Dear diary, all I did today was fix 1 bug. I don't know why it happens, but I know how to make it not happen.#engineering
— Swizec Teller (@Swizec) August 6, 2016
That was on Friday. I still don’t know why the bug happens, but I can reproduce it. Evidence says it’s a Chrome 52 bug, not a general JavaScript bug. Neither Safari nor Firefox make it happen.
Sometimes when you call .fetch()
on a Backbone model, callbacks don’t fire. There’s no JavaScript error, the REST call works, the result is vanished by the browser. Joy of joys.
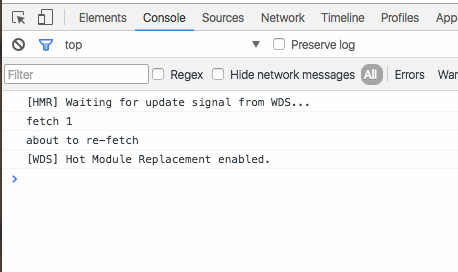
You need:
- Webpack
- Babel 6
- Backbone (maybe Backbone is doing it wrong?)
- A server that can serve JSON files
- 14 lines of code
I used create-react-app
to set up a basic environment, ejected with npm run eject
and added some config to Webpack to allow jQuery. Backbone depends on it. This part is not that relevant.
To show the bug itself, we need a Backbone model:
// A basic Backbone model
class BugModel extends Backbone.Model {
constructor() {
super();
this.url = "bla.json";
}
}
Now I know what you’re thinking, that’s an ES6 object and Backbone was built before ES6. Official docs never mention the new class
syntax. The traditional Backbone approach works too:
var BugModel = Backbone.Model.extend({
url: "bla.json",
});
The same bug happens. I tried.
To cause the bug, we have to call .fetch()
on two instances of BugModel
that share the same id
. The code looks like this:
let bug = new BugModel();
bug.fetch({
success: () => {
console.log("fetch 1"); // this happens
doWeirdness(bug);
},
});
function doWeirdness(bug) {
let newBug = new BugModel({ id: bug.id });
console.log("about to re-fetch");
newBug.fetch({
success: () => console.log("fetch 2", newBug), // don't get here
error: () => console.log("error"),
});
}
We create an instance of BugModel
in a variable called bug
, then call .fetch()
. This makes an API call to /bla.json
, which returns a JSON file that looks like this: {"id": 1, "hai": "hello"}
.
Backbone automagically parses this JSON string and sets an id
and hai
attribute. This part works like a charm.
Then we call doWeirdness
and pass our bug
object as an attribute. Inside doWeirdness
, we make a new instance of BugModel
called newBug
and give it the same id
as bug
had. This is a crucial step.
When we call fetch
on this new instance, the API call happens, but the callbacks do not. 'fetch 2'
never prints.
This whole sequence of events might sound redundant, but it made sense in my real codebase. Even if re-fetching objects doesn’t make sense, it should work. It works in both Safari and Firefox.
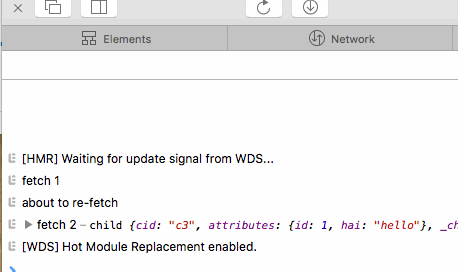
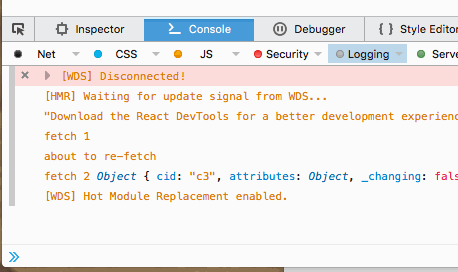
Clues about why
This smells like a bug in memory optimization. Chrome thinks bug
and newBug
are the same object, even though they are not.
If you call fetch()
twice on the same instance of BugModel
, the same bug happens. (clue, part 1)
If both instances have a different id
, both fetch
calls fire callbacks. (clue, part 2)
Curiously, if you add a third fetch()
call, that works.
newBug.fetch({
success: () => console.log("fetch 2", newBug), // don't get here
error: () => console.log("error"),
});
newBug.fetch({
success: () => console.log("fetch 3"), // prints
});
The bug didn’t happen in Chrome 51.
I am confused. ?
Continue reading about JS object optimization bug in Chrome 52
Semantically similar articles hand-picked by GPT-4
- I broke AJAX in Chrome 52 ?
- JavaScript can fetch() now and it's not THAT great
- How to waste hours of life with fetch() and a bit of brainfart
- Backbone → React – Step 1
- The commonest javascript bug
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️