The new React 16.3 brings some changes to the ecosystem that change how we go about integrating React and D3 to build data visualizations.
componentWillReceiveProps
, componentWillUpdate
and compnentWillMount
are on their way out. They were great for making React and D3 happy together, but they cause issues with the async rendering that the React team is planning for React 17.
You tend to use those now-deprecated lifecycle methods to update D3 objects' internal state. Things like setting scale domains and ranges, updating complex D3 layouts, setting up transitions, etc.
But you don't need to! You can do it all with the new lifecycle API.
Here's a small example of building a transition with React 16.3. Using only approved lifecycle callbacks and the new ref
API.
You can play with it on CodeSandbox 👇
How it works
The core issue we're working around is that when you pass new props into a component, React re-renders. This happens instantly. Because the re-render is instant, you don't have time to show a nice transition going into the new state.
You can solve this by rendering your component from state
instead of props and keeping that state in sync.
Something like this 👇
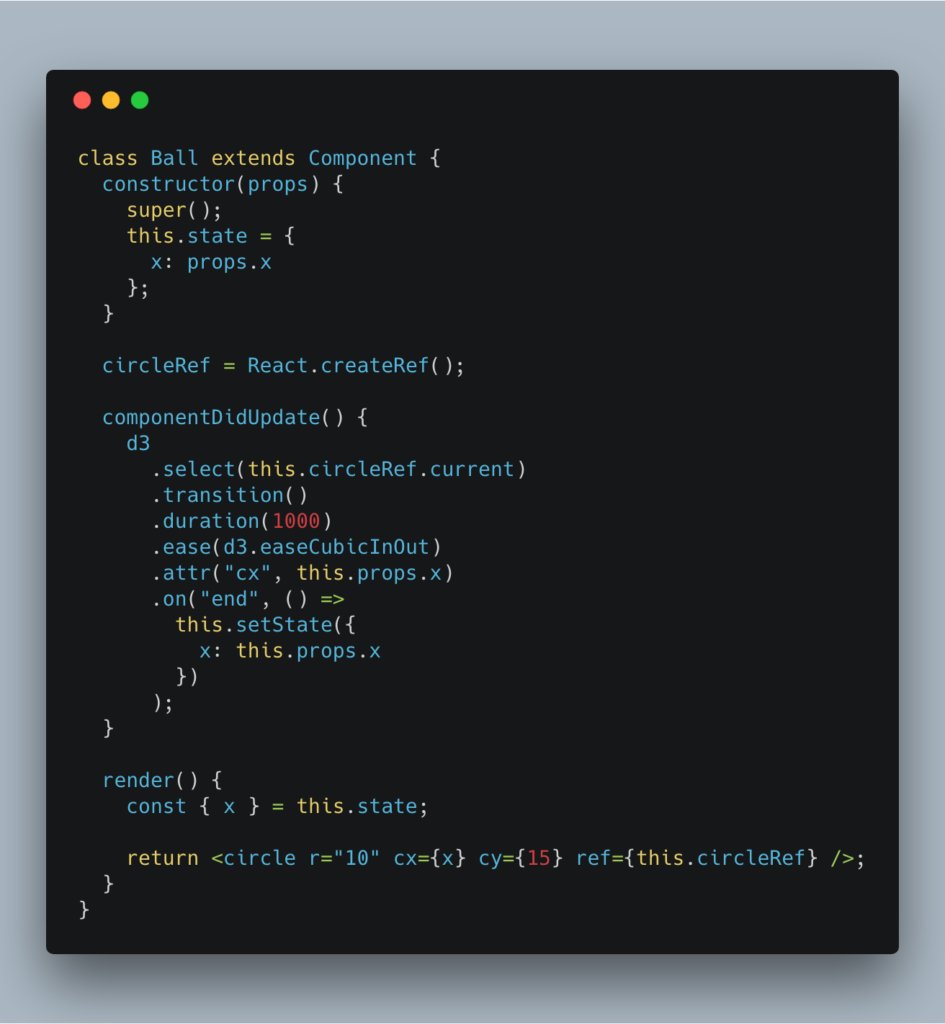
We define a Ball
class-based component. Its state has a single attribute, x
, which is set to the props.x
value by default. That way our Ball component starts off rendered at the position the caller wants.
Next, we create a new circleRef
using the React 16.3 ref API. We use this reference to give D3 control of the DOM so it can run our transition.
That happens in componentDidUpdate
.
componentDidUpdate() {
d3
.select(this.circleRef.current)
.transition()
.duration(1000)
.ease(d3.easeCubicInOut)
.attr("cx", this.props.x)
.on("end", () =>
this.setState({
x: this.props.x
})
);
}
React calls componentDidUpdate
whenever we change our component's props.
We use d3.select()
to give D3 control of the DOM node, run a transition that lasts 1000
milliseconds, define an easing function, and change the cx
attribute to the new value we got from props.
Right now, state
holds the old position and props
hold the new desired position.
When our transition ends, we update state to match the new reality. This ensures React doesn't get upset with us.
At the very end, we have our render()
function. It returns an SVG circle. Don't forget to set the ref
to this.circleRef
.
Declarative 💪
We made sure our implementation is completely declarative. To the outside world at least.
Making the ball jump left to right looks like this:
// state = { ballLeft: false }
// ballJump() flip ballLeft state
// render()
<svg style={{ width: "300", height: "100px" }} onclick={this.ballJump}>
<ball 15="" x="{ballLeft" ?="" :="" 300}=""></ball>
</svg>
Our state
holds a flag that says whether our ball is on the left. If it is, we pass an x
prop value of 15
, otherwise 300
.
When that value changes, the <Ball />
transitions itself to its new position. No need for us to worry.
If we flip positions during a transition, D3 is smart enough to stop the previous transition and start a new one. UI looks perfect.
Try it. 🏀
PS: I'm thinking about updating my React+D3v4 book for React 16.3 and D3v5. Would that interest you? Tell me on Twitter.
Continue reading about Declarative D3 transitions with React 16.3
Semantically similar articles hand-picked by GPT-4
- Declarative D3 charts with React 16.3
- Livecoding #18: An abstract React transition component
- Using d3js transitions in React
- Easy D3 blackbox components with React hooks
- Livecoding Recap: A new more versatile React pattern
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️