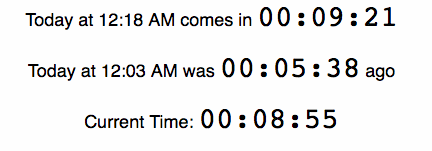
Ever wanted to build a timer in React? It's a tricky thing.
Ideally, React components rely only on their inputs β the props β so that they're testable, reusable, and easier to understand. Time is the ultimate state, that global dependency that just won't sit still.
Calling new Date()
or moment()
to get the current time in your component makes it dirty. You can't test because it throws unpredictable results depending on system context. You can't reuse and re-render either. Damn thing keeps changing!
And you need some mechanism to keep refreshing that time state if you want your component to show something live. You can add a setInterval
that runs this.setState(moment())
every second, and that's going to work. But if you show multiple timers on screen, they're eventually going to diverge and look weird.
Crib from system/hardware design
Those problems have all been solved already in electronics and operating systems: itβs the clock signal in electronics and system time in operating systems. Both reduce time to a stateful resource that everyone shares.
The idea is this:
- make a
Clock
store - emit an action every
period
milliseconds - share
Clock
with everyone
With this approach, time becomes just another prop passed or injected into components that need it. Components themselves can treat it like an immutable value, which makes them easy to test, and because all components rely on the same setInterval
, they're never going to fall out of sync.
Let me show you.
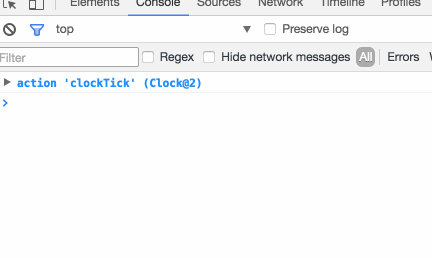
I'm going to use MobX, but the same approach should work with Redux and even the classical state-from-main-component-callbacks-up-components approach.
You can see the code on Github.
Clock store
First, we need a Clock
store. It looks like this:
import { observable, action } from "mobx";
import moment from "moment";
class Clock {
@observable time = moment();
constructor(period = 1000) {
this.interval = setInterval(() => this.clockTick(), period);
}
@action clockTick(newTime = moment()) {
this.time = newTime;
}
}
const clock = new Clock();
export default clock;
We have an @observable
time
property, which means MobX is going to communicate changes to any observer
s. The constructor
starts an interval with the specified period. In our case, this is going to be a 1Hz clock updating once per second.
We store the interval in this.interval
so that we can stop it. This implementation doesn't allow the clock to be stopped or reset, but it would be easy to add an action for that.
Each tick of the clock triggers an @action
called clockTick
, which updates the time
property to the current time. MobX is smart enough to let us naively replace it with a new instance of moment()
, and I'm sure Redux would be too.
The real trick to making this work is in the last two lines:
const clock = new Clock();
export default clock;
We export an instance of Clock, not the class. This ensures that anywhere we use import Clock
, we get the same singleton.
"But inject()! You can inject() and that will make a singleton!β I hear you thinking.
Yes, but you can only inject()
into React components. Sometimes MobX
stores need access to The Clock as well. You can't inject into those, but you can import stuff.
Time display
Clock
was the fun part. Let me show you that it's easy to use :)
We make a Time*
component that displays durations. It can be a functional stateless component that takes Clock
from React contexts via inject()
and renders a thing.
const CurrentTime = inject("Clock")(
observer(({ Clock }) => (
<div>
Current Time: <duration d={Clock.time}></duration>
</div>
))
);
Observes changes on Clock
state and gets Clock
from the context with inject
. You could inject a static object with a time = N
property when testing.
The Duration
component takes a moment()
object and displays it as a digital clock. Like this:
const Duration = ({ d }) => {
let h = d.hours(),
m = d.minutes(),
s = d.seconds();
[h, m, s] = [h, m, s].map((n) => (n < 10 ? `0${n}` : n));
return (
<code>
{h}:{m}:{s}
</code>
);
};
Take hours
/minutes
/seconds
from the object, and prefix with a 0
when each is smaller than 10
. Yes, this breaks for negative values. It looks like this: 0-1:0-4:05
.
