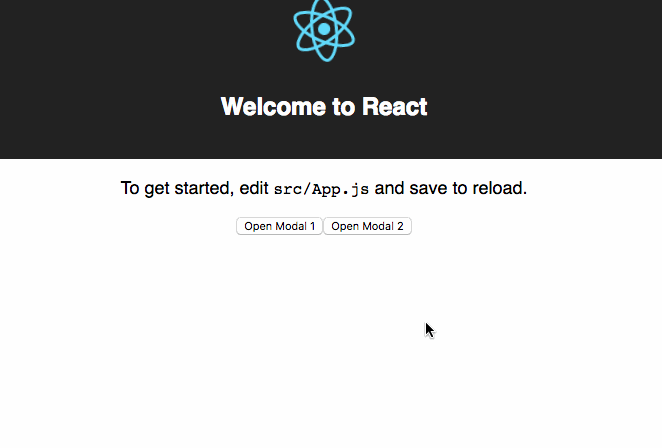
Continuing from yesterday's article about using MobX with create-react-app, we're going to build a simple MobX-driven modal. Why? Because I just did it at The Day Job™, and I think it's neat.
If you don't think it's neat, that's okay. It's neat anyway.
Ingredients:
You can see the full code on GitHub here. There's a link to a compiled&hosted version. I'm still figuring out why the component inception from the gif doesn't work after compiling. Let me know if you have any ideas.
We're going to build a modals system that lets you:
- easily open a modal from anywhere in the code
- specify general modal styling/behavior just once
- define modal contents at call point
- tie modals to URLs if needed
All of this might sound easy to you, but it's harder than it looks. It sounds easy, and it's frustrating as hell every damn time you do it. I've done it more times than I dare count.
MobX with React has been the easiest and most flexiblest approach I've ever used.
You start with a store.
// src/App.js for example purposes
class Store {
@observable modal = {
show: false,
body: null,
};
@action showModal(body) {
this.modal.show = true;
this.modal.body = body;
}
@action closeModal() {
this.modal.show = false;
this.modal.body = null;
}
}
Your real life data store would be bigger and have more properties and more actions. This is an example.
We have an @observable
property modal
, which has a boolean to denote whether the modal is shown, and a variable for the modal body. Ideally, it's a React component, but you can use anything that React's engine knows how to render.
MobX uses the @observable
decorator to create deep getters and setters for object properties. This allows the MobX engine to know when properties are dereferenced (read or set), and run relevant observers.
We use the @action
decorator to say that a function is an action. I don't know if this does anything practical, but it makes your code better.
If you only change store properties in actions, then you can model your application as a state machine. If you can model your application as a state machine, then you can understand what it's doing and your life will be easier.
Limiting yourself to performing changes in actions increases boilerplateiness a little tiny bit, and gives you this in return:
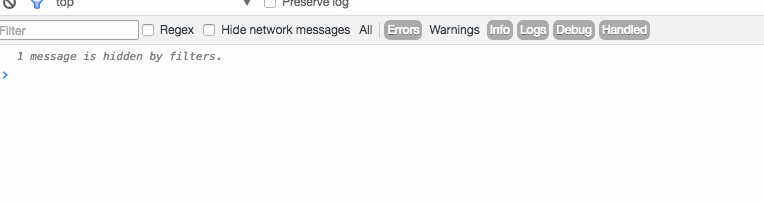
Trust me, you want it. ?
In App.js
, you add a helper function or two. These aren't necessary, but make the markup code cleaner. You'll see.
// src/App.js
@observer
class App extends Component {
store = new Store();
// this is useful for debugging only
componentDidMount() {
window.store = this.store;
}
openModal1() {
this.store.showModal(<strong>Strong Hello</strong>);
}
openModal2() {
this.store.showModal(<app>);
}
</app>
Note that your App
must be an @observer
. This allows the MobX engine to infer which data store changes are interesting to your component.
In our example, the openModal
functions invoke the this.store.showModal
action and give it some JSX as the modal body. That's the nice thing about HTML as a first-class citizen: You can pass it around any way you want. Neat ?
For the modal component itself, we're using simple-react-modal
because it's got a clean API and makes it easy to override the styling. Ask your designer; I'm sure they want custom styling if they can get it.
// src/App.js -> render()
<modal
show={this.store.modal.show}
closeonouterclick={true}
onclose={this.store.closeModal.bind(this.store)}
>
<a onclick={this.store.closeModal.bind(this.store)} style={closeStyle}>
X
</a>
{this.store.modal.body}
</modal>
We're deferring to simple-react-modal
to provide the Modal
component and take care of the basic styling. Dark backdrop, centered white rectangle, that sort of thing.
We tell it to show itself via the show
prop, which we feed straight from our data store. To close our modal, we use the store.closeModal
action, which sets show
to false
. Isn't that cool? Flipping a boolean makes a modal show or hide.
For full flexibility, we use this.store.modal.body
to render the modal's body. React makes it easy to store just about anything in that property. Even <App />
itself… but only in debug mode ?
And… yeah… that's about it, really. You can open modals from anywhere in your application that has access to the data store. Call store.showModal(<stuff>)
, and it shows up. Call store.closeModal()
, and it goes away.
You could tie it to your routing and make it so users can refresh the page and keep the modal. I wish more webapps did that.
PS: if you put the store in window
, you can call actions in your browser console and see how your app reacts.
Continue reading about Simple MobX-driven modals
Semantically similar articles hand-picked by GPT-4
- How to structure your MobX app for the real world
- Livecoding #25: Adding MobX to a vanilla React project
- How to use MobX with create-react-app
- Do you even need that bind?
- useReducer + useContext for easy global state without libraries
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️