This is a Livecoding Recap – an almost-weekly post about interesting things discovered while livecoding. Usually shorter than 500 words. Often with pictures. Livecoding happens almost every Sunday at 2pm PDT on multiple channels. You should follow My Youtube channel to catch me live.
17 years ago, in 6th grade, I set out to build a 3D spinning cube and failed miserably. You can't do 3D without sine and cosine.
Today, I made it happen! Two 3D spinning cubes. 🤘🏼
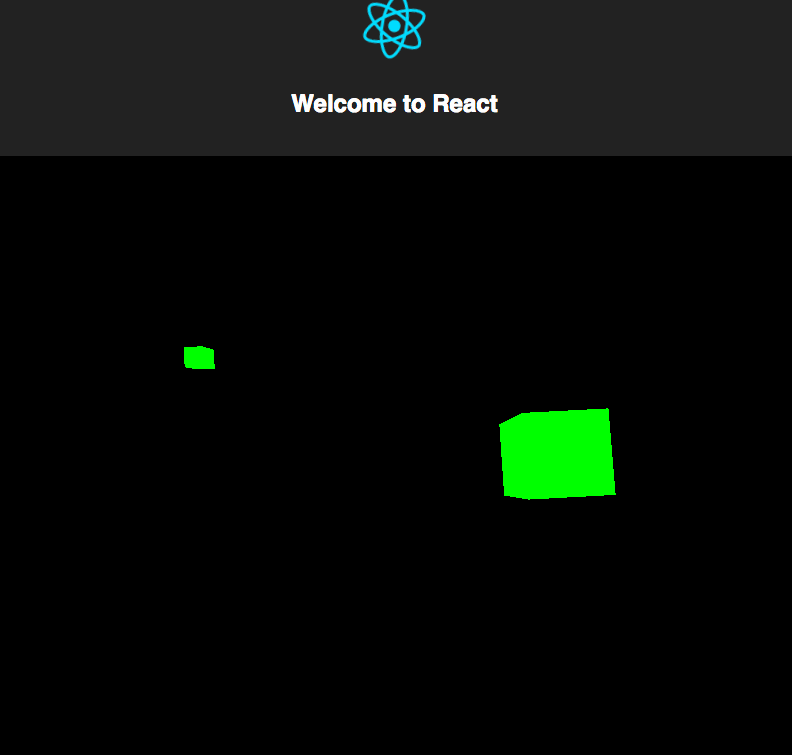
As promised poolside on Sunday, this week's livecoding session happened on Monday. Together, we admired the speed of my new laptop, wondered why YouTube still garbles my voice, and built two green spinning 3D cubes. Declaratively 😏
The main render code looks like this:
<div class="App-intro">
<threescene width={800} height={600} style="{{margin:" '0="" auto'="" }}="">
<perspectivecamera fov={75} aspect={800/600} near={0.1} far={1000} position="{{x:" 0,="" y:="" z:="" 30}}="">
<cube rotation={rotation1} position="{{x:" 2,="" y:="" 0,="" z:="" 25}}="">
<cube 10="" rotation={rotation2} position="{{x:" -10,="" y:="" 5,="" z:="" }}="">
</cube></cube></perspectivecamera>
</threescene>
</div>
A ThreeScene
, which is a scene done with Three.js, contains a PerspectiveCamera
, which contains 2 Cube
elements. Cube positions come from state, get changed on requestAnimationFrame
in an infinite loop, and voilà: Two Spinning Cubes.
The goal was to come up with a declarative approach to creating 3D scenes. Write React components, use all the normal React idioms, and have stuff come out in 3D.
I'm sure someone's already built a React-to-Three library, but I wanted to give it a shot myself. You can think of this as a proof of concept.
This is also the first time I've played with Three.js, and I have to say, it's easier than I thought it would be. At least to render two cubes. Other stuff I'm sure is super hard.
Here's how it works. 👇
PS: Full code is on GitHub
Step 1: A <ThreeScene>
class ThreeScene extends Component {
scene = new THREE.Scene();
renderer = new THREE.WebGLRenderer();
componentDidMount() {
this.updateThree(this.props);
this.refs.anchor.appendChild(this.renderer.domElement);
}
componentDidUpdate() {
this.updateThree(this.props);
}
updateThree(props) {
const { width, height } = props;
this.renderer.setSize(width, height);
}
getChildContext() {
return {
scene: this.scene,
renderer: this.renderer
}
}
render() {
const { width, height, style } = this.props;
return (
<div ref="anchor" style="{[{width," height},="" style]}="">
{this.props.children}
</div>
)
}
}
ThreeScene.childContextTypes = {
scene: PropTypes.object,
renderer: PropTypes.object
}
A ThreeScene
component defines a new Three.js Scene
and a WebGLRenderer
. Both are added into React Context via getChildContext
.
