Have you ever wanted to render a Preact component inside a React project?
It's hard, right? First of all, why? Because. Second of all, how do you reconcile the two different implementations of JSX?
Preact and React are pretty similar, you see. Both are based on components, both leverage JSX to make your life easier, and for the most part, they look interchangeable.
// React component
import React from 'react';
const Header => <h1>I am a header</h1>
// Preact component
import Preact from 'preact';
const Header => <h1>I am a header</h1>
Where they differ greatly is what that <h1>I am a header</h1>
compiles to. For React, it's a createElement()
call. For Preact ,it's a h()
call.
And that's where the trouble begins.
Reconciling createElement() and h()
I got this idea from Jason himself.
Set your pragma to `h` and conditionally assign h within the scope you want:
— Jason Miller 🦊⚛ (@_developit) February 26, 2018
/** @jsx h */
import React from 'react'
import { h } from 'preact'
const WithReact = () => {
let h = React.createElement;
return <div>react</div>
}
const WithPreact = () => (
<div>preact</div>
)
Here's how it looks in code 👇
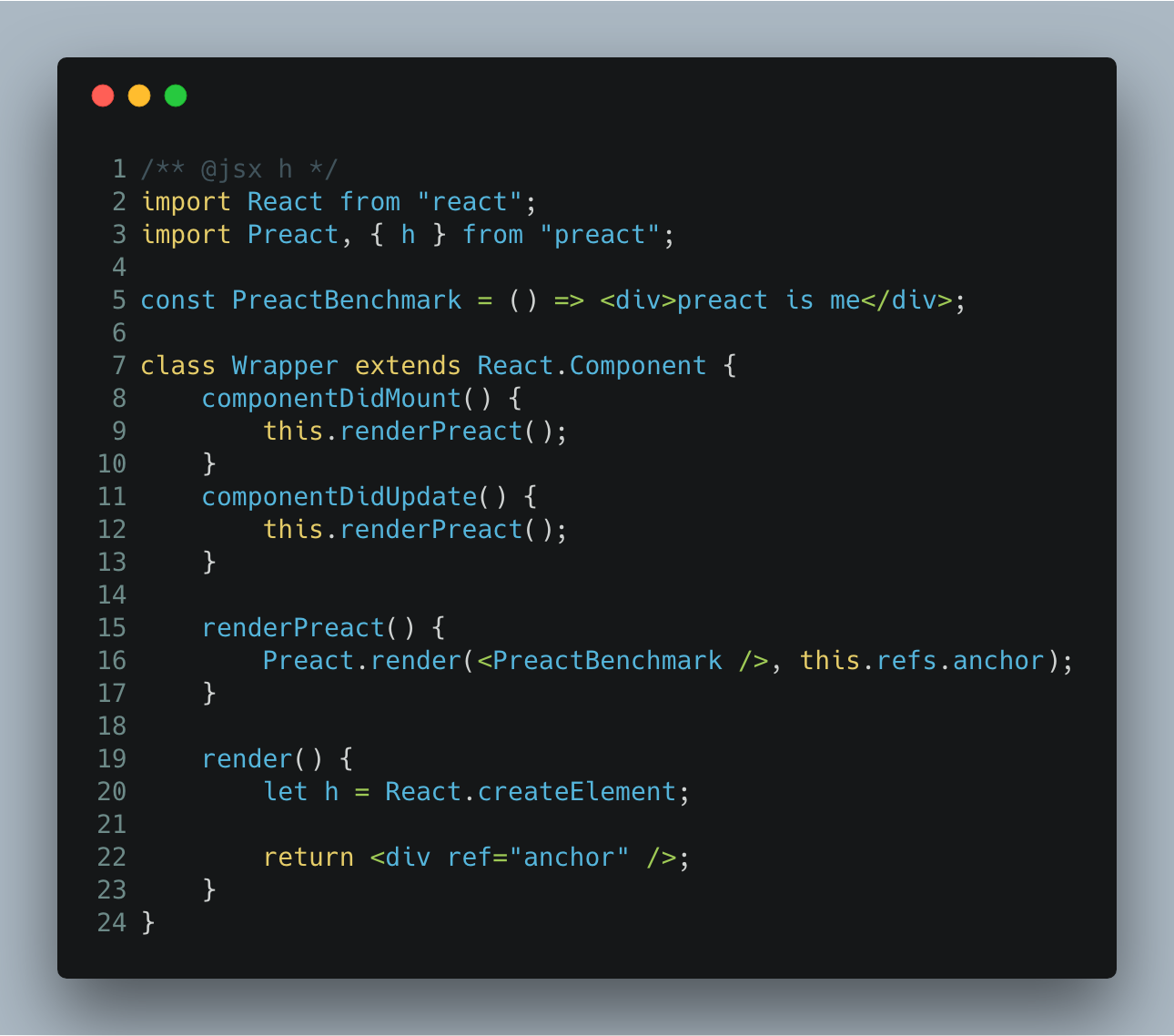
There are 4 parts to this:
- Tell Babel to use
h
for the JSX function. That's the/** @jsx h */
magic comment at the top. - Import both React and Preact. You'll need both.