But that shouldn't be hard to fix ?
In the full code, I built a generalized Time
displays that defers to TimeSince
, TimeUntil
, and CurrentTime
as necessary. Their code is almost identical, so I didn't want to explain it all here.
Putting it together in App.js
Now that you have a system Clock
and a way to show Time
, you can put it all together inside your App
like this:
class App extends Component {
render() {
return (
<provider clock={Clock}>
<div class="App">
<devtools>
<div class="App-intro">
<time until="{moment().add(10," 'minutes')}="">
<time since="{moment().subtract(5," 'minutes')}="">
<time>
</time></time></time></div>
</devtools></div>
</provider>
);
}
}
Provider
puts the Clock
singleton in React context. This gives all your components access to the Clock
via inject()
and you can do anything you want with it.
Cool, isn't it?
And yes, it works in stores, too.
Check this out from my Day Job code.
MobX lets you do really cool things. when() might just be my fav feature. pic.twitter.com/nGDwmsOJ2c
β Swizec Teller (@Swizec) November 8, 2016
It's in a store, isOutOfTime
is a @computed
property that compares Clock.time
to some pre-defined deadline. when()
the deadline passes, an @action
is called.
@computed get timeLeft() {
if (this.should_end_by) {
return moment.duration(
this.should_end_by
.diff(Clock.time)
);
}else{
return null;
}
}
@computed get isOutOfTime() {
return !_.isNull(this.timeLeft) && this.timeLeft <= 0;
}
Isn't that cool? I think it's cool. The code is easy to understand, easy to test, no messing around with intervals and relying on slippery system properties. Things just workβ’.
It's almost like your codebase is in a time monad ?
Continue reading about Modeling time in React
Semantically similar articles hand-picked by GPT-4
- Yet another hard lesson about time
- How to structure your MobX app for the real world
- Simple MobX-driven modals
- Livecoding #25: Adding MobX to a vanilla React project
- Animating with React, Redux, and d3
Learned something new?
Read more Software Engineering Lessons from Production
I write articles with real insight into the career and skills of a modern software engineer. "Raw and honest from the heart!" as one reader described them. Fueled by lessons learned over 20 years of building production code for side-projects, small businesses, and hyper growth startups. Both successful and not.
Subscribe below π
Software Engineering Lessons from Production
Join Swizec's Newsletter and get insightful emails π on mindsets, tactics, and technical skills for your career. Real lessons from building production software. No bullshit.
"Man, love your simple writing! Yours is the only newsletter I open and only blog that I give a fuck to read & scroll till the end. And wow always take away lessons with me. Inspiring! And very relatable. π"
Have a burning question that you think I can answer? Hit me up on twitter and I'll do my best.
Who am I and who do I help? I'm Swizec Teller and I turn coders into engineers with "Raw and honest from the heart!" writing. No bullshit. Real insights into the career and skills of a modern software engineer.
Want to become a true senior engineer? Take ownership, have autonomy, and be a force multiplier on your team. The Senior Engineer Mindset ebook can help π swizec.com/senior-mindset. These are the shifts in mindset that unlocked my career.
Curious about Serverless and the modern backend? Check out Serverless Handbook, for frontend engineers π ServerlessHandbook.dev
Want to Stop copy pasting D3 examples and create data visualizations of your own? Learn how to build scalable dataviz React components your whole team can understand with React for Data Visualization
Want to get my best emails on JavaScript, React, Serverless, Fullstack Web, or Indie Hacking? Check out swizec.com/collections
Did someone amazing share this letter with you? Wonderful! You can sign up for my weekly letters for software engineers on their path to greatness, here: swizec.com/blog
Want to brush up on your modern JavaScript syntax? Check out my interactive cheatsheet: es6cheatsheet.com
By the way, just in case no one has told you it yet today: I love and appreciate you for who you are β€οΈ