This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding. Shorter than 500 words. With pictures. You can follow my channel, here. New content almost every Sunday at 2pm PDT. There’s live chat ?
Halp, I need an expert! It looks like we pushed the particle generator to the limits of canvas performance. But that can’t be right, can it? Surely I’m still doing something wrong.
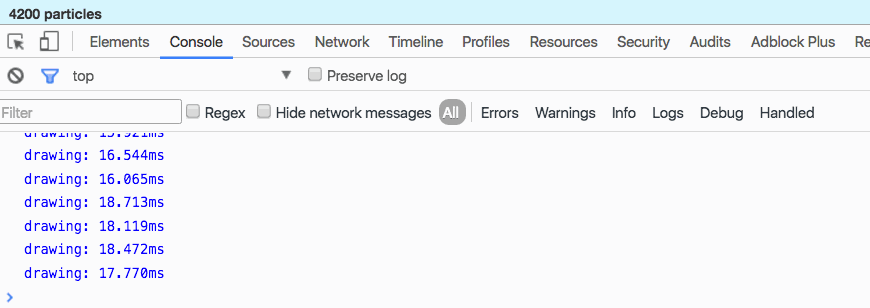
Pure canvas frame redraw speed
We removed everything that could possibly slow us down. There’s no more React or Konva for the main drawing part. All that’s left are the raw HTML5 canvas APIs. Despite our best efforts, it still takes almost 50 milliseconds to draw 10,000 circles.
A huge improvement from last time, yes. But it’s not good enough. With a drawing time of 50ms per frame, the upper bound on my laptop is about 20 frames per second. Animated, but choppy.
And there’s some 5ms of React and Redux overhead on top of that. Down to 18 frames per second.
Ugh!
The drawing code couldn’t be simpler:
drawParticle(particle) {
let { x, y } = particle;
this.context.beginPath();
this.context.arc(x, y, 1, 0, 2*Math.PI, false);
this.context.stroke();
}
componentDidUpdate() {
let particles = this.props.particles;
console.time('drawing');
this.context.clearRect(0, 0, this.canvas.width, this.canvas.height);
this.context.lineWidth = 1;
this.context.strokeStyle = 'black';
for (let i = 0; i < particles.length; i++) {
this.drawParticle(particles[i]);
}
console.timeEnd('drawing');
}
Even profiling confirms that drawing has become the slow part:
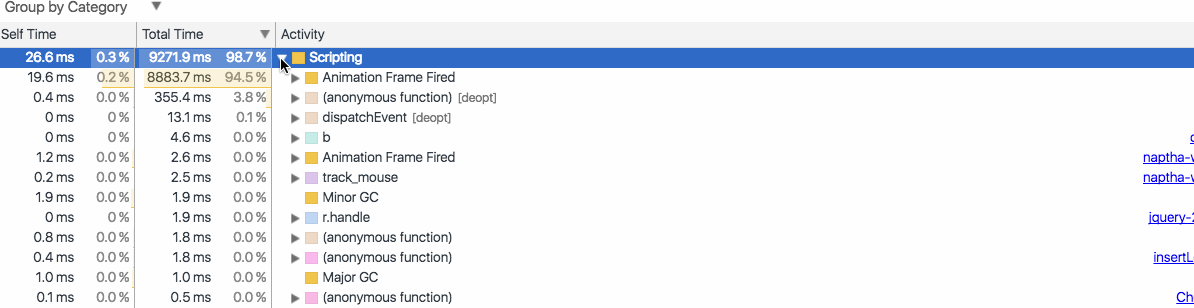
Profiling shows canvas operations are the bottleneck
I am at a loss. What else is there to try? There’s no lower layer of abstraction to drop to. Canvas is the bottom. It’s the last in the chain.
Well … there’s always manual bitmaps and those base64-encoded strings for images. If you knew how to manipulate those directly, you could trick the browser … no, that’s a silly idea. We’re not doing that. That’s crazy talk.
A friend suggested drawing a single circle in an off-screen canvas, then using drawImage
to copy-paste it around. But we tried that with Konva, and it didn’t quite work. Maybe it was a Konva problem, and it’s going to work better if we do it ourselves.
I dunno. It does look like stroke
and arc
are the biggest bottlenecks in drawCircle
. Sprites might help.
Oh, another optimization that we made was to give Konva more context about what’s going on. Disable most transformations with transformsEnabled = 'position'
on all shapes, and set listening = false
. That way it knows not to try scale/rotation transformations, and not to listen for events.
It helped a bit, but not as much as going to the bare metal for the key drawing part.
Next time, we’ll try the drawImage
approach, and if that doesn’t work, we’re going to start exploring WebGL. If that can’t do it, nothing can.
Are you a canvas expert? Please help. What am I doing wrong?
PS: the edited and improved versions of these videos are becoming a video course. Readers of the engineer package of React+d3js ES6 get the video course for free when it’s ready.
Continue reading about Livecoding #15: Reaching the limits of canvas redraw speed
Semantically similar articles hand-picked by GPT-4
- Livecoding #13: rendering React components with canvas
- Livecoding #16: canvas.drawImage performance is weird but magical
- Livecoding #14: Mostly-smooth animation up to 4,000 elements with React and canvas
- Livecoding #12: towards animating 10k+ elements with React
- Declarative `canvas` Animation with React and Konva
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️