Context is messy but it gives every child access to the scene and renderer. They'll need the scene for rendering themselves, and the camera component will need access to the renderer.
In componentDidMount
, we manually update the DOM and appendChild
to the rendered anchor element. This mounts our Three.js canvas to the DOM.
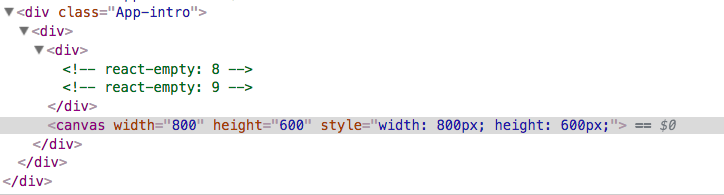
Step 2: A <PerspectiveCamera>
Three.js comes with a bunch of different camera configurations, and I don't know what they all mean. I think one of them, the StereoCamera, is meant for VR.
The base example uses PerspectiveCamera
, so that's what I used, too. This component sets up our camera and renders our scene.
class PerspectiveCamera extends Component {
constructor(props) {
super(props);
this.updateThree(props);
}
componentDidUpdate() {
this.updateThree(this.props);
this._render();
}
updateThree(props) {
const { fov, aspect, near, far, position } = this.props;
this.camera = new THREE.PerspectiveCamera(fov, aspect, near, far);
this.camera.position.x = position.x;
this.camera.position.y = position.y;
this.camera.position.z = position.z;
}
componentDidMount() {
this._render();
}
_render() {
this.context.renderer.render(this.context.scene, this.camera);
}
render() {
return <div>{this.props.children}</div>;
}
}
PerspectiveCamera.contextTypes = {
scene: PropTypes.object,
renderer: PropTypes.object,
};
We call updateThree
when <PerspectiveCamera>
is first initialized and whenever props or children update. This creates a new camera instance with updated properties.
I'm sure Three.js supports changing field of view, aspect ratio, and other properties of an already instantiated camera, but there was no clear way of doing so. It looks like creating a new camera on every requestAnimationFrame
doesn't cause issues.
Every time React updates our component, we call _render
. It performs the actual Three.js rendering of our scene.
Step 3: Two <Cube>
s
Finally, our <Cube>
components know how to render a 3D cube from props, and add themselves to the scene when mounted. They should remove themselves when unmounted, but I didn't implement that part due to "Eh, it's just an example.”
class Cube extends Component {
componentWillMount() {
this.geometry = new THREE.BoxGeometry(1, 1, 1);
this.material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
this.cube = new THREE.Mesh(this.geometry, this.material);
this.context.scene.add(this.cube);
}
componentDidUpdate() {
const { rotation, position } = this.props;
this.cube.rotation.x = rotation.x;
this.cube.rotation.y = rotation.y;
this.cube.position.x = position.x;
this.cube.position.y = position.y;
this.cube.position.z = position.z;
}
render() {
return null;
}
}
Cube.contextTypes = {
scene: PropTypes.object,
};
Officially, a <Cube>
component renders null
. Unofficially, it adds itself to the scene with scene.add
in componentWillMount
.
When it's being mounted, it also sets up the object itself with Geometry
, adds a green Mesh
, and updates its own rotation
and position
on every componentDidUpdate
. This creates smooth animation if we change props often enough.
Every requestAnimationFrame
is best.
Fin
And that's how we can render declarative 3D scenes in React. This has been a 2-hour experiment. With some more work, it could become a proper way to use Three.js with a React approach.
Surely someone's already built that…
Continue reading about Livecoding recap #41: Towards declarative 3D scenes with React and Three.js
Semantically similar articles hand-picked by GPT-4
- Livecoding Recap: A new more versatile React pattern
- Livecoding Recap #46: 3D is hard, WebAR defeats me
- Livecoding #12: towards animating 10k+ elements with React
- Livecoding #13: rendering React components with canvas
- Livecoding #14: Mostly-smooth animation up to 4,000 elements with React and canvas
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below 👇
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails 💌 on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. 👌"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help 👉 swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers 👉 ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are ❤️