- Make a Preact component. Or take one from somewhere else. It doesn't matter. Whatever you want :)
- Create a
<Wrapper />
React component
That <Wrapper />
component is where the magic happens.
The render()
method sets h
to React.createElement
and outputs an anchor div. Setting h
ensures that this part of JSX compiles into React's createElement
calls. This makes the component integrate seamlessly with the rest of your project.
Then we hook into the component lifecycle with componentDidMount
and componentDidUpdate
. We call renderPreact
in both of them to ensure our wrapper component always ends up rendering Preact.
Same as my React D3 blackbox approach for quickly wrapping D3 code in React components.
In renderPreact
, we then use Preact's render()
function to render the Preact component into our anchor div. This works the same way as Preact's normal DOM rendering where you call render(<App />, document.getElementById('root'))
to put your app into a root div.
The JSX compiles into Preact's h()
calls because we didn't mess with the setting, and React refs give us a direct reference to the anchor DOM node.
👌
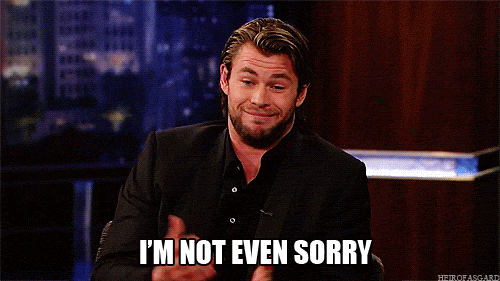
The benchmark
You can now compare React and Preact side-by-side in my interactive DOM benchmark. I think it's a fair comparison because Preact handles its own internals.
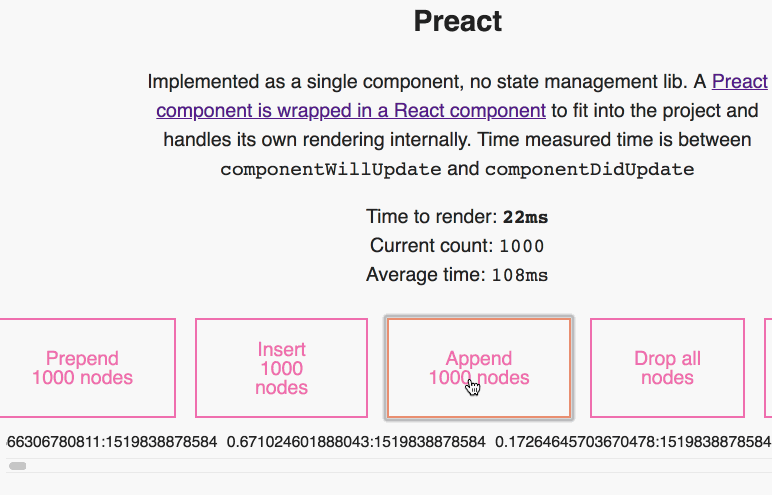
Numbers are kinda high though. Preact is supposed to be faster than React because it's closer to the metal. I wonder if it's running in dev mode 🤔
Continue reading about Seamlessly render a Preact component in a React project
Semantically similar articles hand-picked by GPT-4
- Easy D3 blackbox components with React hooks
- React components as jQuery plugins
- An experiment to merge React and Vue
- Livecoding Recap: A new more versatile React pattern
- Building an interactive DOM benchmark, preliminary results
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️