Yes it's new, I just open sourced it 😛
The other day I wanted to measure some DOM nodes. This is useful when you have to align items, or respond to browser width, or ... lots of reasons okay.
I had to align a curvy line with elements that aren't under my control. This little stepper component uses flexbox to evenly space circles, CSS layouting aligns the title, and you see where this is going.
Doesn't look like much but it's handy for data visualization. Especially when you want to align things with other things.
— Swizec Teller (@Swizec) March 12, 2019
I used a few of those snippets to position a curved line on this stepper pic.twitter.com/W9RLizRLPG
SVG in the background detects position of itself, positions of the title and circle, and uses those to define the start
and end
line of my curve. 👌
Many ways you can do this.
@lavrton linked to a list of existing NPM packages that sort of do it. @mcalus shared how he uses react-sizeme
to get it done.
All great, but I wanted something even simpler. I also didn't know about them and kind of just wanted to make my own.
Here's an approach I found works great
Last night I figured out how to measure DOM dimensions with React Hooks. I'm sure it's been covered before but I had fun finding out. #200wordsTIL pic.twitter.com/TmFWZ9Chk0
— Swizec Teller (@Swizec) March 12, 2019
useDimensions hook
This seemed like a neat approach so I turned it into an open source React Hook. You might enjoy it.
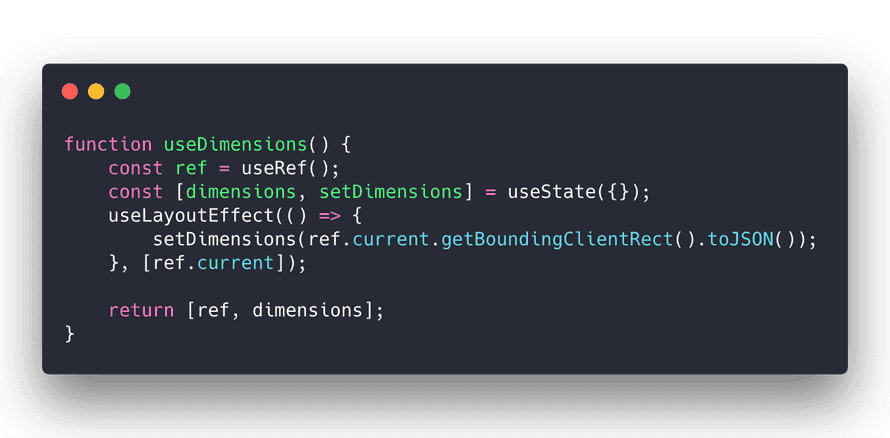
useDimensions source
Yep that's it. It really is that simple.
useRef
creates a React.ref, lets you access the DOMuseState
gives you place to store/read the resultuseLayoutEffect
runs before browser paint but after all is knowngetClientBoundingRect()
measures a DOM node. Width, height, x, y, etctoJSON
turns a DOMRect object into a plain object so you can destructure
Here's how to use it in your project 👇
First, add useDimensions
to your project
$ yarn add react-use-dimensions or $ npm install --save react-use-dimensions
Using it in a component looks like this
import React from "react"
import useDimensions from "react-use-dimensions"
const MyComponent = () => {
const [ref, { x, y, width }] = useDimensions()
return <div ref={ref}>This is the element you'll measure</div>
}
useDimensions
returns a 2-element array. First the ref
, second the dimensions.
This is so multiple useDimensions
hooks in the same component don't step on each others' toes. Create as many refs and measurement objects as you'd like.
const MyComponent = () => {
const [stepRef, stepSize] = useDimensions()
const [titleRef, titleSize] = useDimensions()
console.log("Step is at X: ", stepSize.x)
console.log("Title is", titleSize.width, "wide")
return (
<div>
<div ref={stepRef}>This is a step</div>
<h1 ref={titleRef}>The title</h1>
</div>
)
}
MIT License of course.
Consider retweeting if you think it's neat
🔥useDimensions is now an open source react hook 🔥
— Swizec Teller (@Swizec) March 13, 2019
🐙 GitHub --> https://t.co/CNPj4RVfOw
✍ short blog --> https://t.co/S5Wp6PJX95
Simple and works great 👌 pic.twitter.com/8ZWYUCUhIe
Enjoy ✌ ️
~Swizec
Continue reading about useDimensions – a React Hook to measure DOM nodes
Semantically similar articles hand-picked by GPT-4
- Trying the new ResizeObserver and IntersectionObserver APIs
- Use ref callbacks to measure React component size
- Build responsive SVG layouts with react-svg-flexbox
- Livecoding Recap: A new more versatile React pattern
- Build an animated pure SVG dynamic height accordion with React and D3
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️