.SurfaceShape.Flat);
leftPanel.setAngle(-0.6, 0);
r360.renderToSurface(r360.createRoot("TopPosts"), leftPanel);
The extreme 1200 height doesn't look so great in a web browser, but works great in VR goggles because you can look up and down.
360 degree really is a lot of room for activities. Really. Wish I knew how to record what I can see inside my goggles.
Fin
There's a lot of potential here. In production mode, react-360 is fast enough to avoid nausea; in dev, not so much. The engineering UX is similar to React Native, and VR is growing like crazy.
You might not realize it, but there's a lot of VR users out there. 171,000,000 according to statista. Similar to the web in 1999.
Now's the time to start playing around 😉
Watch me build the squished Baymax 👇 and preorder React + D3 2018, likely the first React book/course to include VR stuff 😛
Continue reading about ReactVR/react-360 is great, but maybe not quite there yet
Semantically similar articles hand-picked by GPT-4
- Livecoding recap #41: Towards declarative 3D scenes with React and Three.js
- Livecoding Recap: A new more versatile React pattern
- Behind the curve ... of my bar donut chart 🤨
- Why dataviz does better for your career than TODOapps
- Announcing: Big update to my best selling book and course, React + D3v4
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️